Differences Between C# and Java: A Comparative Overview
C# and Java share similarities in syntax and features, but there are key distinctions that set them apart. From class inheritance to memory management, this comparison highlights the unique aspects of C# programming. Explore how C# diverges from Java in terms of multiple inheritance, explicit heap allocation, array handling, control statements, and more. Understand the nuances that make C# a distinct language while identifying commonalities with Java.
Download Presentation
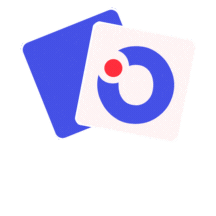
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
C# In many ways, C# looks similar to Java there are some areas of C# that look like C++ for instance, extending a class is handled by : as with C++ Rather than covering C# from scratch, we concentrate on the differences Like Java, C# programs are implemented in classes with one being static and having a main method C# classes inherit from only one class (no multiple inheritance) but abstract classes can be used similar to Java to inherit additional items heap items are explicitly allocated using new and are garbage collected all objects are heap objects, referenced by reference variables
Similarities with Java Must have a class with main, variable declarations are the same Arrays are mostly treated the same C# has indexers which can allow non-array list types to be indexed as if arrays C# also permits true multidimensional arrays as well as arrays of arrays Both languages do full type-checking at compile time at run-time, both languages test a polymorphic variable s type to ensure it has been cast correctly and if not, throw exceptions according to one researcher, Java is less safe Same set of control statements the switch requires that break be inserted in every case, even a default case the switch statement can be used on string variables C# has an explicit iterator loop (foreach) while the for loop was modified in later versions of Java to act as an iterator
Continued Both languages have classes and primitives but in C#, all primitives are objects however, primitives can be stored on the run-time stack instead of the heap, and access to primitives is without message passing so neither language is a pure OOPL Same use of public, private, protected, this, static, new the package level of visibility is called internal in C#, and is used for classes that are in the same assembly (discussed in a few slides) C# also has protected internal and private protected C# uses the reserved word override as opposed to Java s @Override C# uses sealed instead of final for classes that cannot be extended and methods that cannot be overridden
Continued Exception handling are similar (try/catch/finally blocks, built-in exceptions) C# has no equivalent of a non RuntimeException they have different Exception class hierarchies Both languages use classes for handling all I/O C# has an ArrayList but it cannot be used with Generics Polymorphism is the same including the need to cast when assigning a polymorphic variable to a subclass object Both languages are interoperable in that code is compiled to an intermediate level in C#, intermediate code of different languages can call each other (e.g., an F# object can communicate with a C# object) C# however can be compiled directly into executable code
Differences to Java Rather than using super to invoke parent class constructor, you can use public ClassName(params) : base(params) here, the second set of params are sent to the parent class constructor You can define your own destructor for a class (not necessary but could potential be useful) if you have one, you cannot invoke it directly, instead it is invoked by the garbage collector when collection a no-longer-referenced object the garbage collector invokes the Finalize method of the Object class which itself invokes your class destructor C# allows namespaces (Java supports this via packages but there are subtle differences0 C# has an enum type
Continued C# has more primitives including unsigned values Java only has signed numeric values C# objects can be created either from a class and stored in the heap from a struct and stored in either the heap or the run-time stack structs are not like C/C++ structs because they are encapsulated with methods and use visibility modifiers (although do not support inheritance/polymorphism) in this way, structs are used to create non-object-oriented ADTs C# has explicit pointers these pointers can be used to pass primitives by reference rather than copy (covered later in these notes) C# pointers can be used only in Unsafe code (Unsafe is a reserved word)
Continued The reserved word new is not only used to create a new object but used to hide a previously defined method when inheriting or implementing from a virtual class C# includes some reserved words from C++ not found in Java like sizeof, virtual, typeof C# does not have checked exceptions that Java has C# files can contain multiple public classes in Java, this is only possible if the classes are nested inside another class C# permits anonymous (nameless or lambda) methods C# is a more complex and larger language both because of the added features described here and the number of built-in classes supporting C#
Other C# Concepts Constants are declared using const or readonly const constants are static and must be initialized in their declaration readonly can be initialized either in their declaration or in the constructor (and if you have different constructors, the variable can be set to different values by each constructor that is, the constant only stores one value, but that value can be controlled at run-time by the selection of the constructor) Assemblies this is a feature of .Net more so than C# files containing classes are compiled into an intermediate code called an assembly (like Java s byte code) assemblies contain metadata (features of the classes) and a file called the Manifest (information about the current version of the assembly) the main difference between this and byte code is that the assemblies can be from differing languages namespaces are available in C# in case there are name collisions between assemblies being used by another class
