Understanding Object-Oriented Programming Concepts
Object-oriented programming enables the effective development of large-scale software and GUIs by defining classes to represent entities in the real world as objects with unique identities, states, and behaviors. Objects have data fields representing their properties and methods defining their actions. Classes serve as templates for objects, allowing for instantiation and the use of constructors for object initialization.
Download Presentation
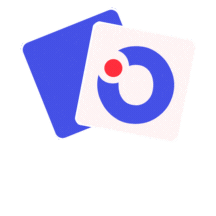
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Objects and Class Object Oriented Programming 1
Object Oriented Programming Object-oriented programming enables you to develop large-scale software and GUIs effectively. However, these Java features (whatever learnt so far) are not sufficient for developing graphical user interfaces and large-scale software systems. Suppose you want to develop a graphical user interface as shown below: Object Oriented Programming 2
Defining classes for objects A class defines the properties and behaviors for objects. An object represents an entity in the real world that can be distinctly identified. For example, a student, a desk, a circle, a button, and even a loan can all be viewed as objects. Object Oriented Programming 3
Cont. An object has a unique identity, state, and behavior. The state of an object (also known as its properties or attributes) is represented by data fields with their current values. A circle object, for example, has a data field radius, which is the property that characterizes a circle. A rectangle object has the data fields width and height, which are the properties that characterize a rectangle. The behavior of an object (also known as its actions) is defined by methods. To invoke a method on an object is to ask the object to perform an action. For example, you may define methods named getArea() and getPerimeter() for circle objects. A circle object may invoke getArea() to return its area and getPerimeter() to return its perimeter. You may also define the setRadius(radius) method. A circle object can invoke this method to change its radius. Object Oriented Programming 4
Cont. A class is a template, blueprint, or contract that defines what an object s data fields and methods will be. An object is an instance of a class. You can create many instances of a class. Creating an instance is referred to as instantiation. A Java class uses variables to define data fields and methods actions/behaviour. to define Object Oriented Programming 5
Cont. Additionally, a class provides methods of a special type, known as constructors, which are invoked to create a new object. A constructor can perform any action, but constructors are designed to perform initializing actions, such as initializing the data fields of objects. Object Oriented Programming 6
The above class is different from all the classes we have seen so far. The class which contains main method is called main class. Another way to write the same program SimpleCircle.java TestSimpleCircle.java Object Oriented Programming 8
Cont. You can put the more than one classes into one file, but only one class in the file can be a public class. Furthermore, the public class must have the same name as the file name. Therefore, the file name is TestSimpleCircle.java, since TestSimpleCircle is public. Each class in the source code is compiled into a .class file. When you compile TestSimpleCircle.java, two class files TestSimpleCircle.class and SimpleCircle.class are generated. Object Oriented Programming 9
Cont. In java file, more than one class may contain main method. So, we can invoke the main method of specific class as follow: >java a - this will call main method of class a >java b - this will call main method of class b Object Oriented Programming 10
Cont. Consider television sets. Each TV is an object with states (current channel, current volume level, power on or off) and behaviors (change channels, adjust volume, turn on/off). You can use a class to model TV sets. TestTV.java Object Oriented Programming 11
Constructor A constructor is invoked to create an object using the new operator. Constructors are a special kind of method. They have three peculiarities: A constructor must have the same name as the class itself. Constructors do not have a return type not even void. Constructors are invoked using the new operator when an object is created. Constructors play the role of initializing objects. Object Oriented Programming 12
Cont. A class normally provides a constructor without arguments (e.g., Circle()). Such a constructor is referred to as a no-arg or no- argument constructor. A class may be defined without constructors. In this case, a public no-arg constructor with an empty body is implicitly defined in the class. This constructor, called a default constructor, is provided automatically only if no constructors are explicitly defined in the class. Object Oriented Programming 13
Accessing object via reference variable An object s data and methods can be accessed through the dot (.) operator via the object s reference variable. Objects are accessed via the object s reference variables, references to the objects. Such variables are declared using the following syntax: ClassName objectRefVar; which contain Object Oriented Programming 14
Cont. Accessing data field and method using dot operator. Reference field are set default to null. Object Oriented Programming 15
Primitive type Vs Reference type Every variable represents a memory location that holds a value. Object Oriented Programming 16
Using Classes from Java Library The Java API contains a rich set of classes for developing Java programs. Date class: Object Oriented Programming 17
