Python GUI Development: Creating User-Friendly Interfaces
Python can be effectively used for creating Graphical User Interfaces (GUIs) by leveraging libraries like Tkinter and wxPython. This guide covers GUI design principles, event-based programming, and tips for enhancing the user experience. It emphasizes the importance of designing intuitive interfaces, providing helpful functionalities, and conducting usability testing in GUI development.
Uploaded on Sep 23, 2024 | 0 Views
Download Presentation
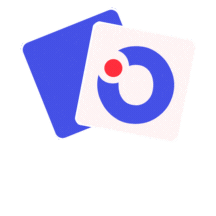
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Graphical User Interfaces (GUIs) In general, Python isn't much used for user interfaces, but there's no reason for not doing so. Build up WIMP (Windows; Icons; Menus; Pointers) Graphical User Interfaces (GUIs). The default package to use is TkInter. This is an interface to the basic POSIX language Tcl ("Tickle", the Tool Command Language) and its GUI library Tk. Also more native-looking packages like wxPython: https://www.wxpython.org/
Basic GUI import tkinter root = tkinter.Tk() # Main window. c = tkinter.Canvas(root, width=200, height=200) c.pack() c.create_rectangle(0, 0, 200, 200, fill="blue") # Layout tkinter.mainloop() # Wait for interactions.
Event Based Programming Asynchronous programming, where you wait for user interactions. In Python, based on callbacks: where you pass a function into another, with the expectation that at some point the function will be run. You register or bind a function with/to an object on the GUI. When an event occurs, the object calls the function.
import tkinter Simple event def run(): pass root = tkinter.Tk() menu_bar = tkinter.Menu(root) root.config(menu=menu_bar) model_menu = tkinter.Menu(menu_bar) menu_bar.add_cascade(label="Model", menu=model_menu) model_menu.add_command(label="Run model", command=run) tkinter.mainloop()
The user experience Many people design for geeks. Users learn by trying stuff - they rarely read manuals, so think carefully about what the default behavior of any function should be. We need to design for the general public, but make advanced functions available for those that want them. We should try to help the user by... Using familiar keys and menus (e.g. Ctrl + C for copy). Including help systems and tutorials.
Designing for users At every stage when designing the GUI, think "is it obvious what this does?" Make all screens as simple as possible. Turn off functionality until needed, e.g.: model_menu.entryconfig("Run model", state="disabled") # Until the user has chosen files, then: model_menu.entryconfig("Run model", state="normal") Hide complex functionality and the options to change defaults in Options menus. Most of all consult and test. There is a formal element of software development called 'usability testing' in which companies watch people trying to achieve tasks with their software.