Continued Class properties provides a way to access private instance data in public ways without explicitly having methods to do so we cover this in the next couple of slides Delegates classes that encapsulate one or more references to methods recall in C, LISP and other languages, you can pass functions (or pointers to functions) to functions delegates are differentiated between singlecast delegates delegate contains a single method, its parent is Delegate multicast delegates delegate contains multiple methods, its parent is MulticastDelegate Operator overloading available for classes defined using: public returntype operator operation(params) {body} for instance: public String operator * (String x, String y) { }
Properties A property of a class is a means of accessing private instance data while hiding the actual accessor/mutator methods details for instance, the code used in a mutator to verify proper data A property is a name defined to stand for an instance datum for instance, we might define an instance datum name and the property Name The property can be accessed via code written using get define an accessor set define a mutator value keyword indicating the value being tested in a set => syntactic notation used to define a value to set to a property Properties can be given visibility modifiers to control which code can use them for instance, public versus protected or internal Access the datum via the property, not the instance datum
Example public class Person private string first, last; From main: public Person(string f, string l) { first = f; last = l; } public string First { get { return first; } set { if(value!= ) first = value; else first = unknown ; } } var p = new Person( Frank , Zappa ); Console.WriteLine(p.Name); public string Last { get { return last; } set { if(String.IsNullOrEmpty(value)) last = value; else last = unknown ; } } public string Name => $ {first} {last} ; }
Parameter passing C# by default uses pass by copy just like Java C# also has a pass by reference mode place ref or out in both the method call and method header foo(ref x, y); public void foo(ref int x, int y) { } the difference between ref and out is that out is a true out mode while ref requires that the parameter be initialized before you can pass it as a reference (that is, ref is an in-out mode) There are two ways to pass optional parameters using params allows you to pass any number of values for a single argument the parameter must be some type of array (e.g., array of Strings like in Java or an array of objects) another form of optional parameter requires that you specify a default value in case the parameter is not passed as in foo(int x, string optional = default string ) C# also permits keyword parameters instead of positional parameters
Implementing Delegates Remember that the delegate is not the method that will be passed to another method but is a reference variable that can point to (hold) a method this reference can change what it holds at runtime so that different methods can be passed to a method via the delegate First define the delegate itself which includes a prototype of the type of method that can be referenced public delegate returntype Name(params) public delegate String MyDelegate(String s); Now define methods that fit this prototype public delegate String uppercase(s); public delegate String lowercase(s); Assign our delegate to one of the methods and then invoke the method through the delegate MyDelegate foo = new uppercase(s); (or new lowercase(s);) someString = foo(someString);
Example Imagine that we have several String methods as follows remove non-alphabetic characters (removeNonAlpha) remove non-alphanumeric characters (removeNonAlphaNumeric) remove HTML tags (removeHTML) We are not sure at compile time which method we need to call upon to parse some text file that has been entered into a String so we implement the following public delegate string ChangeString(string x); static void main(string[] args) { ChangeString myDelegate; switch (choice) { case non-alphabetic : myDelegate = removeNonAlpha; break; case non-alphanumeric : myDelegate = removeAlphaNumeric; break; case html : myDelegate = removeHTML; break; default: myDelegate = ; break; } string x = myDelegate(getTextFile());
Returning a Delegate In the previous example, our method invoked a method via the delegate based on some condition We can also return the method via a delegate as follows public static ChangeString(string conditional) { ChangeString temp; if(conditional.equals( non-alphabetic ) temp = removeNonAlpha; else if(conditional.equals( non-alphanumeric ) temp = removeAlphaNumeric; else if(conditional.equals( html ) temp = removeHTML; else temp = ; return temp; } We can then call this using string s = ChangeString(condition).Invoke(getTextFile());
Why Use Delegates In these examples, why bother with the delegate? Just invoke the method in the if-else/switch statement in most cases, using method calls is simpler as you don t have to define the delegate itself But there are some situations where the delegate approach is better imagine having hundreds of methods and the one invoked is based on an int value you can create an array of delegates and invoke the method using the int value as an array index, thus saving having to write a lengthy nested if-else or switch statement the delegate serves as a method placeholder you can add more methods later and have less code to recompile you would have to compile the new methods and the code that assigns the delegate to the method, but not the class that uses the delegate delegates are used to implement event handling in C#