Cont. Random class: Math.random() Another way to generate random numbers is to use the java.util.Random class Object Oriented Programming 18
Cont. Point2D class: Java API has a conveninent Point2D class in the javafx.geometry package for representing a point in a two- dimensional plane Object Oriented Programming 19
Cont. Object Oriented Programming 20
Static variable, constant and methods The radius in circle1 is independent of the radius in circle2 and is stored in a different memory location. Changes made to circle1 s radius do not affect circle2 s radius,and vice versa. Object Oriented Programming 21
Cont. If you want all the instances of a class to share data, use static variables, also known as class variables. A static variable is shared by all objects of the same class. A static method cannot access instance members of the class. Static variables store values for the variables in a common memory location. Because of this common location, if one object changes the value of a static variable, all objects of the same class are affected. Java supports static methods as well as static variables. Static methods can be called without creating an instance of the class. Object Oriented Programming 22
Cont. TestCircleWithStaticMembers.java Object Oriented Programming 23
Visibility modifiers Visibility modifiers can be used to specify the visibility of a class and its members. You can use the public visibility modifier for classes, methods, and data fields to denote that they can be accessed from any other classes. If no visibility modifier is used, then by default the classes, methods, and data fields are accessible by any class in the same package. This is known as package-private or package-access. Object Oriented Programming 24
Cont. Packages can be used to organize classes. To do so, you need to add the following line as the first non comment and nonblank statement in the program: package packageName; If a class is defined without the package statement, it is said to be placed in the default package. Java recommends that you place classes into packages rather than using a default package. Object Oriented Programming 25
Cont. The private modifier makes methods and data fields accessible only from within its own class. Object Oriented Programming 26
Cont. If a class is not defined as public, it can be accessed only within the same package. Object Oriented Programming 27
Cont. There is no restriction on accessing data fields and methods from inside the class. Object Oriented Programming 28
Data field encapsulation Making data fields private protects data and makes the class easy to maintain. The data fields numberOfObjects CircleWithStaticMembers class in previous program can be modified directly. radius in and the Object Oriented Programming 29
Cont. This is not a good practice for two reasons: First, data may be tampered with. For example, numberOfObjects is to count the number of objects created, but it may be mistakenly set to an arbitrary value (e.g., CircleWithStaticMembers.numberOfObjects = 10). Second, you want CircleWithStaticMembers ensure that the radius is nonnegative after other programs have already used the class. to modify class the to Object Oriented Programming 30
Cont. A private data field cannot be accessed by an object from outside the class that defines the private field. So, how to make a private data field accessible: provide a getter method to return its value. To enable a private data field to be updated, provide a setter method to set a new value. A getter method is also referred to as an accessor and a setter to a mutator. TestCircleWithPrivateDataFields.java Object Oriented Programming 31
Program (The Fan class) Design a class named Fan to represent a fan. The class contains: Three constants named SLOW, MEDIUM, and FAST with the values 1, 2, and 3 to denote the fan speed. A private int data field named speed that specifies the speed of the fan (the default is SLOW). A private boolean data field named on that specifies whether the fan is on (the default is false). A private double data field named radius that specifies the radius of the fan (the default is 5). A string data field named color that specifies the color of the fan (the default is blue). The accessor and mutator methods for all four data fields. A no-arg constructor that creates a default fan. A method named toString() that returns a string description for the fan. If the fan is on, the method returns the fan speed, color, and radius in one combined string. If the fan is not on, the method returns the fan color and radius along with the string fan is off in one combined string. Write a test program that creates two Fan objects. Assign maximum speed, radius 10, color yellow, and turn it on to the first object. Assign medium speed, radius 5, color blue, and turn it off to the second object. Display the objects by invoking their toString method. Object Oriented Programming 32
Program (The Rectangle class) design a class named Rectangle to represent a rectangle. The class contains: Two private double data fields named width and height that specify the width and height of the rectangle. The default values are 1 for both width and height. A no-arg constructor that creates a default rectangle. A constructor that creates a rectangle with the specified width and height. The accessor and mutator methods for both data fields. They don t allow to set non-negative values. A method named getArea() that returns the area of this rectangle. A method named getPerimeter() that returns the perimeter. Write a test program that creates two Rectangle objects one with width 4 and height 40 and the other with width 3.5 and height 35.9. Display the width, height, area, and perimeter of each rectangle in this order. Object Oriented Programming 33
