Understanding C++ Data Types, Variables, and Assignments
Dive into the fundamentals of C++ programming with a focus on data types, variables, and assignments. Learn about the importance of type safety, computation input and output, declaring and initializing variables, and writing assignment statements in C++. Dr. Xiaolan Zhang from Fordham University provides insights into these key concepts, setting a solid foundation for your programming journey.
Download Presentation
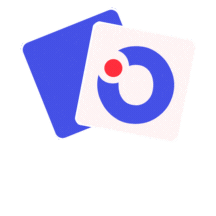
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
C++ Data Type and Type Safety Dr. Xiaolan Zhang Fordham University
Computation Input: from keyboard, files, other input devices, other programs, other parts of a program Computation what our program will do with the input to produce the output. Output: to screen, files, other output devices, other programs, other parts of a program Code (input) data (output) data data 3
Overview Variables and Assignments Data Types and Expressions Input and Output Program Style 4
C++ Variables A variable is some memory that can hold a value of a given type Each variable has name, type, value A declaration names a variable int a = 7; char c = 'x'; string s = "qwerty"; 7 a: c: 'x' 6 s: "qwerty" Size of memory varies for objects of different types ! 5
Declaring Variables Before use, variables must be declared Declaration syntax: type_name variable_1 , variable_2, . . . ; Tells the compiler: I need variable(s) named to store .. type of data int number_of_bars; double one_weight, total_weight; Try this: what does the above three declarations say? How about this? char response, option; 6
Assignment Statements An assignment statement changes the value of a variable total_weight = one_weight + number_of_bars; total_weight is set to the sum one_weight + number_of_bars Left hand side (LHS): variable whose value is to be changed Right hand side (RHS): new value for the LHS variable: Constants -- age = 21; Variables -- my_cost = your_cost; Expressions -- circumference = diameter * 3.14159; 7
Initializing Variables Declaring a variable does not give it a value Giving a variable its first value is initializing the variable Variables are initialized in assignment statements double mpg; // declare the variable mpg = 26.3; // initialize the variable Declaration and initialization can be combined Method 1 double mpg = 26.3, area = 0.0 , volume; Method 2 double mpg(26.3), area(0.0), volume; 8
Types C++ provides a set of types E.g. bool, char, int, double Called built-in types C++ programmers can define new types Called user-defined types We'll get to that eventually, mostly in CS2 C++ standard library provides a set of types E.g. string, vector, complex Technically, these are user-defined types they are built using only facilities available to every user 9
Literal constants int pennies = 8; cout << " Hello world\n "; Literal constants: values that occurs in the program (red part above) Literal, as we can only speak of it in terms of its value Constant: its value cannot be changed How to write literals? Depending on the type of the literal 8 is of type int, Hello world\n is of type string More examples: bool validInput = true; bool continue = false; // true, false are reserved words Character literals: 'a', 'x', '4', '\n', $ // use single quotation mark Integer literals: 0, 1, 123, -6, 0x34, 0xa3, 024 //0x means it s in base 16 Floating point literals: 1.2, 13.345, .3, -0.54, 1.2e3, . 3F, .3F String literals: "asdf", "Howdy, all y'all! " //double quotation mark 10
floating point constants: two forms floating point constants: two forms Simple form must include a decimal point e.g., 34.1 23.0034 1.0 89.9 Scientific Notation form e.g. 3.41e1 means 34.1 3.67e17 means 367000000000000000.0 5.89e-6 means 0.00000589 Number left of e does not require a decimal point Exponent cannot contain a decimal point 11
Types and Varaibles closer look o A type defines a set of possible values and a set of operations o A value is a sequence of bits in memory, interpreted according to its type o An object is a piece of memory that holds a value of a given type int a = 7; char c = 'x'; string s = "qwerty"; String object keeps the # of chars in the string, and the chars .. We will learn how to access each char, s[0], s[1], 7 a: c: x 6 qwerty s: 12
More example What s the difference? double x=12; string s= 12 ; 12 12 x: s: 2 1. x stores the value of number 12 s2 stores the two characters, 1 , 2 2. applicable operations are different x: arithmetic operations, numerical comparison, s: string concatenation, string comparison 13
value: a sequence of bits in memory o interpreted according to a type o E,g, int x=8; 8 x: is represented in memory as a seq. of binary digits (i.e., bits): 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 o An integer value is stored using the value s binary representation (demo this) In everyday life, we use decimal representation 14
