Overview of Java Socket Programming and GUI APIs
Java Socket Programming facilitates data sharing between computing devices through client-server communication using sockets. This tutorial covers topics on Socket Programming, establishing socket connections, and a demo showcasing client-server interactions. Additionally, it explores GUI APIs like AWT, Swing, JavaFX, and their evolution in Java development.
Download Presentation
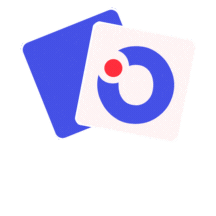
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Java Tutorial Zhe Li
Topics Socket Programming GUI Maven Project(Tomcat)
Socket Programming Java socket programming provides facility to share data between different computing devices. A socket is an endpoint between two way communication.(Server and Client) Overview
Socket Programming Client Server Socket Class ServerSocket Class public Socket accept() Socket socket = new Socket( 127.0.0.1 , 5000) public InputStream getInputStream() public synchronized void close() public OutputStream getOutputStream() public synchronized void close()
Establish a Socket Connection A socket connection means the two machines have information Socket socket = new Socket( 127.0.0.1 , 5000) about each other s network location (IP Address) and TCP port. Communication public InputStream getInputStream() To communicate over a socket connection, streams are used to both input and output the data. public OutputStream getOutputStream() Closing the connection The socket connection is closed explicitly once the message to public synchronized void close() server is sent.
socket demo Feature1: Feature2: Client keeps reading input from user and sends to the server until Over is typed. Client will write first to the server then server will receive and print the text. Server prints out what the client sends to it. Then server will send the message back to the client and the client will receive and print the text.
GUI Java APIs for graphic programming: AWT(Abstract Windowing Toolkit) Swing JavaFX HTML/CSS, Jquery, JavaScript Graphical User Interface
GUI AWT API was introduced in JDK 1.0. Swing API, a much more comprehensive set of graphics libraries that enhances the AWT, was introduced as part of Java Foundation Classes (JFC) after the release of JDK 1.1. JFC consists of Swing, Java2D, Accessibility, Internationalization, and Pluggable Look-and-Feel Support APIs. JFC has been integrated into core Java since JDK 1.2. The latest JavaFX, which was integrated into JDK 8, is meant to replace Swing.
AWT java.awt package contains the core AWT graphics classes: java.awt.event package supports event handling: GUI Component classes, such as Button, TextField, and Label. Event classes, such as ActionEvent, MouseEvent, KeyEvent and WindowEvent GUI Container classes, such as Frame and Panel Event Listener Interface, such as ActionListener, MouseListener, MouseMotionListener Custom graphic classes, such as Graphic, Color and Font Event Listener Adapter classes, such as MouseAdapter, KeyAdapter, and WindowAdapter
Awt demo It has a top-level container Frame, which contains three components - a Label "Counter", a non-editable TextField to display the current count, and a "Count" Button. The TextField shall display count of 0 initially. Each time you click the button, the counter's value increases by 1.
Swing If you understood the AWT programming (in particular, container/component and event-handling), switching over to Swing is straight-forward. Compared with the AWT component classes (in package java.awt), Swing component classes (in package javax.swing) begin with a prefix "J", e.g., JButton, JTextField, JLabel, JPanel, JFrame, or JApplet.
Writing Swing Applications In summary, to write a Swing application, you have: 1. 2. Use the Swing components with prefix "J" in package javax.swing, e.g., JFrame, JButton, JTextField, JLabel, etc. A top-level container (typically JFrame) is needed. The JComponents should not be added directly onto the top-level container. They shall be added onto the content-pane of the top-level container. You can retrieve a reference to the content-pane by invoking method getContentPane() from the top-level container. Swing applications uses AWT event-handling MouseEvent/MouseListener, etc. Run the constructor in the Event Dispatcher Thread (instead of Main thread) for thread safety, as shown in the following program template. 3. classes, e.g., ActionEvent/ActionListener, 4.
Swing demo Let's convert the earlier AWT application example into Swing. Compare the two source files and note the changes (which are highlighted). The display is shown below. Note the differences in look and feel between the AWT GUI components and Swing's
HTML+iQuery(Web-based) HTML jQuery HyperText Markup Language Manipulation of html elements Purpose: How the website will look like(Layout)
jQuery(Library) jQuery is a library used primarily for Document Object Model (DOM) manipulation. The DOM is a tree-like structure that represents all elements on a webpage. jQuery can select DOM elements, create animations, handle events, and more. Its goal is to be extensible, simple, and clear to use. It takes care of all cross-browser incompatibilities, and it promotes the separation of HTML and JavaScript.
Bootstrap (framework) Bootstrap is an open-source front-end framework to help you with designing websites. It features a number of CSS styles, icons, components, and JavaScript plugins. These plugins include a lot of common things you might use when building a website. This includes modals, dropdowns, alerts, and buttons, to name a few.
AngularJS HTML is great for declaring static documents, but it falters when we try to use it for declaring dynamic views in web-applications. Data-binding eliminates DOM manipulation. Controllers are the behavior behind the DOM elements.
Maven Project Maven is a build automation tool used primarily for Java projects. It addresses two aspects of building software: First, it describes how software is built, and second, it describes its dependencies.
Demo http://javalin-websocket-example.herokuapp.com/
tips about tomcat Tomcat version Mvn clean install