Introduction to Python Programming: Basics and Arithmetic Operations
Learn the fundamentals of Python programming with a focus on setting up the development environment, understanding the Python shell, working with arithmetic expressions, data types, and numerical operations. Explore operator precedence and solve simple arithmetic expressions to grasp the basics of Python programming.
Download Presentation
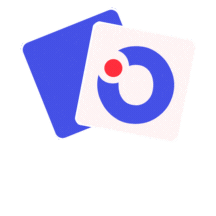
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Intro to Programming using Python Lecture #1 Yosef Mendelsohn With many thanks to Dolores Kalayta for the original version of these notes. 1
Getting started with Python Install Python on your computer The latest release of Python version 3 Download the Python software from: https://www.python.org/download Select the appropriate installer for your operating system (Windows, Linus/Unix, Mac OS X) Find and click on the IDLE shortcut 3
The Python shell Whether in Command Window or IDLE, Python is waiting for us to say something >>> (this is the Python prompt) In this mode, Python will instantaneously INTERPRET our statements and return an answer This is known as "interactive mode". As opposed to 'script mode' Interactive mode means that each statement is executed once you hit 'enter' 4
Open IDLE What are the expected results for: >>> 5 + 1.3 >>> 5 1.3 >>> 5 * 1.3 >>> 5 / 1.3 >>> 8 - 9.3 --> Note the unusual result >>> 2 ** 3 5
Arithmetic expressions, data types and functions 6
Data Types / Python Numeric values Integer Values without a decimal point Python data type int Floating point Values with a decimal point Python data type float 7
Numerical Operations / Symbols Operation Symbol Addition + Subtraction - Multiplication * Division (returns float) / Division Quotient (returns int) // Division Remainder (returns int) % Exponentiation ** 8
Operator precedence () ** * / // % + - What does (4-1) + 2 * 3 ** 2 evaluate to? 9
Result Data Type DT Op DT Result DT int + - * int int int / int float int // int int int % int int float % float float int ** int int float ** int float This information may seem pedantic, but it's not! Be sure that you are clear on the concept. 10
Practice For each of the following expressions predict the result and it s data type; then execute the expression in IDLE to determine if you prediction is correct.idle 4 + 2 4.0 + 2 6 * 7 9/3 6 * 7 3 2.5 / (1 + 4) 6.2 + 8 * 3 10/5 (6.2 + 8) * (3 10/5) 11
Built-in functions abs(x) Returns the absolute value of a number float(x) Returns a floating point number constructed from a number format(value [, format_spec]) Convert a value to a formatted representation, as controlled by format_spec int(x) Returns an integer value constructed from a number min (arg1, arg2, ) Returns the smallest of two or more arguments max(arg1, arg2, ) Returns the largest of two or more arguments round(number[, ndigits]) Returns the floating point value number rounded to ndigits after the decimal point https://docs.python.org/3/library/functions.html 12
Python Standard Library This is a standard library of functions that are present with every installation of Python. As your programming needs become more advanced, you may need to install additional libraries separately. Python has many, many built-in functions as part of the standard library. Because there are so many functions, we organize them into groups calls 'modules'. In order to use functions that are in a certain module, we must first import that module. For example, if we wanted to use some more advanced mathematical functions that live in a module called 'math', we would first type: import math Numeric and Mathematical Modules math fractions random Try it: The area of a circle is pi * radius2 Suppose you have r=5, get Python to output the area. Solution is in the PPT notes. >>> import math >>> math.pi >>> 3.141592653589793 13
Variables 14
Variables Suppose we performed a complex calculation in Python and we wanted to SAVE the result for future use In order to do that we need to have a container in memory where we can store our piece of data Such containers are called variables 15
Variables In the code below, we have two variables, one that holds a value in pounds, and a second that holds a value in kilograms. pounds = 163 kilograms = pounds / 2.2 print(kilograms) Try it: Create a variable called 'dollars' and assign it a value of 50. Then create a variable called 'euros' and assign it a value of dollars*0.84, and output the result. Solution is in the notes below. 16
Variables: assignment statements Formula for calculating compound interest A is the value after t periods P is the principal amount (initial investment) r is the annual nominal interest rate ( not reflecting the compounding) n is the number of times the interest is compounded per year t is the number of years the money is invested Given: n = 12 months, t = 10 years, P = 5000, r = 1/100 Write a line of code to determine the value and assign it to the variable A 17
Variables: assignment statements We have done the following in memory for each variable: An object (container) was created for the variables For the first variable, we put a label n on the object The type label of the object 'n' is int The number 12 was stored as this object t n r 10 12 .01 Main Memory P A 5000 5525.624479061677 What is the type for label A? 20
Legal Python variable names A variable name a.k.a. identifier, can be made up of Letters Digits The underscore character _ Can not begin with a digit Can be as long as you want Variable names are case sensitive EVERYTHING in Python is case sensitive! MyStuff and mystuff are entirely different variables Awareness of case sensitivity in Python is very important! Most commonly used programming languages are case sensitive 21
Reserved words Besides variable names, that we make up, there are other words that are used in Python These special identifiers are called reserved words . They are words that already have a predefined meaning / usage in Python A reserved word cannot be used as an identifier (e.g. as a variable name) 22
Python reserved words and as assert break class continue def del elif else except false finally for from global if import in is int lambda none nonlocal not or pass raise return true try while with yield 23
Multi-word variable names There are two conventions for names with multiple words: Using underscore as delimiter shoe_size Using camelCase capitalizing the initial of the second (third, ) word shoeSize You can choose one style and stick with it Be consistent within each program 24
Go to IDLE Click File New File If you have not already saved the program, click OK and give this file a name. When you click save the program will execute 26
IDLE Shell The output is returned to the IDLE Shell 27
Variables: assignment statements Formula for calculating compound interest A is the value after t periods P is the principal amount (initial investment) r is the annual nominal interest rate ( not reflecting the compounding) n is the number of times the interest is compounded per year t is the number of years the money is invested Given: n = 12 months, t = 10 years, p = 5000, r = 1/100 28
compound_interest_v1.py RESULTS: ======= RESTART: C:\Users\ymendels\Dropbox\401\compound_interest_v1.py ======= The investment balance is 5525.62 The interest amount is 525.62 >>> 29
Practice Problem You scored x/100, y/100, z/100 on homework What is your total homework percentage? 30
Create algorithm We want the program to use 3 variables and calculate the average percentage Step 1: Assign a value to score_1 Step 2: Assign a value to score_2 Step 3: Assign a value to score_3 Step 4: Calculate Average by summing the scores and dividing by total possible points Step 5: Multiply Average by 100 to get percentage Step 6: Print percentage 31
Strings 32
Strings A string value is represented as a sequence of characters enclosed within quotes A string value can be assigned to a variable. String values can be manipulated using string operators and functions 33
Explicit conversion of int to string Adding two or more strings together to make a longer string is known as concatenation This is used a lot in programming Only string data can be concatenated To change an int data type to a string use str() Example: >>> age = 16 >>> print('Adam is ' + age + ' years of age') TypeError: must be str, not int >>> print ('Adam is ' + str(age) + ' years of age') Adam is 16 years of age 34