Understanding Static Variables and Methods in Java
Static variables in Java belong to the class rather than instances of the class and are initialized only once at the start of execution. They can be accessed directly by the class name. Similarly, static methods can access static variables directly without creating an object. This content explains the concept of static variables, methods, and their initialization in Java with examples.
Download Presentation
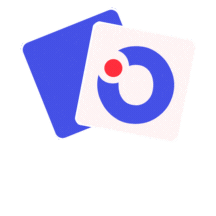
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Amity School of Engineering & Technology Static Variable, Method and Block 1
Amity School of Engineering & Technology Static Variable Static variable which belong to the class and initialize only once at the start of the execution. It is belong to the class ,not to the object(instance). A single copy of to be shared by all the object of the class. A static variable can be accessed directly by the class name and not need any object.
Amity School of Engineering & Technology Example class VarDemo { static int count=0; public void increment() { count++; }
Amity School of Engineering & Technology Example public static void main(String args[]) { VarDemo obj1=new VarDemo(); VarDemo obj2=new VarDemo(); obj1.increment(); obj2.increment(); System.out.println("Obj1: count is="+obj1.count); System.out.println("Obj2: count is="+obj2.count); } }
Amity School of Engineering & Technology Static Variable can be accessed directly in a static method class JavaExample{ static int age; static String name; //This is a Static Method static void disp(){ System.out.println("Age is: "+age); System.out.println("Name is: "+name); }
Amity School of Engineering & Technology Example public static void main(String args[]) { age = 30; name = "Steve"; disp(); } }
Amity School of Engineering & Technology Static variable initialization Static variables are initialized when class is loaded. Static variables are initialized before any object of that class is created. Static variables are initialized before any static method of the class executes.
Amity School of Engineering & Technology Default values for static and non- static variables are same. primitive integers(long, short etc): 0 primitive floating points(float, double): 0.0 boolean: false object references: null const int pi;
Amity School of Engineering & Technology Static final variables public class MyClass{ public static final int MY_VAR=27; } Final variable always needs initialization, if you don t initialize it would throw a compilation error. have a look at below example- public class MyClass{ public static final int MY_VAR; }
Amity School of Engineering & Technology Cont.. The variable MY_VAR is public which means any class can use it. It is a static variable so you won t need any object of class in order to access it. It s final so the value of this variable can never be changed in the current or in any class.
Amity School of Engineering & Technology Static Method Static methods are the methods in Java that can be called without creating an object of class. They are referenced by the class name itself or reference to the Object of that class. Static method can only access static data. Static method can not access non static data.
Memory Allocation: Amity School of Engineering & Technology They are stored in the Permanent Generation space of heap as they are associated with the class in which they reside not to the objects of that class. But their local variables and the passed argument(s) to them are stored in the stack. Since they belong to the class, so they can be called to without creating the object of the class.
Amity School of Engineering & Technology Important Points Static method(s) are associated with the class in which they reside i.e. they are called without creating an instance of the class i.e ClassName.methodName(args) They are designed with the aim to be shared among all objects created from the same class. Static methods can not be overridden, since they are resolved using static binding by the compiler at compile time. However, we can have the same name methods declared both superclass and subclass, but it will be called Method Hiding as the derived class method will hide the base class method. in static
Amity School of Engineering & Technology Example class Geek { public static String geekName = ""; public static void geek(String name) { geekName = name; } }
Amity School of Engineering & Technology Example class GFG { public static void main(String[] args) { // Accessing the static method geek() // and field by class name itself. Geek.geek("vaibhav"); System.out.println(Geek.geekName); // Accessing the static method geek() // by using Object's reference. Geek obj = new Geek(); obj.geek("mohit"); System.out.println(obj.geekName); } }
Amity School of Engineering & Technology When to use static methods? When you have code that can be shared across all instances of the same class, put that portion of code into static method. They are basically used to access static field(s) of the class.
Amity School of Engineering & Technology Instance method vs Static method Instance method can access the instance methods and instance variables directly. Instance method can access static variables and static methods directly. Static methods can access the static variables and static methods directly. Static methods can t access instance methods and instance variables directly. They must use reference to object. And static method can t use this keyword as there is no instance for this to refer to.
Amity School of Engineering & Technology Static Block In a Java class, a static block is a set of instructions that is run only once when a class is loaded into memory. A static block is also called a static initialization block. This is because it is an option for initializing or setting up the class at run- time. Static block always executed before main function
Amity School of Engineering & Technology Static Block class Test { staticint i; // Static Variable int j; // Non-Static Variable static //Static Block { i = 10; System.out.println("static block called "); } } 19
Amity School of Engineering & Technology class Test { // Main driver method publicstaticvoid main(String args[]) { // Although we don't have an object of Test, static // block is called because i is being accessed in // following statement. System.out.println(Test.i); } } 20