Java Programming Exercises Week 1 to Week 4 Solutions
Solutions and explanations for Java programming exercises from Week 1 to Week 4. Includes code fragments, explanations of Java variable names, calculations, error corrections, user input, and more.
Download Presentation
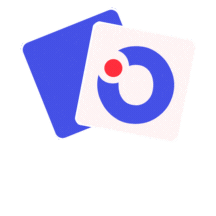
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Solutions to Exercises Week 1 to Week 4
Notes on the Solutions I use the following abbreviations in code: sout System.out.println sop System.out.print Those need to be expanded to make valid Java code. The answers are program fragments just pieces of the programs the rest of the required parts of a Java program need to be added to get the solutions to run! These answers are suitable for tests you can use the same abbreviations your answers will also be program fragments
Week 2, Slide #28 Is your surname a valid Java variable? Yes, unless it has a space or punctuation (e.g. hyphen or apostrophe) Young yes; O'Toole no; Apple-Dumpling no; von Beethoven no If not, what's wrong? O'Toole apostrophe; Apple-Dumpling hyphen; von Beethoven space If so, is it a variable name or a class name Class name unless it starts with a small letter Young class; Klein class; Khosraviani class; Chin class
Week 2, Slide #43 Write a program fragment to report how much 25 boxes of girl guide cookies would cost, given that each box costs $2. howMany = 25; pricePer = 2; total = howMany * pricePer; sout("Your " + howMany + " boxes of cookies cost you $" + total + ".");
Week 2, Slide #50 Fin the errors: To find the area of a circle 1. get it s diameter 2. raduis = diameter / pi 3. area = pi * radius * squared Syntax error: it's its Syntax error: raduis radius Logic error: pi 2 Syntax error: squared radius
Week 2, Slide #65 Ask the user what year it is and how old they are. Then calculate and print the year they were born. Use variables and suitable output. sop("What year is it? "); year = kbd.nextInt(); kbd.nextLine(); sop("How old are you? "); age = kbd.nextInt(); kbd.nextLine(); born = year age; sout("You were born in about " + born + ".");
Week 2, Slide #71 2 * 2 + 3 * 4 = PEDMAS Multiplication is before Addition otherwise left-to-right b) 4 + 3 * 4 = 4 + 12 = 16 5 / 3 1 = PEDMAS Division is before Subtraction a) 1 1 = 0
Week 2, Slide #78 Say what value gets assigned int j; double f; f = 3; f = f + 2.6; j = (int)f; f = (double)j / 2 + 1.1; f = (double)(j / 2) + 1.1; j f __ __ 5 __ __ 3.0 5.6 __ 3.6 3.1
Week 3, Slide #9 if grade is greater than or equal to 50, print out You passed! if (grade >= 50) { sout("You passed!); } if age is less than 18, print out I m sorry, to see this movie. if (age < 18) { sout("I'm sorry, but you're not allowed to see this movie."); } if guess is equal to secretNumber, print out You guessed it! if (guess == secretNumber) { sout("You guessed it!); } if y plus 9 is less than or equal to x times 2, set z equal to zero. if (y + 9 <= x * 2) { z = 0; }
Week 3, Slide #13 What is the output of the following code? int age = 24; int height = 180; int weight = 70; int income = 120000; if (age < 18) { if (height > 180) { if (weight <= 50) { if (income >= 100000) { System.out.println("Young!"); } System.out.println("Tall!"); } System.out.println("Thin!"); } System.out.println("Rich!"); } Rich!
Week 3, Slide #28 Change if (answer.toUpperCase().startsWith("Y")) so that it only accepts abbreviations of YES as Yes . but still allow capital letters Y, y, YE, ye, YES, yes, Ye, yE, YeS, Yes, Accept anything that's an abbreviation of YES abbreviation it's the first one, two or three letters of "YES" So, "YES" starts with what the user said if ("YES".startsWith(answer.toUpperCase())) note: also accepts empty string! Should maybe fix that .