Passing object to methods Passing an object to a method is to pass the reference of the object. You can pass objects to methods. Like passing an array, passing an object is actually passing the reference of the object. Object Oriented Programming 34
Cont. Java uses exactly one mode of passing arguments: pass-by-value. In the preceding code, the value of myCircle is passed to the printCircle method. This value is a reference to a Circle object. TestPassObject.java Object Oriented Programming 35
Array of objects An array can hold objects as well as primitive type values. Circle[] circleArray = new Circle[10]; To initialize circleArray, you can use a for loop like this one: for (int i = 0; i < circleArray.length; i++) { circleArray[i] = new Circle(); } An array of objects is actually an array of reference variables. Object Oriented Programming 36
Immutable objects and classes Normally, you create an object and allow its contents to be changed later. However, occasionally it is desirable to create an object whose contents cannot be changed once the object has been created. We call such an object as immutable object and its class as immutable class. The String class, for example, is immutable. Object Oriented Programming 37
Cont. How to make class immutable? If you deleted the setter method in the CircleWithPrivateDataFields class, the class would be immutable, because radius is private and cannot be changed without a setter method. Object Oriented Programming 38
Scope of variable The scope of instance and static variables is the entire class, regardless of where the variables are declared. Instance and static variables in a class are referred to as the class s variables or data fields. A variable defined inside a method is referred to as a local variable. The scope of a class s variables is the entire class, regardless of where the variables are declared. A class s variables and methods can appear in any order in the class. Object Oriented Programming 39
Cont. You can declare a class s variable only once, but you can declare the same variable name in a method many times in different non-nesting blocks. If a local variable has the same name as a class s variable, the local variable takes precedence and the class s variable with the same name is hidden. Object Oriented Programming 40
The this reference The keyword this refers to the object itself. It can also be used inside a constructor to constructor of the same class. invoke another Object Oriented Programming 41
Cont. Using this to invoke constructor It can be used to refer instance variable of current class It can be used to invoke or initiate current class constructor It can be passed as an argument in the method call It can be passed as argument in the constructor call It can be used to return the current class instance Click to know the example link Object Oriented Programming 42
Object Oriented Thinking The focus of this chapter/topic is on class design and explores the differences between procedural programming and object-oriented programming. Four main concepts in OOPs are Abstraction, Encapsulation, Inheritance and Polymorphism (AEIP) Object Oriented Programming 43
Class abstraction Class abstraction is the separation of class implementation from the use of a class. The creator of a class describes the functions of the class and lets the user know how the class can be used. The collection of methods and fields that are accessible from outside the class, together with the description of how these members are expected to behave, serves as the class s contract. Object Oriented Programming 44
Cont. What is the need for Data Abstraction? : If you want to hide your business logic from the outside world. To achieve this implementation we can use Data Abstraction. TV or Car remote is assembled from the collection of circuits but they don't show to the user all circuits behind the remote, They only provide remote to the user to use it. When the user presses the key on remote the channel gets changed. They provide only necessary information to the user. Object Oriented Programming 45
Class encapsulation The details of implementation are encapsulated and hidden from the user. This is known as class encapsulation. Class abstraction and encapsulation are two sides of the same coin. Many examples in real life shows the concept of abstraction/encapsulation building computer system, mobile, car. Object Oriented Programming 46
Cont. What Encapsulation? : If you want to hide the complexity and provide the security to data. To achieve this implementation the oops concept came i.e. Data Encapsulation. Remote is assembled from the collection of circuits but they hide all circuits from the user. They encapsulate all circuits in one thing called remote and provide to the user to use it. And also remote can provide security to all circuits used inside the remote. is the need for Data Object Oriented Programming 47
Cont. Abstraction hides details at the design level, while Encapsulation hides details at the implementation level. Object Oriented Programming 48
Procedural lang. Vs. OOP lang. ComputeLoan.java That program cannot be reused in other programs because the code for computing the payments is in the main method. One way to fix this problem is to define static methods for computing the monthly payment and total payment. However, this solution has limitations. Suppose you wish to associate a date with the loan. There is no good way to tie a date with a loan without using objects. Object Oriented Programming 49
Cont. TestLoanClass.java Object Oriented Programming 50