Understanding Interfaces and Abstract Classes in Java
Interfaces and abstract classes play a crucial role in Java programming by defining contracts and blueprints for classes to implement. Interfaces provide a way for classes to declare their capabilities, while abstract classes allow for partial implementation. This article explains the concepts of interfaces and abstract classes in Java through examples, illustrating how they are used for implementing different functionalities in classes.
Download Presentation
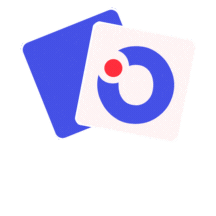
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Interfaces An interface is a contract between its producer and client The general format of an interface definition: public interface InterfaceName { //(Method headers...) } All methods specified by an interface are public by default. A class (as a producer) can implement one or more interfaces. 9-2
Interface in UML and Java <<Interface>> IUSBMemory save() Public interface IUSBMemory { void save(); }
Producer of an Interface If a class implements an interface, it uses the implements keyword in the class header. public class USBMemory implements IUSBMemory { // more fields are declared void save() { }; // more methods are implemented } <<Interface>> IUSBMemory save() USBMemory save() 9-4
Client of an Interface Class Computer uses any class implementing IUSBMemory to save a file public class Computer { private IUSBMemory usb; // more fields are here. void setUsb(IUSBMemory m) { usb = m; } public void editAfile() { usb.save(); } } <<Interface>> IUSBMemory save() Computer usb 1 editAfile() USBMemory save()
Now run your program Remember a class is only a blue-print. To run it, you need to buy computer and usb memory objects. public class Test { public static void main(String[] args) { Computer myComputer = new Computer(); IUSBMemory myusb1 = new USBMemory(); myComputer.setUsb(myusb1); myComputer.editAfile(); } }
New Product Comes A new product implementing IUSBMemory called phone is available. public class Computer { private IUSBMemory usb; // more fields are here. void setUsb(IUSBMemory m) { usb = m; } public void editAfile() { usb.save(); } } <<Interface>> IUSBMemory save() Computer usb 1 editAfile() Phone save() Your Computer Class still works. No need to change!!!
Dont forget to buy a phone!!! Remember a class is a blue-print. Don t forget to buy a phone and connect to your computer. public class Test { public static void main(String[] args) { Computer myComputer = new Computer(); IUSBMemory myusb1 = new USBMemory(); myComputer.setUsb(myusb1); myComputer.editAfile(); Phone phone = new Phone(); myComputer.setUsb(phone); myComputer.editAfile(); } } public class Computer { private IUSBMemory usb; .. void setUsb(IUSBMemory m) { usb = m; } .. }
Fields in Interfaces An interface can contain field declarations: all fields in an interface are treated as final and static. Because they automatically become final, you must provide an initialization value. public interface Doable { int FIELD1 = 1, FIELD2 = 2; (Method headers...) } In this interface, FIELD1 and FIELD2 are final static int variables. Any class that implements this interface has access to these variables. 9-9
Problem of Interface IUSBMemory has many features other than save a file, such as set brand, get brand, set speed and get speed etc. When a producer implements IUSBMemory, it should provide its implementations. But if many producers implement IUSBMemory but share same implementation for some of methods such as set brand and get brand etc. Only difference is how to save, i.e. the implementation of save()?
Abstract Class We declare AbsUSBMemory as an abstract class public abstract class AbsUSBMemory { private String brand; public void setBrand(String b) {brand = b;} public String getBrand() { return brand;} // more fields are here public abstract void save(); } <<abstract>> AbsUSBMemory -brand:String void setBrand(String b) String getBrand() <<abstract>> void save()
Your Computer Class Class computer can use the all the features defined in an abstract class. public class Computer { private AbsUSBMemory usb; // more fields are here. void setUsb(AbsUSBMemory m) { usb = m; } public void editAfile() { usb.save(); } } <<Abstract>> AbsUSBMemory save() Computer usb 1 editAfile()
Still Need to wait.. No product based on AbsUSBMemory is available yet. Method save() is not implemented yet public class USBMemory extends AbsUSBMemory { public void save() { // Your implementation // is here } } USBMemory <<abstract>> AbsUSBMemory -brand:String void setBrand(String b) String getBrand() <<abstract>> void save() save()
Now you can buy your products You can buy your computer with a usb memory based on the features provided by AbsUSBMemory public class Test { public static void main(String[] args) { Computer myComputer = new Computer(); AbsUSBMemory myusb1 = new USBMemory(); myComputer.setUsb(myusb1); myComputer.editAfile(); } }
Similarly, New Product Available A new product called phone extends AbsUSBMemory and is available. public class Computer { private AbsUSBMemory usb; // more fields are here. void setUsb(AbsUSBMemory m) { usb = m; } public void editAfile() { usb.save(); } } <Abstract>> AbsUSBMemory save() Computer usb 1 editAfile() Phone save() Your Computer Class still works. No need to change!!!
Now you can buy your phone You can buy your computer with a usb memory based on the features provided by AbsUSBMemory public class Test { public static void main(String[] args) { Computer myComputer = new Computer(); Phone myusb1 = new Phone(); myComputer.setUsb(myusb1); myComputer.editAfile(); } }
Abstract Classes An abstract class cannot be instantiated, but other classes are derived from it. An Abstract class serves as a superclass for other classes. The abstract class represents the generic or abstract form of all the classes that are derived from it. A class becomes abstract when you place the abstract key word in the class definition. public abstract class ClassName 9-17
Abstract Methods An abstract method has no body and must be overridden in a subclass. An abstract method is a method that appears in a superclass, but expects to be overridden in a subclass. An abstract method has only a header and no body. AccessSpecifier abstract ReturnType MethodName(ParameterList); Example: Student.java, CompSciStudent.java, CompSciStudentDemo.java 9-18
Abstract Methods Notice that the key word abstract appears in the header, and that the header ends with a semicolon. public abstract void setValue(int value); Any class that contains an abstract method is automatically abstract. If a subclass fails to override an abstract method, a compiler error will result. Abstract methods are used to ensure that a subclass implements the method. 9-19