value: a sequence of bits in memory (contd) o interpreted according to a type o E,g, char x= 8 ; 8 x: o is represented in memory as a seq. of binary digits (i.e., bits) 0 0 1 1 1 0 0 0 o A char value is stored using char s ASCII code 15
ASCII Code 16
A bit sequence (string) Interpretation o Given a bit string in memory 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 o If it s interpreted as integer, then it represents value 8 1*23=8 o If interpreted as char, there are two chars, a NULL char, and a BACKSPACE char 17
A technical detail In memory, everything is just bits; type is what gives meaning to the bits char c = 'a'; cout << c; // print the value of character variable c, which is a int i = c; cout << i; // print the integer value of the character c, which is 97 int i = c; Right-hand-side (RHS) is a value of char type is an int type variable Left-hand-side (LHS) Assign a char value to a int type variable ?! A safe type conversion ! Why? 18
Sizeof operator Yields size of its operand with respect to size of type char cout <<"sizeof bool is " << sizeof (bool) << "\n" <<"sizeof char is " << sizeof (char) << "\n" <<"sizeof int is " << sizeof (int) << "\n" <<"sizeof short is " << sizeof (short) << "\n" <<"sizeof long is " << sizeof (long) << "\n" <<"sizeof double is " << sizeof (double) << "\n" <<"sizeof float is " << sizeof (float) << "\n"; sizeof bool is 1 sizeof char is 1 sizeof int is 4 sizeof short is 2 sizeof long is 8 sizeof double is 8 sizeof float is 4 19
Char-to-int conversion char c = 'a'; cout << c; int i = c; cout << i; // print the value of character variable c, which is a c: 01100001 0000000000000000000000001100001 // print the integer value of the character c, which is 97 i: No information is lost in the conversion char c2=i; //c2 has same value as c Can convert int back to char type, and get the original value Safe conversion: bool to char, int, double char to int, double int to double 20
Type safety o Type safety is extent to which a programming language discourages or prevents type errors. o Ideally, every object will be used only according to its type A variable will be used only after it has been initialized Only operations defined for the variable's declared type will be applied Every operation defined for a variable leaves the variable with a valid value E.g., double x; //x is not initialized, // memory contains random bit string double y=x; //use uninitialized x 21
#include <iostream> using namespace std; int main() { int pennies = 8; int dimes = 4; int quarters = 3; double total = pennies * 0.01 + dimes * 0.10 + quarters * 0.25; // Total value of the coins cout << "Total value = " << total << "\n"; return 0; } //what if change 8 to "eight"? Implicit type conversion int to double 22
Type safety ENFORCEMENT o A language supports static type safety if A program that violates type safety will not compile Compiler reports every violation when you program, the compiler is your best friend o A language supports dynamic type safety, if a program that violates type safety it will be detected at run time Some code (typically run-time system") detects every violation not found by compiler 23
C++ Type safety o C++ is not (completely) statically type safe No widely-used language is (completely) statically type safe o C++ is not (completely) dynamically type safe Many languages are dynamically type safe o Being completely statically or dynamically type safe may interfere with the ability to express ideas and often makes generated code bigger and/or slower A trade-off ! o Most of what you ll be taught here is type safe We ll specifically mention anything that is not 24
A type-safety violation ( implicit narrowing ) // Beware: C++ does not prevent you from trying to put a large value // into a small variable (though a compiler may warn) int main() { int a = 20000; char c = a; int b = c; if (a != b) else } (demo2.cpp) Try it to see what value b gets on your machine, why? 20000 a ??? c: ?? b // != means not equal cout << "oops!: " << a << "!=" << b << '\n'; cout << "Wow! We have large characters\n"; 25
narrowing conversion int main() { double d =0; while (cin>>d) { // repeat the statements below as long as we type in numbers int i = d; // try to squeeze a double into an int char c = i; // try to squeeze an int into a char int i2 = c; // get the integer value of the character cout << "d==" << d // the original double << " i=="<< i // converted to int << " i2==" << i2 // int value of char << " char(" << c << ")\n"; // the char } } Demo: TestCode/narrow 26
A type-safety violation (Uninitialized variables) // Beware: C++ does not prevent you from trying to use a variable // before you have initialized it (though a compiler typically warns) int main() { int x; char c; double d; double dd = d; cout << " x: " << x << " c: " << c << " d: " << d << '\n'; } Always initialize your variables beware: debug mode may initialize valid exception to this rule: input variable // x gets a random initial value // c gets a random initial value // d gets a random initial value // not every bit pattern is a valid floating-point value // potential error: some implementations //can t copy invalid floating-point values 27
