Understanding Object-Oriented Design Principles and Subtyping in Java
Explore the concepts of object-oriented design, patterns, and the strategy pattern. Learn how to translate user stories into classes and implement them in Java. Delve into the theory of Is-A, Has-A, and Can-Do relationships, and understand the importance of subtyping and supertyping in creating flexible and extensible code structures.
Uploaded on Oct 08, 2024 | 0 Views
Download Presentation
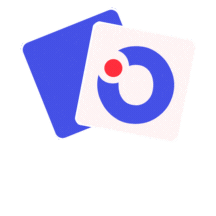
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Advanced OOD, Patterns and the Strategy Pattern 1
From Requirements to Object-Oriented Design As a user I want see my nearby friends on the map So that I know who to contact for a spontaneous meet-up 1. Identify the things me (previous user story) friends, friend map (previous, built-in) Identify the messages me.logged-in (previous) friend.location-known friend.location friend.online friend.near(me) friend.image Assemble into classes Right things call right messages (TBD) Given I am logged in And my location is known When my friends are online And my friends location is known And my friends are near me Then I see them on the map 2. class Friend { Location location(); // null > not-known boolean online(); Bitmap image(); } class Friends implements Iterable<Friend> { Friends online(); Friends locationKnown(); // > online? } 3. 4. 2
Object-Oriented Typing Rehash: Is-A vs Has-A vs Can-Do Theory of Object-Oriented, III 3
Typing and Subtyping: is a In Object-Oriented, it s all about behavior A particular behavior is described by a type Mammalian behavior is the Mammal type Tiger behavior is the Tiger type Because a Tiger always behaves like a Mammal, we say that Tiger is a subtype of Mammal Feline is asubtype of Mammal House Cat is asubtype of Feline s Subtype c means all s salways act like a c but not all c sact like an s All Felines are Mammals But not all Mammals are Felines Means that Feline object will always work wherever a Mammal is needed Mammal Feline Canine Tiger House Cat 4
Typing and Subtyping: is a (II) Feline a subtype of Mammal means Mammal a supertype of Feline A supertype variable can hold values of any of its subtypes void petFur(Mammal m) { int coarseness = m.furValue(); } Use of a supertype variable like Mammal allows all current and future subtypes of Mammal to be petted petFur will work for Canine subclass Dog when it is added We say that petFur is open for extension Mammal Feline Canine Tiger House Cat 5
Three Ways to Declare Subtype in Java Mammal Subclass a class classMammal { } class Feline extendsMammal { } Feline is a subtype of Mammal Feline gets all of Mammal s implementation (can override) Feline Canine Tiger House Cat Subclassing abstract class is similar Can t new an abstract C b/c not all methods implemented abstractclassMammal { } class Feline extendsMammal { } Feline must implement missing methods of Mammal Abstract class asserts I m a category of thing, not a real thing Interface has no methods implemented Like a totally abstract class interface Cuddly { } class HouseCat extends Feline implements Cuddly { } 6
More on Interfaces In Java, a class can extend just one superclass But a class can implement multiple interfaces interface Cuddly { } interfaceSpotted { } class Tabby extends Feline implements Cuddly, Spotted { } Means interfaces are much more flexible Mammal Can mix in all the supertypes you want Feline Canine Not just a tree of types anymore Spotted Cuddly Bengal Tiger Tabby 7
More on Interfaces, II Not all classes represent a unique type Maybe want another impl of same thing for performance, say Consider two possible impl s of Set class TinySet implements Set { /* linked list */ } class BigSet implements Set { /* uses hard disk */ } Identical interfaces, input/output behavior > same type One faster for tiny sets, the other faster for big sets Use interfaces for implementation alternatives Since TinySet real subtype, extends would be misleading Since no shared implementation, extends would be misleading 8
How represent Feline (and Why)? A. Class B. Abstract Class C. Interface D. Extends Abstract class allows reusing some common implementation among Felines, and yet cannot be new d (instantiated). Interface is a reasonable answer, too, as the sharing might not be too great. Mammal Feline Canine Tiger House Cat 9
Interfaces are Central to Java Java Collections Framework Interface Collection methods like add(Object) & contains(Object) interface List extendsCollection interface Set extendsCollection Wherever Collection variable appears, allows for a a ListArray or HashSet, etc. Requires no particular implementation Super-flexible code 10