Week 3, Slide #35 if temp is over 30, print Go swimming ; otherwise print Play video games if (temp > 30) { sout("Go swimming"); } else { sout("Play video games"); } if count is not zero, print sum divided by count; otherwise print No numbers entered . if (count != 0) { sout(sum / count); } else { sout("No numbers entered"); } if answer starts with a y (or Y), then set price to $10; otherwise set it to $15. if (answer.toUpperCase().startsWith("Y")) { price = 10; } else { price = 15; } if num is even, set it equal to n divided by 2; otherwise set it to n times 3, plus 1 if (n % 2 == 0) { n = n / 2; } else { n = n * 3 + 1; }
Week 3, Slide #38 Possible outcomes: outcome #1: A B C E F outcome #2: A D E F What is the code? sop("A "); if (?) { sop("B C "); } else { sop("D "); } sop("E F "); Possible outcomes: outcome #1: A B C D outcome #2: A B What is the code? sop("A B "); if (?) { sop("C D "); }
Week 3, Slide #39 Possible outcomes: outcome #1: A B C F G outcome #2: A D E F G outcome #3: A B C F outcome #4: A D E F What is the code? sop("A "); if (?) { sop("B C "); } else { sop("D E "); } sop("F "); if (?) { sop("G "); }
Week 3, Slide #49 (a) Write nested if/if-else to generate: either "A", "AB", "ABC" or "ABD" sop("A"); if (?) { sop("B"); if (?) { sop("C"); } else { sop("D"); } }
Week 3, Slide #49 (b) Write nested if/if-else to generate: either "A", "ABC" or "AC" sop("A"); if (?) { if (?) { sop("B"); } sop("C"); }
Week 3, Slide #49 (c) Write nested if/if-else to generate: either "A", "ABC", or "D" if (?) { sop("A"); if (?) { sop("BC"); } } else { sop("D"); }
Week 3, Slide #53 Write Boolean expressions for whether x is greater than 0 and y is less than 5 x is greater than zero or y is equal to z answer does not start with N it s not the case that x is greater than the product of y and z NOTE: translate directly to Java y is greater than x and less than z x is equal to y but not to z m or n is greater than 0 x > 0 && y < 5 x > 0 || y == z !answer.startsWith("N") !(x > y * z) y > x && y < z x == y && x != z m > 0 || n > 0
Week 3, Slide #60 Write Good morning if time < 12, Good afternoon if 12 time < 17, Good evening otherwise if (time < 12) { sout("Good morning"); } else if (time < 17) { sout("Good afternoon"); } else { sout("Good evening"); } Write Small if size is 1, Medium if size is 2, Large if size is 3, X- Large if size is 4, and XX-Large if size is 5 don t write anything if size is not 1..5 no else at the end! if (size == 1) { sout("Small"); } else if (size == 2) { sout("Medium"); } else if (size == 3) { sout("Large"); } else if (size == 4) { sout("X-Large"); } else if (size == 5) { sout("XX-Large"); }
Week 3, Slide #67 Create a variable named saidYes; Ask user if they are an adult; Set saidYes to true or false (true if they said Yes (ignoring case), false otherwise) boolean saidYes; sop("Are you an adult? "); String answer = kbd.nextLine(); saidYes = answer.equalsIgnoreCase("YES"); Can you do it without using any variables for what the user typed in? boolean saidYes; sop("Are you an adult? "); saidYes = kbd.nextLine().equalsIgnoreCase("YES");
Week 4, Slide #30 Track the variables values a = 1; a++; b = a + 6; b--; c = b * 5; c += a; a *= 10; b /= 2; c %= 4; a *= b + c; a 1 2 20 80 b ? c ? 8 7 35 37 3 1
Week 4, Slide #44 Declare Java constants for the maximum allowable enrolment for a course (which is 100) public static final int MAX_ENROLMENT = 100; the number of feet in a mile (5280) public static final int FEET_PER_MILE = 5280; the number of cm in an inch (2.54) public static final double CM_PER_INCH = 2.54; the name of a data file (numbers.txt) public static final String DATA_FILE_NAME = "numbers.txt";
Week 4, Slide #47 Write a loop to print out Hello 10 times (each Hello on its own line) for (int i = 1; i <= 10; ++i) { sout("Hello"); } Write a loop to print out the numbers from 1 to 20 on a single line separate the numbers by spaces end the line when you re done for (int i = 1; i <= 20; ++i) { sop(i + " "); } sout();
Week 4, Slide #49 Change this loop into a loop to find the minimum value int max, n; max = Integer.MIN_VALUE; for(n = 1; n <= N; ++n) { num = kbd.nextInt(); max = Math.max(max, num); } int min, n; min = Integer.MAX_VALUE; for (int n = 1; n <= N; ++n) { num = kbd.nextInt(); min = Math.min(min, num); }
Week 4, Slide #50 Change this loop to be data-controlled stop when negative number is entered int max, n; max = Integer.MIN_VALUE; num = kbd.nextInt(); while (num >= 0) { max = Math.max(max, num); num = kbd.nextInt(); } int max, n; max = Integer.MIN_VALUE; for(n = 1; n <= N; ++n) { num = kbd.nextInt(); max = Math.max(max, num); }
Week 4, Slide #56 Write a loop to read in six grades print Good work! if that grade is 90+ for (int a = 1; a <= 6; ++a) { sop("Enter your grade on A" + a + ": "); grade = kbd.nextInt(); if (grade >= 90) { sout("Good work!"); } } Enter your grade on A1: 100 Good work! Enter your grade on A2: 80 Enter your grade on A3: 95 Good work! Enter your grade on A4: 78 Enter your grade on A5: 84 Enter your grade on A6: 90 Good work!
Week 4, Slide #62 Write nested loops to read numbers (integers) until a negative number is read print that many Hello s on one line num = kbd.nextInt(); while (num >= 0) { for (int h = 1; h <= num; ++h) { sop("Hello"); } num = kbd.nextInt(); }
Week 4, Slide #64 Write nested loops to read three valid assignment scores and calculate their average valid = between 0 and 100 (inclusive) for (int a = 1; a <= 3; ++a) { sop("Enter your A" + a + " score: "); grade = kbd.nextInt(); while (grade < 0 || grade > 100) { sout("That's not valid. Try again!"); sop("Enter your A" + a + " score: "); grade = kbd.nextInt(); } sum += grade; } average = (double) sum / 3;