Understanding Python Dictionaries: Key-Value Mapping
Python dictionaries are key-value mappings where elements are stored as key-value pairs instead of being indexed by numbers like in sequences. Dictionaries allow unique keys and provide efficient ways to create, access, and update key-value pairs in Python programming. Learn how to utilize dictionaries effectively to manage data in your Python projects.
- Python Data Structures
- Key-Value Mapping
- Python Dictionary Methods
- Accessing Dictionary Items
- Python Programming
Download Presentation
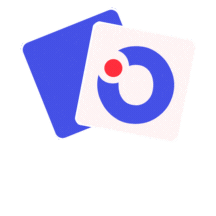
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Module 5 Module 5 Part 2 Part 2 Mapping Types
Dictionaries Dictionaries Python only has one mapping type: Dictionaries Unlike sequences which have their elements indexed by number, Dictionaries index their elements by keys Items in a Dictionary are stored as key-value pairs. Where one specific key maps to a specific value Keys are always unique
Dictionaries Dictionaries Creating Creating # creates an empty dictionary age = {} # creates a dictionary with some key-value pairs ages = { Alice : 30, Bob : 40, Charlie : 50} #creates a dictionary using dictionary comprehension numbers = {x: x ** 2 for x in range(10)} # creates a dictionary using the dictionary constructor ages = dict(Alice = 30, Bob = 40, Charlie = 50)
Dictionaries Dictionaries Key Key- -Value pairs Value pairs In the example below, we have a dictionary with 3 items (3 key-value pairs) ages = { Alice : 30, Bob : 40, Charlie : 50} The keys present in this dictionary are Alice , Bob , and Charlie The Alice key is associated with the value 30 The Bob key is associated with the value 40 The Charlie key is associated with the value 50
Dictionaries Dictionaries Accessing Accessing Given that the values in a dictionary are indexed by keys and not by numbers, we must use the keys to access a particular value ages = { Alice : 30, Bob : 40, Charlie : 50} print(age[ Alice ]) # prints 30 Like with lists, we can replace the value associated with a key by doing an assignment age[ Alice ] = 60 # replaces Alice value with 60
Dictionaries Dictionaries Accessing Accessing We can check if a key exists in a dictionary much like how we would check if an item exists in a sequence print( Eve in ages) # will print False Trying to read from a non-existent key is an error print(ages[ Eve ]) # crashes your program Trying to assign to a non-existent key adds that key ages[ David ] = 70 # key David has been added
Dictionaries Dictionaries Accessing Accessing Printing a dictionary gives you all the key-value pairs in dictionary form # prints {'Alice': 30, 'Bob': 40, 'Charlie': 50} print(ages) Converting the dictionary into a list gives you all the keys inside that list # prints ['Alice', 'Bob', 'Charlie'] print(list(ages))
Dictionaries Dictionaries Accessing Accessing We can use the dictionary s values() to retrieve a list of all the values in a dictionary # prints [30, 40, 50] print(list(ages.values)) We can use the dictionary s items() to retrieve all key-value pairs. Notice that they will be stored inside of tuples # prints [('Alice', 30), ('Bob', 40), ('Charlie', 50)] print(list(ages.items()))
Dictionaries Dictionaries Accessing Accessing We can use the dictionary s values() to retrieve a list of all the values in a dictionary # prints [30, 40, 50] print(list(ages.values)) We can use the dictionary s items() to retrieve all key-value pairs. Notice that they will be stored inside of tuples # prints [('Alice', 30), ('Bob', 40), ('Charlie', 50)] print(list(ages.items()))
Summary Summary Sequence types can any number of elements of different types Elements in a Sequence are always ordered, but may not be sorted Lists can vary in size once created Strings, Tuples and Ranges cannot vary in size once created You can access one or more elements in a Sequence