Understanding Classes and Objects in Python
Introduction to classes in Python: defining classes, creating objects, defining attributes and methods, class instantiation with __init__ method. Learn how to work with classes and objects in Python effectively.
Download Presentation
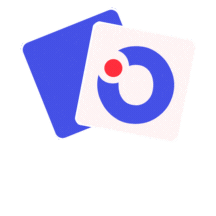
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Objectives O This chapter introduces 1. Introduction to a class Class design Class object Method object Class and instance variables 2. 3. 4. 5. 2
Classes O Classes provide a means of bundling data and functionality together O Creating a new class creates a new type of object, allowing new instances of that type to be made O Each class instance can have attributes attached to it for maintaining its state O Class instances can also have methods (defined by its class) for modifying its state 3
Class Definition O Class definitions, like function definitions (def statements) must be executed before they have any effect O The statements inside a class definition will usually be function definitions, but other statements are allowed O When a class definition is left normally (via the end), a class object is created class ClassName: <statement-1> <statement-N> 4
Class Objects O Class objects support two kinds of operations: attribute references and instantiation O Attribute references use the standard syntax used for all attribute references in Python: obj.name obj.name class MyClass: i = 12345 def f(self): return hello world O MyClass.i and MyClass.f are valid attribute references, returning an integer and a function object 5
Class Objects O Class instantiation uses function notation O Just pretend that the class object is a parameterless function that returns a new instance of the class class MyClass: i = 12345 def f(self): #It will call Myclass.f(obj) return hello world obj = MyClass() O The above code creates a new instance of the class and assigns this object to the variable obj obj 6
Class Objects O Many classes like to create objects with instances customized to a specific initial state O A class may define a special method named __init__() O When a class defines an __init__() method, class instantiation automatically invokes __init__() for the newly created class instance class MyClass: def __init__(self): #It will call Myclass.__init__(obj) print('hello') 7 obj = MyClass() # hello will be printed when the class instance is created
Method Objects objfunc = obj.f while True: print(objfunc()) #It will continue to print hello world until the end of time class MyClass: i = 12345 def f(self): return hello world obj = MyClass() O In the MyClass example, obj.f the string 'hello world O However, it is not necessary to call a method right away O obj.f is a method object, which can be stored away and called at a later time obj.f() () will return 8
Method Objects objfunc = obj.f while True: print(objfunc()) #It will continue to print hello world until the end of time class MyClass: i = 12345 def f(self): return hello world obj = MyClass() O A method object is created by packing the instance object and the function object just found together in an abstract object O When the method object is called with an argument list, a new argument list is constructed from the instance instance object object and and the the argument function object is called with this new argument list O obj.f() is equivalent to MyClass.f(x) the argument list list, and the 9
Class and Instance Variables O Instance variables Instance variables are for data unique to each instance O Class variables Class variables are for attributes and methods shared by all instances of the class shared by all instances of the class class Dog: kind = canine # class variable def __init__(self, name): self.name = name # instance variable class variable shared by all instances instance variable unique to each instance d = Dog('Fido') e = Dog('Buddy') 10
Class and Instance Variables class Dog: kind = canine # class variable def __init__(self, name): self.name = name # instance variable class variable shared by all instances instance variable unique to each instance d = Dog('Fido') e = Dog('Buddy') O d.kind and e.kind have the same string 'canine' O Because class variable is shared by all instances O d.name and e.name are 'Fido and 'Buddy , respectively O Because instance variable unique to each instance11
Class and Instance Variables class Dog: kind = canine # class variable def __init__(self, name): self.name = name # instance variable class variable shared by all instances instance variable unique to each instance d = Dog('Fido') e = Dog('Buddy') O Instance variables need not be declared O Like local variables O They spring into existence when they are first assigned to O For example d.age = 15 print(d.age) del d.age 12
Examples class MyClass: def __init__(self, num): self.attr1 = num obj1 = MyClass(10) obj2 = MyClass(20) print(obj1.attr1) #output 10 print(obj2.attr1) #output 20 13
Examples class MyClass: attr2 = 5 def __init__(self, num): self.attr1 = num MyClass.attr2 += 1 #Use classname to access class variables obj1 = MyClass(10) print(obj1.attr2) #output 6 obj2 = MyClass(20) print(obj1.attr2) #output 7 print(obj2.attr2) #output 7 print(MyClass.attr2) #output 7 14
Examples class Person: count = 0 def __init__(self, name, height): self.name = name self.height = height Person.count += 1 users = {} users['Andy'] = Person('Andy', 150) print(users['Andy'].name, users['Andy'].height, users['Andy'].count) users['Bob'] = Person('Bob', 176) users['Mary'] = Person('Mary', 180) users['Andy'].height += 20 users['Bob'].name = 'John' for i in sorted(users.keys()): print(users[i].name, users[i].height, users[i].count) 15 15
Default Methods O The classes in python provide several default methods O __str__() O The __str__ method in Python represents the class objects as a string class Person: def __init__(self, name , age): self.name = name self.age = age def __str__(self): return "Hi! My name is " + self.name + ".\nI'm " + str(self.age) + " years old." Output: 16 person1 = Person('John', 24) print(person1)
Default Methods O __del__() O The __del__() method is referred to as a destructor method O It is called after collection occurs, which happens after all references to the item have been destroyed. an object s garbage class Person: def __init__(self, name , age): self.name = name self.age = age print(self.name + ': Hi!') def __del__(self): print(self.name + ': Goodbye!') person1 = Person('John', 24) del person1 17 Output:
Exercise O Please design a program that enables the following instructions: O Input 0: Exit program O Input 1: Insert student data, including ID, name, and score O Input 2: Delete student data by the specified ID O Input 3: Update student data by the specified ID O Input 4: Show all student information 18
Source O References: O https://docs.python.org/3/tutorial/classes.ht ml 19