Managing OOP Championships with ECP Class in Java
Develop a Java program using ECP class to manage OOP championships, simulate match results, and query team information. Utilize classes like Team, Championship, and helper methods to handle operations efficiently. Follow best practices to process queries and avoid static variables for logical operations.
Download Presentation
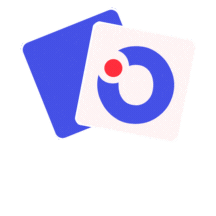
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Sit-In Lab 2 - OOP Championship
Manage championships and perform these types of queries: Simulate one team wins in a championship Simulate a match with the result of draw in a championship Print the name of the highest-ranked team in a given championship Print the name of the team that has played the most number of matches across all championship Print the top three teams names in a given championship Problem Description
ECP Team1 Championship1 Team2 Team3 Championship2 Team4 Problem Illustration
Class ECP ECP - championships : ArrayList<Championship> + //methods Class Team Class Group Table Group - - - - - + getName() : String + setPoints() : void + getPoints() : int name : String points : int numOfWins: int numOfMatchesPlayed: int champName:String - - name : String teamList : ArrayList<Team> + getName() : String + getTeamList() : ArrayList<Team> + addTeam() : void Class Overview
ECP: public class ECP() Team: class Team(String teamName, String champName) Championship: class Championship(String name) Constructors
public Team(String teamName, String champName) { this.name = teamName; this.points =0; this.numOfWins = 0; this.numOfMatchesPlayed = 0; this.champName = champName; } public Championship(String name) { this.name = name; teams = new ArrayList<Team>(); } Constructors
Some tips for processing the queries: Perform all logical operations in the ECP class The methods in the Team and Championship class are only for operations that modify or return their own attributes. Avoid the use of static variables and methods We define helper methods in ECP as we go along, e.g: getChampionship(String championshipName): returns the championship with the name championshipName getTeamBasedOnName(String teamName): returns the team with the name teamName teamName Useful Tips
public class ECP { private ArrayList<Championship> championships = new ArrayList<Championship>(); public static void main(String args[]) { ECp ecp = new ECP(); ecp.run(); } public void run() { Scanner sc = new Scanner(System.in); int numOfChamps = sc.nextInt(); int numOfTeams = sc.nextInt(); int numOfQueries = sc.nextInt(); loadChamps(sc,numOfChamps); loadTeams(sc,numOfTeams); executeQueries(sc,numOfQueries); } }
private void loadChampionships(Scanner sc,int numOfChamps ) { for (int i=0; i<numOfChamps; i++) { championships.add( new Championship(sc.next())); // read input // new an instance of Championship // add the instance to ArrayList championships } } private void loadTeams(Scanner sc,int numOfTeams) { for (int i =0; i<numOfTeams; i++) { // read input String teamName = sc.next(); String championshipName = sc.next(); // new an instance of Team ... // find the championship by name Championship championship = getChampionship(championshipName); // add the team to ArrayList teamList in championship ... } }
private Championship getChampionship(String championshipName) { // iterating through ArrayList championships, // return the championship with name championshipName } private Team getTeamBasedOnName(String teamName) { // iterating through ArrayList championships, get the teamList in it // iterating through teamList // return the team with name teamName } Helper Methods
private void executeQueries(Scanner sc, int numOfQueries) { for (int i=0; i<numOfQueries; i++) { //execute queries based on type switch (sc.next()) { case "win": winMatch(sc.next(), sc.next()); break; case "draw": drawMatch(sc.next(), sc.next()); break; case "top": printTop(sc.next()); break; case "max": printMax(); break; case "final": printFinal(sc.next()); } } }
Query win private void winMatch(String winningTeamName, String losingTeamName) { // find the two teams Team winningTeam = getTeamBasedOnName(winningTeamName); Team losingTeam = getTeamBasedOnName(losingTeamName); // check if they are in the same championship if (!checkIfValidMatching(winningTeam, losingTeam)) { System.out.println("invalid matching"); } else { // update points of winningTeam ... // update numOfWins of winningTeam ... // update numOfMatchesPlayed of winningTeam ... // update numOfMatchesPlayed of losingTeam ... // print the name of championship System.out.println(winningTeam.getChampName()); } } Query 1: Assign group to favorite table
private boolean checkIfValidMatching(Team team1, Team team2) { return team1.getChampName().equals(team2.getChampName()); } Helper Methods
Query draw private void drawMatch(String team1Name, String team2Name) { // find the two teams Team team1 = getTeamBasedOnName(team1Name); Team team2 = getTeamBasedOnName(team2Name); // check if they are in the same championship if (!checkIfValidMatching(team1, team2)){ System.out.println("invalid matching"); } else { //update points of two teams ... //update numOfMatchesPlayed of two teams ... //print the name of championship System.out.println(team1.getChampName()); } } Query 2: Assign group to a table
Query top private void printTop(String championshipName) { // find the championship by given name Championship championship=getChampionship(championshipName); // find the top team Team topTeam=getTopTeam(championship); // print the name of top team System.out.println(topTeam.getName()); }
private Team getTopTeam(Championship championship) { // initial top team Team topTeam = championship.getTeamList().get(0); // iterating through teamList for (Team team:championship.getTeamList()) { if (team.getPoints()>topTeam.getPoints()) { // more number of points topTeam = team; } else if (team.getPoints()==topTeam.getPoints() && team.getNumOfWins()>topTeam.getNumOfWins()) { // more number of wins topTeam = team; } else if (team.getPoints()==topTeam.getPoints() && team.getNumOfWins()>topTeam.getNumOfWins() && team.getName().compareTo(topTeam.getName())<0) { // lexi-smaller topTeam = team; } } return topTeam; }
Query max private void printMax() { Team maxTeam = championships.get(0).getTeamList().get(0); // iterating through ArrayList championships, get the teamList in it // iterating through teamList // compare numOfMatchesPlayed // if two number are the same, choose the lexi-smaller one // print the name of maxTeam }
Query final: the last query private void printFinal(String championshipName){ // find the championship by given name Championship championship = getChampionship(championshipName); if (only two teams) { // find the top team Team topTeam = getTopTeam(championship); // print topteam name,space ... // remove topTeam from teamList ... // print top team name } else { // loop for three times // find the top team, print name, space(no space for the third one) // remove the top team } }