Understanding Flow Control in Java
In Java programming, flow control determines the sequence in which instructions are executed. This involves sequential execution, branching with conditional statements, handling multiple instructions in one branch, nested branching, and cascaded branching. Learn how to control program flow effectively in Java with the help of various examples and illustrations.
Download Presentation
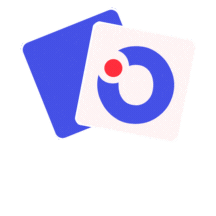
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Controlling which instruction to execute next Sequential Similar to walking, one step after another Branching Similar to a fork in the road Depending on the destination, you choose one way or the other, not both Repetition Similar to running on a track in the Olympics Repeating the same track in a loop
Sequential x = 1; x = x + 1; As expected First instruction first Second instruction second What if we swap the two instructions? That is Instructions cannot be in arbitrary order
Branching (Conditional Statements) if ( x < y ) x = x + 1; // execute if true else y = y * 10; // execute if false // boolean condition
Second/Else Branch is Optional if ( x < y ) // boolean condition x = x + 1; // execute if true
Multiple Instructions in One Branch if ( x < y ) { // note the matching braces x = x + 1; y = y x; } else { y = y * 10; x = y / x; }
Nested Branching if ( x < y ) { x = x + 1; if ( y > 10) y = y x; } else { y = y * 10; x = y / x; }
Cascaded Branching if (score >= 90) grade = A ; else if (score >= 80) grade = B ; else if (score >= 70) grade = C ; else if (score >= 60) grade = D ; else grade = F ;
Version 2: always correct answer? if (score >= 90) grade = A ; if (score >= 80) grade = B ; if (score >= 70) grade = C ; if (score >= 60) grade = D ; if (score < 60) grade = F ;
Version 3: always correct answer? if (score >= 90) grade = A ; if (score >= 80 && score < 90) grade = B ; if (score >= 70 && score < 80) grade = C ; if (score >= 60 && score < 70) grade = D ; if (score < 60) grade = F ;
Repetition (looping) for counting loops frequently (sometimes inappropriately) used while general loops most flexible do-while ( Repeat until ) at least once least used
ICU Initialize (start) What is the initial/starting condition? Continue (or stop) When to continue/stop? In what condition does it continue/stop? Update How to update the condition? If ICU is not carefully designed (common mistake) your program will be in ICU
Counting loop 1, 2, 3, 10 for (int num = 1; num <= 10; num++) System.out.println(num); for (initialize; continue; update) body -- instruction(s) to be repeated Continue --boolean (continue if true, stop if false)
How about from 55 to 123? for (int num = ?; ?; ?) System.out.println(num);
How about from 55 to 123? for (int num = 55; num <= 123; num++) System.out.println(num);
How about 10 numbers from 55? for (int num = ?; ?; ?) System.out.println(num);
How about 10 numbers from 55? // version 1? for (int num = 55; num <= 64; num++) System.out.println(num); // version 2? for (int num = 55; num <= 65; num++) System.out.println(num); // version 3? for (int num = 55; num < 65; num++) System.out.println(num);
How about 10 even numbers from 2? for (int num = ?; ?; ?) System.out.println(num);
How about 10 even numbers from 2? // version 1? for (int num = 2; num <= 20; num=num+2) System.out.println(num); // version 2? for (int num = 2; num <= 18; num=num+2) System.out.println(num); // version 3? for (int num = 2; num < 20; num=num+2) System.out.println(num);
How about 10 even numbers down from 100? for (int num= ? ; ?; ?) System.out.println(num);
How about 10 even numbers down from 100? // version 1? for (int num=100; num >= 80; num=num-2) System.out.println(num); // version 2? for (int num=100; num >= 82; num=num-2) System.out.println(num); // version 3? for (int num=100; num > 82; num=num-2) System.out.println(num);
Anything that is strange? for (int num=10; num < 10; num++) System.out.println(num);
Anything that is strange? for (int num=10; num < 10; num++) System.out.println(num); continue is never true, body never executes
Anything that is strange? for (int num=10; num >= 10; num++) System.out.println(num);
Anything that is strange? for (int num=10; num >= 10; num++) System.out.println(num); Continue is always true, infinite loop (eventually stops since int has an upper limit and num overflows)