Explicit type conversion In lab quiz1, we calculate the running total, and average of numbers until -1 is entered * Link to solution for lab quiz1 To calculate the average, we divide total by the count How to obtain the average with decimal parts? Need to make one of the operands a double/float type short a=2000; int b; b = (int) a; // c-like cast notation b = int (a); // functional notation 28
Overview Variables and Assignments Data Types Expressions Input and Output Program Style 29
Input and Output A data stream is a sequence of data: in the form of characters or numbers An input stream is data for the program to use, originating from keyboard, a file An output stream is the program s output, destining to monitor, or a file , .. Include directives: add library files to our programs To make definitions of the cin and cout available : #include <iostream> Using directives: include a collection of defined names To make names cin and cout available to our program: using namespace std; 30
Output using cout cout is an output stream for program to send data to monitor insertion operator "<<" inserts data into cout cout << number_of_bars << " candy bars\n"; sends two items to monitor: value of number_of_bars, and quoted string constant No space added between items, therefore space before the c in candy, A blank space can also be inserted with cout << name << " " <<age <<endl ; A new insertion operator is used for each item of output same as cout << number_of_bars ; cout << " candy bars\n"; cout an expression directly cout << "Total cost is $" << (price + tax); 31
Formatting Output Escape sequences: tell the compiler to treat characters in a special way, allow one to specify special characters '\' is escape character To create a newline in output use \n, or endl; cout << "Hello\n"; cout << "Hello"<<endl; Other escape sequences: \t -- a tab \\ -- a backslash character \" -- a quote character When printing receipt, use \t to line up different columns Other ways possible 32
Formatting Real Numbers Real numbers (type double) produce a variety of outputs double price = 78.5; cout << "The price is $" << price << endl; output could be any of these: The price is $78.5 The price is $78.500000 The price is $7.850000e01 unlikely to get: The price is $78.50 33
Showing Decimal Places cout includes tools to specify the output of type double To specify fixed point notation setf(ios::fixed) To specify that decimal point will always be shown setf(ios::showpoint) To specify that two decimal places will always be shown precision(2) e.g.: cout.setf(ios::fixed); cout.setf(ios::showpoint); cout.precision(2); cout << "The price is $" << price << endl; 34
Input Using cin cin is an input stream bringing data from the keyboard extraction operator (>>) removes data to be used e.g., cout << "Enter the number of bars in a package\n"; cout << " and the weight in ounces of one bar.\n"; cin >> number_of_bars; // program will wait for input cin >> one_weight; code prompts user to enter data then reads two data items from cin first value read is stored in number_of_bars second value read is stored in one_weight Data is separated by spaces when entered 35
Reading Data From cin Multiple data items are separated by spaces (space, tab, newline) cin skips blanks and line breaks looking for data Data is not read until enter key is pressed Allows user to make corrections cin >> v1 >> v2 >> v3; Requires three space separated values User might type 34 45 12 <enter key> Or 34 <enter key> 45 <enter key> 12 <enter key> 36
Reading Character Data following reads two characters but skips any space that might be between char symbol1, symbol2; cin >> symbol1 >> symbol2; User normally separate data items by spaces J D Results are same if data is not separated by spaces JD 37
Designing Input and Output Prompt the user for input that is desired cout statements provide instructions cout << "Enter your age: "; cin >> age; Notice the absence of a new line before using cin Echo the input by displaying what was read Gives the user a chance to verify data cout << age << " was entered." << endl; 38
Overview Variables and Assignments Data Types Expressions Input and Output Constants 39
Program Style - Constants Literal constants: have no mnemonic value, i.e., no name total_price = large*14.82+small*12.34; cout << Hello world ; Cons: difficult to find and change when needed, harder to understand Named constants: give a name to a constant Allow us to change all occurrences simply by changing value of constant e.g.: const int WINDOW_COUNT = 10; declares a constant named WINDOW_COUNT const is keyword to declare a constant Its value cannot be changed by the program (unlike a variable) Common practice: name constants with all capitals 40