Understanding Object-Oriented Programming (OOP) in Python
Object-Oriented Programming (OOP) is a programming paradigm that focuses on organizing code into objects with attributes and behaviors. Python supports various OOP concepts such as classes, objects, inheritance, polymorphism, abstraction, and encapsulation. Classes serve as blueprints for creating objects, encapsulating data and functions. Objects are instances of classes with attributes and methods. In Python, class inheritance allows for creating subclasses that inherit and modify attributes and methods from their superclasses, promoting code reuse and modularity.
Download Presentation
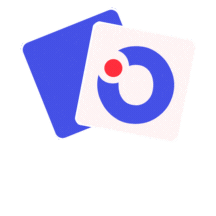
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Object Oriented Programming (OOPs) CSE 1300 Module 7
Content What is Object-oriented Programming? Classes and Objects in Python. Inheritance Polymorphism Abstraction Encapsulation.
Object-oriented programming Object-oriented programming (OOP) is a programming paradigm that focuses on organizing code into objects that have attributes and behaviors. Python supports OOP concepts such as: Classes Objects Inheritance Polymorphism Abstraction Encapsulation.
Classes and Objects in Python: Class: A class is a blueprint or template for creating objects that defines a set of attributes and methods. It encapsulates data and functions that work together to represent an entity in the program. A class is defined using the "class" keyword, followed by the name of the class. Classes are used to organize code and create reusable code modules. Objects: An object is an instance of a class. Objects have attributes, which are data elements that store values, and methods, which are functions that operate on those values.
Class and objects in Python Objects in Python are a fundamental part of object-oriented programming and allow for the creation of complex, reusable code structures. To create an object in Python, you first need to define a class. Once the class is defined, you can create objects from it. Example: class Person: def __init__(self, name, age): self.name = name self.age = age person1 = Person("Alice", 25)
Explanation: In the example, we define a class called Person with two attributes: name and age . We also define a constructor method called __init__ that sets the values of these attributes when a new object is created. To create an object of the Person class, we call the constructor method and pass in values for the name and age attributes. We assign this object to a variable called person1. We can access the attributes of the person1 object using dot notation: print(person1.name) # Output: Alice print(person1.age) # Output: 25 Overall, objects in Python are a fundamental part of object-oriented programming and allow for the creation of complex, reusable code structures.
Inheritance Class inheritance is a mechanism in object-oriented programming that allows a new class to be based on an existing class, inheriting attributes and methods from the superclass while also adding new functionality to create a modified version of the original class. The subclass inherits attributes and methods from the superclass, and can also add new attributes and methods, or override existing ones. This allows for code reuse and promotes modularity and extensibility.
Example: class Animal: def __init__(self, name, species): self.name = name self.species = species def eat(self): print(f"{self.name} is eating.") class Dog(Animal): def bark(self): print("Woof!") class Cat(Animal): def meow(self): print("Meow!") Here, Animal is the superclass, and Dog and Cat are subclasses. They inherit the name and species instance variables and eat() instance method from the Animal class.
Single Inheritance: Single level inheritance allows a subclass to inherit traits from only one parent class. Inheritance is a mechanism that allows a new class to be based on an existing class, inheriting its properties and methods. Single inheritance means that a new class is derived from a single base class. In Python, we can create a new class by inheriting from an existing class using the syntax class ChildClass(ParentClass): . The child class (also known as subclass) inherits all the attributes and methods of the parent class (also known as superclass). The child class can also add new attributes and methods or override the ones inherited from the parent class.
Example: Suppose we have a parent class called "Animal" with a method called "speak" that prints a message. We want to create a subclass called "Cat" that inherits from the "Animal" class and also has its own method called "meow" that prints a different message. Here's how we can do it in Python: # Define the parent class class Animal: def speak(self): print("Hello!") # Define the subclass class Cat(Animal): def meow(self): print("Meow!")
Explanation: In this example, the "Cat" class inherits the "speak" method from the "Animal" class and also defines its own method "meow". We can now create objects of the "Cat" class and call its methods like below: # Create an object of the Cat class my_cat = Cat() # Call the inherited method my_cat.speak() # Call the subclass method my_cat.meow() Output: Hello! Meow! As you can see, the "Cat" object can call both the inherited "speak" method and the subclass-specific "meow" method.
Multilevel Inheritance In multilevel inheritance, a class inherits from another derived class. This creates a hierarchy of classes, with each new class inheriting properties and methods from the previous classes. Multilevel inheritance in Python allows us to create a hierarchy of classes that inherit properties and methods from the previous classes. This promotes code reuse and makes our code more organized and readable.
Example: class Animal: def __init__(self, name): self.name = name def sound(self): pass class Dog(Animal): def sound(self): return "Woof! class GermanShepherd(Dog): def sound(self): return "Woof! Woof! my_dog = GermanShepherd("Max") print(my_dog.name) # Output: Max print(my_dog.sound()) # Output: Woof! Woof!
Explanation: We have a base class Animal that defines the __init__ method and a sound method that is empty. The Dog class inherits from the Animal class and overrides the sound method to return "Woof!". The GermanShepherd class inherits from the Dog class and overrides the sound method to return "Woof! Woof!". We create an instance of GermanShepherd and call the name attribute and the sound method to get the output.
Hierarchical Inheritance Hierarchical inheritance is a type of inheritance in which a single base class is inherited by multiple derived classes. In this type of inheritance, the derived classes inherit all the properties and behaviors of the base class, but they can also have their own unique properties and behaviors. Here,we can implement hierarchical inheritance by defining a base class and then creating derived classes that inherit from the base class.
