Understanding Abstract Classes in C++ and Java
Abstract classes play a crucial role in C++ and Java programming. In C++, a class with at least one pure virtual function becomes abstract, while in Java, the 'abstract' keyword is used to define an abstract class. This article discusses the concept of abstract classes, their key characteristics, examples, and the importance of abstract classes in object-oriented programming.
Download Presentation
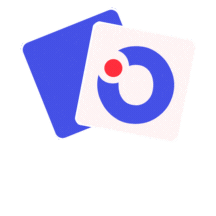
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Amity School of Engineering & Technology ABSTRAACT CLASS
Amity School of Engineering & Technology What is Abstract Class In C++, if a class has at least one pure virtual function, then the class becomes abstract. Unlike C++, in Java, separate keyword abstract is used to make a class abstract. abstract class Shape { int color; // An abstract function abstract void draw(); } 2
Amity School of Engineering & Technology Important Point About Abstract Class An instance of an abstract class can not be created. Constructors are allowed. We can have an abstract class without any abstract method. Abstract classes can not have final methods because when you make a method final you can not override it but the abstract methods are meant for overriding. We are not allowed to create object for any abstract class. We can define static methods in an abstract class 3
Amity School of Engineering & Technology Example abstractclass Base { abstractvoid fun(); } // Class 2 class Derived extends Base { void fun() { System.out.println("Derived fun() called"); } } 4
Amity School of Engineering & Technology Cont.. // Class 3 // Main class class Main { // Main driver method publicstaticvoid main(String args[]) { // We can have references of Base type. Base b = new Derived(); b.fun(); } } 5
Amity School of Engineering & Technology Example abstractclass Base { // Constructor of class 1 Base() { // Print statement System.out.println("Base Constructor Called"); } // Abstract method inside class1 abstractvoid fun(); } 6
Amity School of Engineering & Technology Cont.. class Derived extends Base { // Constructor of class2 Derived() { System.out.println("Derived Constructor Called"); } // Method of class2 void fun() { System.out.println("Derived fun() called"); } } 7
Amity School of Engineering & Technology Cont.. class Main { // Main driver method publicstaticvoid main(String args[]) { // Creating object of class 2 // inside main() method Derived d = new Derived(); } } 8
Amity School of Engineering & Technology Example 3 abstractclass Base { // Demo method void fun() { // Print message if class 1 function is called System.out.println( "Function of Base class is called"); } } 9
Amity School of Engineering & Technology Cont.. Class Derived extend Base {} //class 2 class Main { // Main driver method publicstaticvoid main(String args[]) { // Creating object of class 2 Derived d = new Derived(); // Calling function defined in class 1 inside main() // with object of class 2 inside main() method d.fun(); } } 10
Amity School of Engineering & Technology Abstract Classes Can Also Have final Methods abstractclass Base { finalvoid fun() { System.out.println("Base fun() called"); } } // Class 2 class Derived extends Base { } 11
Amity School of Engineering & Technology Cont.. // Class 3 // Main class class Main { // Main driver method publicstaticvoid main(String args[]) { // Creating object of abstract class Base b = new Derived(); // Calling method on object created above // inside main() b.fun(); } } 12
Amity School of Engineering & Technology We can define static methods in an abstract class that can be called independently without an object. abstractclass Helper { // Abstract method staticvoid demofun() { // Print statement System.out.println("Geeks for Geeks"); } } 13
Amity School of Engineering & Technology Cont.. // Main class extending Helper class publicclass GFG extends Helper { // Main driver method publicstaticvoid main(String[] args) { // Calling method inside main() // as defined in above class Helper.demofun(); } } 14
Amity School of Engineering & Technology Questions What is Abstraction in Java? Abstraction in Java is a technique by which we can hide the data that is not required to users. It hides all unwanted data so that users can work only with the required data 15
Amity School of Engineering & Technology 2 How to achieve or implement Abstraction in Java? There are two ways to implement abstraction in java. They are as follows: a) Abstract class (0 to 100%) b) Interface (100%) 16
Amity School of Engineering & Technology 3 What is Abstract class in Java? How to define it? An abstract class in java is a class that is declared with an abstract keyword. Example of Abstract class: abstract class test { abstract void display(); } 17
Amity School of Engineering & Technology 4 What is the difference between abstract class and concrete class? We cannot create an object of abstract class. Only objects of its non-abstract (or concrete) sub classes can be created. It can have zero or more abstract methods that are not allowed in a non-abstract class (concrete class). 18
Amity School of Engineering & Technology 5 What is Abstract in Java? Abstract is a non-access modifier in java that is applicable for classes, interfaces, methods, and inner classes. 19
Amity School of Engineering & Technology 6 What is Abstract method in Java? A method which is declared with abstract modifier and has no implementation (means no body) is called abstract method in java. It does not contain any body. It has simply a signature declaration followed by a semicolon 20
Amity School of Engineering & Technology 7 Can an abstract method be declared as static? No 21
Amity School of Engineering & Technology 8 Can an abstract method be declared with private modifier? No, it cannot be private because the abstract method must be implemented in the child class. If we declare it as private, we cannot implement it from outside the class. 22
Amity School of Engineering & Technology 9 What is Concrete method in Java? A concrete method in Java is a method which has always the body. It is also called a complete method in java. 23
Amity School of Engineering & Technology 10. When to use Abstract class in Java? An abstract class can be used when we need to share the same method to all non-abstract sub classes with their own specific implementations 24
Amity School of Engineering & Technology 11 Is it possible to create an object of abstract class in Java? No. It is not possible but we can create an object of its subclass. 25
Amity School of Engineering & Technology 12 Is it possible that an abstract class can have without any abstract method? Yes 26
Amity School of Engineering & Technology 13,14,15 Can an abstract class have constructor? Yes. 17. Is abstract class allow to define private, final, static, and concrete methods? Yes. 18. Is it possible to achieve multiple inheritance through abstract class? No. 27
Amity School of Engineering & Technology 16 Can we define an abstract method inside non-abstract class (concrete class)? No, we cannot define an abstract method in non-abstract class. 28
Amity School of Engineering & Technology 17 What will happen if we do not override all abstract methods in subclass? Java compiler will generate compile time error. We will have to override all abstract methods in subclass. 29
Amity School of Engineering & Technology 18 What is the difference between Abstraction and Encapsulation? Abstraction hides the implementation details from users whereas, encapsulation wraps (binds) data and code into a single unit 30
Amity School of Engineering & Technology 19 Why abstract class has constructor even though you cannot create object? We cannot create an object of abstract class but we can create an object of subclass of abstract class. When we create an object of subclass of an abstract class, it calls the constructor of subclass. This subclass constructor has a super keyword in the first line that calls constructor of an abstract class. Thus, the constructors of an abstract class are used from constructor of its subclass. If the abstract class doesn t have constructor, a class that extends that abstract class will not get compiled. 31
Amity School of Engineering & Technology 20 Why should we create reference to superclass (abstract class reference)? We should create a reference of the superclass to access subclass features because superclass reference allows only to access those features of subclass which have already declared in superclass. If you create an individual method in subclass, the superclass reference cannot access that method. Thus, any programmer cannot add their own additional features in subclasses other than whatever is given in superclass. 32
Amity School of Engineering & Technology 21 What is the advantage of Abstract class in Java? Abstract class makes programming better and more flexible by giving the scope of implementing abstract methods. Programmer can implement abstract method to perform different tasks depending on the need. We can easily manage code. 33