Finding Sum of 1 to 10 int sum = 0; for (int num = 1; num <= 10; num++) sum = sum + num;
Finding Sum of 1 to 10 int sum = 0; for (int num = 1; num <= 10; num++) sum = sum + num; // --- version 2 ? --- int sum = 0; for (int num = 1; num < 10; num++) sum = sum + num;
Sum of first 10 even numbers int sum = 0; for (int num = ?; ? ; ?) sum = sum + num;
Sum of first 10 even numbers int sum = 0; for (int num = 0; num <= 18; num = num + 2) sum = sum + num;
Printing a Line of 5 Stars for (int star = 1; star <= 5; star++) { System.out.print( * ); } System.out.println(); // new line // --- output: --- *****
Printing a Line of 5 Stars for (int star = 1; star <= 5; star++) { System.out.print( * ); } System.out.println(); // new line // --- version 2 ? --- for (int star = 0; star < 5; star++) { System.out.print( * ); } System.out.println(); // new line
4x5 Rectangle of Stars ?? for (int star = 1; star <= 5; star++)//5 stars { System.out.print( * ); } System.out.println(); // --- Output: --- ***** ***** ***** *****
4x5 Rectangle of Stars nested loop for (int line = 1; line <= 4; line++) //4 lines { for (int star = 1; star <= 5; star++)//5 stars { System.out.print( * ); } System.out.println(); } // --- Output: --- ***** ***** ***** *****
Triangle of Stars * ** *** **** *****
While loop int num = 1, sum = 0; while (num <= 10) { sum = sum + num; num++; } initialize while (continue) // repeat if continue is true { update }
A program with an exit command boolean exit = false; while (exit == false) { // do stuff if ( //exit command is entered ) exit = true; }
Is num a prime number? Definition Only divisible by 1 or itself Check divisors between 2 and num 1 to see if num is divisible by divisor Don t need to check divisors larger than num
Checking different divisors Initialize Start divisor with 2 Continue Divisor is less than num and num is not divisible by divisor Update Increment divisor
Checking different divisors // I: start divisor with 2 int divisor = 2; // C: divisor less than num and // num not divisible by divisor while (divisor < num && num % divisor != 0) { divisor++; // U: increment factor }
Print the answer as well // I: start divisor with 2 int divisor = 2; // C: divisor less than num and // num not divisible by divisor while (divisor < num && num % divisor != 0) { divisor++; // U: increment factor } System.out.println(?);
Print the answer as well // I: start divisor with 2 int divisor = 2; // C: divisor less than num and // num not divisible by divisor while (divisor < num && num % divisor != 0) { divisor++; // U: increment factor } if (divisor == num)//not divisible by smaller divisors System.out.println( prime ); else System.out.println( not prime );
Which do you prefer? int divisor = 2; int divisor = 2; boolean prime = true; while (divisor < num && num % divisor != 0) { divisor++; } while (divisor < num) { if (num % divisor == 0) prime = false; divisor++; } if (divisor == num) System.out.println( prime ); else System.out.println( not prime ); if (prime == true) System.out.println( prime ); else System.out.println( not prime );
Do-While loop Execute the loop body at least once continue is checked after the loop body is executed initialize do { update } while (continue); // repeat if continue is true // note the semicolon at the end
Checking input int numTickets = 0; do { System.out.print( Please enter # of tickets: ); numtickets = keyboard.nextInt(); } while (numTickets <= 0);
Checking Password String username = , password = ; do { System.out.print( Please enter password: ); password = keyboard.next(); } while (!valid(username, password)); System.out.print( Please enter username: ); username = keyboard.next();
How to add at most 3 Trials? String username = , password = ; do { System.out.print( Please enter password: ); password = keyboard.next(); } while (!valid(username, password)); System.out.print( Please enter username: ); username = keyboard.next();
At most 3 Trials String username = , password = ; int trials=0; do { System.out.print( Please enter username: ); username = keyboard.next(); System.out.print( Please enter password: ); password = keyboard.next(); trials++; } while (!valid(username, password) && trials < 3);