Example class Animal: def __init__(self, name): self.name = name def speak(self): print(f"{self.name} is speaking.") class Dog(Animal): def __init__(self, name): super().__init__(name) def bark(self): print(f"{self.name} is barking.") class Cat(Animal): def __init__(self, name): super().__init__(name) def meow(self): print(f"{self.name} is meowing.")
Explanation In this example, we have a base class called Animal, which has a constructor method that initializes the name attribute and a speak method that prints the name of the animal and that it's speaking. We then have two derived classes: Dog and Cat. Both of these classes inherit from the Animal class and have their own constructor methods that call the super() function to call the constructor of the base class. The Dog class has a bark method that prints the name of the dog and that it's barking, while the Cat class has a meow method that prints the name of the cat and that it's meowing. This is an example of hierarchical inheritance because both the Dog and Cat classes inherit from the same base class, Animal.
Polymorphism Polymorphism is the ability of objects of different classes to be used interchangeably, even if they have different implementations. In other words, polymorphism allows us to write code that can work with different types of objects, without having to know their specific class or implementation details. It is one such OOP methodology where one task can be performed in several different ways. To put it in simple words, it is a property of an object which allows it to take multiple forms In Python, polymorphism is achieved through two types: Compile-Time Polymorphism and Run-Time Polymorphism
Compile-Time Polymorphism Compile-Time Polymorphism also known as Method Overloading. It allows a class to have multiple methods with the same name but different parameters. The decision of which method to call is made during the compilation of the code. Example: class Calculator: def add(self, a, b): return a + b def add(self, a, b, c): return a + b + c calc = Calculator() print(calc.add(2, 3)) # Output: TypeError: add() missing 1 required positional argument: 'c' print(calc.add(2, 3, 4)) # Output: 9
Run-Time Polymorphism Run-Time Polymorphism is also known as Method Overriding. It allows a subclass to have a method with the same name as a superclass method. The decision of which method to call is made during the execution of the code. The decision of which method to call is made during the compilation for Compile-Time Polymorphism and during the execution for Run-Time Polymorphism.
Example class Animal: def make_sound(self): print("Animal is making a sound.") class Dog(Animal): def make_sound(self): print("Dog is barking.") class Cat(Animal): def make_sound(self): print("Cat is meowing.") animals = [Dog(), Cat()] for animal in animals: animal.make_sound() Output Dog is barking. Cat is meowing.
Abstraction Abstraction is a programming concept that allows developers to focus on essential features of a problem domain while hiding unnecessary details. In Python, abstraction can be achieved through the use of classes, functions, and modules. Abstraction is an essential concept in Python programming that allows us to create more manageable and reusable code. By using abstraction,we can focus on the essential features of the problem domain and reduce complexity.
Example: def add_numbers(num1, num2): return num1 + num2 def multiply_numbers(num1, num2): return num1 * num2 result = add_numbers(2, 3) print(result) result = multiply_numbers(2, 3) print(result) Here, we've created two functions, add_numbers and multiply_numbers, that perform specific mathematical operations. We can then call these functions whenever we need to perform those operations, without having to rewrite the same code over and over again.
Encapsulation Encapsulation is one of the fundamental concepts of object-oriented programming (OOP) It refers to the idea of bundling data and methods that work on that data within one unit, i.e., a class In Python, encapsulation is achieved through the use of access modifiers, namely public, private, and protected These access modifiers control the visibility of class attributes and methods to the outside world Encapsulation is a powerful concept in OOP that allows us to protect data and methods within a class In Python, we can achieve encapsulation through access modifiers and defining getter and setter methods
Access Modifiers Public: Denoted by no underscore, accessible to everyone Private: Denoted by double underscore (i.e., __), accessible only within the class Protected: Denoted by single underscore (i.e., _), accessible only within the class and its subclasses Let's say we have a class called Employee, which has attributes like name, age, and salary. We want to ensure that these attributes are only accessible and modifiable through methods, not directly.
Example class Employee: def init(self, name, age, salary): self.__name = name self.__age = age self.__salary = salary def get_name(self): return self.__name def set_name(self, name): self.__name = name def get_age(self): return self.__age def set_age(self, age): self.__age = age def get_salary(self): return self.__salary def set_salary(self, salary): self.__salary = salary
Example In this example, we use double underscores to make the attributes private, and we define getter and setter methods to access and modify them. This way, we can ensure that the attributes are only accessed and modified in a controlled way.
Advantages of OOPS: OOP provides several benefits that can help you write more efficient, effective, and maintainable code. By using OOP, you can create more reusable, flexible, and adaptable code that is easier to manage and maintain over time. Reusability in OOP promotes reuse of code through inheritance, where a subclass can inherit the properties and behavior of its parent class. This reduces the amount of code that needs to be written and makes it easier to maintain and modify the code. Modularity in OOPs allows developers to break down a large program into smaller, more manageable modules. Each module can be developed and tested independently, making it easier to maintain and modify the code. OOP provides encapsulation, which means that data and methods that work on that data are bundled together within a class. This allows developers to hide the implementation details of a class from the outside world, which promotes better code organization and reduces the risk of unintended side effects.
Advantages of OOPS: OOP supports polymorphism, which means that objects of different types can be treated as if they were of the same type. This allows developers to write code that is more generic and flexible, making it easier to modify and extend the code over time. OOP makes it easier to add new functionality to existing code without breaking the existing code. Developers can do this by creating new classes that inherit from existing classes and adding or overriding methods as needed. Maintainability: OOP promotes better code organization and reduces the risk of unintended side effects, making it easier to maintain and modify code over time. This is especially important for large, complex projects that require ongoing maintenance.