Software Design Patterns An Introduction 11
Design Patterns, Generally? Consider designing a kitchen Common challenges Need a sink, a stove/oven, a refrigerator, likely a dishwasher Needs counter space, and don t want doors and drawers to collide Need space to stand Common patterns for a solution One-wall, Galley, L-shaped, U-Shaped, Peninsula, Working triangle (Refrigerator, range, sink) Same is true for basic bathroom Tub is 5 x 2.5-31, Sink is 2-2.5 , Toilet is 1.5-2 Overall minimum dimension is 5 x 7 Tub must go against wall can t cross Don t want to see toilet from door and toilets longer than sinks Standard solution Sink, Toilet, Tub, with door in front of sink. 13
Design Patterns, Generally? Bedrooms upstairs; Kitchen, Dining room, main room downstairs Privacy for family spaces, downstairs for entertaining Master bedroom on third floor Privacy for parents or guests One bedroom on lower level Gives privacy from family level Doubles as quiet room near activity, e.g. office, hobby room or den You see the idea Experience leads to an understanding of common challenges And common solutions to those challenges Education leads to learning about these without needing to experience them Learn to spot the common challenges and consider the common patterns for addressing them Also gives us a way of talking about things, e.g. Galley or Working Triangle or Master suite , or Multi-Purpose Den 14
Software Design Patterns The same is true of software Common challenges Good ways of addressing these challenges Great way of passing on and receiving experience Common vocabulary and building blocks for conversation Shared expectation about design and code structure Key Elements Name, or we can t talk about it Common, interesting problem Good, exemplar solution A teaching, i.e. what makes the pattern worth learning Consensus, agreement about above and acceptance into vernacular Just like patterns for the design of physical spaces, software design patterns aren t actual solutions to specific real problems, they are ideas that can be applied in many circumstances Idioms are implementations of patterns, i.e. in specific languages 15
Software Design Patterns Tricks Identifying common problems Separating common problems from context and arbitrary complexity For example, software design patterns aren t accepted work- around for language specific issues, these are idioms Good solutions, articulating teaching Promulgation and acceptance 16
Strategy Pattern Composition + Delegation + Interfaces 17
Strategy Pattern Name: Strategy Pattern, a.k.a. Policy Pattern Challenge: Runtime selection of mechanism for implementing a particular behavior, e.g. choosing the best algorithm for the circumstances Solution: Define the methods via an interface, that abstracts the behavior and its arguments from the implementation. At runtime, a reference via this interface can be associated with an instance with the proper implementation. Teaching: Interface references can enable interchangeable strategies, i.e. algorithms, at runtime. https://en.wikipedia.org/wiki/Strategy_pattern#/media/File:Strategy_Pattern_in_UML.png 18
Shopping Cart, continued class ShoppingCart { DiscountCalculator discountCalculator; int finalPriceCents () { return total * (1 discountCalculator.getDiscount()); } interface unsigned float DiscountCalculator { unsigned float getDiscount(); } class ClubCustomer implements DiscountCalculator { public unsigned float getDiscount() { return (1 MAX_DISCOUNT); } } class RetailCustomer implements DiscountCalculator { public unsigned float getDiscount() { unsigned float discount = points /POINTS_PER_DPERCENT); return (discount > MAX_DISCOUNT, 1 discount, 1 MAX_DISCOUNT); } 19
Shopping Cart class ShoppingCart { DiscountCalculator discountCalculator; public ShoppingCart(Customer c) { discount = c.getDiscountCalculator(); } int finalPriceCents () { return total * (1 discountCalculator.getDiscount()); } 20
Car Description: Whats Wrong? abstract class CarDesc { Color paintColor(); int wheelbase(); int tireSize(); boolean tirePerf(); String tireSeason(); /* should be enum */ } A. CarDesc and maybe SmallCar should be an interface B. All these classes should implement a Tire interface C. KiaSoul should have a Tire As implemented, KiaSoul only allows one set of values for tire behavior. By adding a Tire via an instance variable and delegating to its function, then all sorts of tires will beas an possible. (See next slide.) abstract class SmallCar extends CarDesc { } class KiaSoul extends SmallCar { int tireSize() { return 165; } boolean tirePerf() { return false; } String tireSeason() { return All ; } 21