Android Permissions Overview
This detailed overview provides insights into the various permissions categories in Android, including location, hardware, services, Bluetooth, accounts, text messaging, user information, phone calls, and miscellaneous permissions like voicemail access and system functionalities. Each section highlights specific permissions granted within that category, emphasizing the importance of understanding permissions for app development and user privacy.
Download Presentation
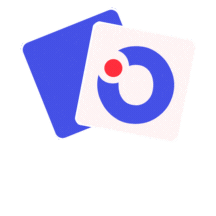
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Cosc 5/4730 Permissions
note each new android version has added new permissions, removed others. so this lecture is not complete and some maybe version dependent. such Bluetooth for example added a set of 4 new ones and removed the old ones at api 32. specific permissions will be listed with the lectures on a each topic.
Permissions Note that all of start with android.permission. Location: ACCESS_COARSE_LOCATION, ACCESS_FINE_LOCATION, ACCESS_LOCATION_EXTRA_COMMANDS, ACCESS_MOCK_LOCATION About cell Network ACCESS_NETWORK_STATE, ACCESS_WIFI_STATE, CHANGE_NETWORK_STATE, CHANGE_WIFI_MULITCAST_STATE, CHANGE_WIFI_STATE, INTERNET (needed to use networking) Hardware: BATTERY_STATS, CALL_PHONE, CAMERA, MODIFY_AUDIO_SETTINGS, NFC, READ_EXTERNAL_STORAGE, WRITE_EXTERNAL_STORAGE, RECEIVE_BOOT_OCMPLETED, RECORD_AUDIO, VIBRATE, WAKE_LOCK (prevent screen from dimming and lock), Varying services BIND_INPUT_METHOD (InputMethodService), BIND_REMOTEVIEWS (RemoteViewsService), BIND_TEXT_SERVICE (TextService like spellcheckerservice), BIND_VPN_SERVICE (VpnService), BIND_WALLPAPER (WallpaperService) Bluetooth BLUETOOTH, BLUETOOTH_ADMIN, Accounts: (AccountManager) GET_ACCOUNTS, MANAGE_ACCOUNTS, USER_CREDENTIALS Text messaging READ_SMS, RECEIVE_SMS, RECEIVE_MMS, SEND_SMS, WRITE_SMS
Permissions (2) User information : READ_CALENDAR, WRITE_CALENDAR, READ_CONTACTS, WRITE_CONTACTS, READ_USER_DICTIONARY, WRTIE_USER_DICTIONARY READ_HISTORY_BOOKMARKS, WRITE_HISTORY_BOOKMARKS, READ_SOCIAL_STREAM, SUBSCRIBE_FEEDS_READ, SUBSCRIBE_FEEDS_WRITE, WRITE_SOCAIL_STREAM, READ_SYNC_SETTINGS, READ_SYNC_STATS, WRITE_SYNC_SETTINGS, SET_ALARM, USE_SIP, WRITE_SETTINGS (system settings), RECEIVE_WAP_PUSH, SET_TIME_ZONE SET_WALLPAPER, SET_WALLPAPER_HINTS READ_PROFILE and WRITE_PROFILE (user s personal profile data) Phone calls: READ_PHONE_STATE, READ_CALL_LOG, WRITE_CALL_LOG, PROCESS_OUTGOING_CALLS Mostly depreciated as of API 30 (android 11) for CallScreeningService. Running applications (and recent apps too). GET_TASKS, REORDER_TASKS
Other Misc Permissions ADD_VOICEMAIL Added in API level 14, app must have access to a voicemail server. Constant Value: "com.android.voicemail.permission.ADD_VOICEMAIL" DISABLE_KEYGUARD, EXPAND_STATUS_BAR FLASHLIGHT, GET_PACKAGE_SIZE KILL_BACKGROUND_PROCESSES Added in API level 8 Allows an application to call killBackgroundProcesses(String)
Other permissions. I skipped over all the system permissions. Says, Not for use by third-party applications. in the documentation. Your app must be a system level app, which you can t do without rooting your phone or becoming a vendor, like say Motorola or Samsung. http://stackoverflow.com/questions/14256687/how-to-make-my- application-system
Calendar Example Once a calendar is connected to the device You can use the following example from google to read/write to the calendar Uses the Calendar Provider http://developer.android.com/guide/topics/providers/calendar -provider.html
Contacts Example Same with contacts http://developer.android.com/guide/topics/providers/contacts -provider.html
User Dictionary Android use the user dictionary for the example in the content provider basics example. http://developer.android.com/guide/topics/providers/content- provider-basics.html
AccountManager Provides a centralized registry of the user online accounts Example: Facebook, dropbox, Evernote, etc There are some examples of how to use and create your account for your online service. http://www.finalconcept.com.au/article/view/android-account-manager- step-by-step http://developer.android.com/reference/android/accounts/AccountManager. html
Incoming/outgoing phone calls deprecated as of API 29. Uses a callscreeningservice, that is built into the app dialer, until api 29 which is buggy, but can be separate. Uses: PROCESS_OUTGOING_CALLS and READ_PHONE_STATE The example uses two Broadcast Receivers You can do it in one, but split for the example. Able to get the phone number and the information. http://androidlabs.org/short-experiments/broadcast-receivers/process- outgoing-calls/ This example also shows you have to change the incoming phone number! http://code.google.com/p/krvarma-android- samples/source/browse/trunk/DetectCalls/?r=37
Incoming/outgoing phone calls (2) You can block outgoing phone calls You use: setResultData(null); That will stop the outbound call! http://stackoverflow.com/questions/599443/hang-up-outgoing-call-in-android You can not drop/ignore incoming calls thought. Requires the MODIFY_PHONE_STATE permission, which is a system level permission. http://stackoverflow.com/questions/15012082/rejecting-incoming-call-in-android http://androidsourcecode.blogspot.in/2010/10/blocking-incoming-call-android.html
CallScreeningService Started in API 24 But had to be part of the dialer. As of API 29 (android 10), the screener could be separate. Allows you disallow incoming calls, plus reject (hang up), no notifications, and no logging in the dialer in API 29 added no ring option, which is a standard option. https://developer.android.com/reference/kotlin/android/telec om/CallScreeningService
android.xml file <service android:name="your.package.YourCallScreeningServiceImplementation" android:permission="android.permission.BIND_SCREENING_SERVICE"> <intent-filter> <action android:name="android.telecom.CallScreeningService"/> </intent-filter> </service>
main activity and permissions. private static final int REQUEST_ID = 1; You'll need to request permission to be the screener. So something like this: public void requestRole() { RoleManager roleManager = (RoleManager) getSystemService(ROLE_SERVICE); Intent intent = roleManager.createRequestRoleIntent(RoleManager.ROLE_CALL_SCREENING); startActivityForResult(intent, REQUEST_ID); } @Override public void onActivityResult(intrequestCode, int resultCode, Intent data) { if (requestCode == REQUEST_ID) { if (resultCode == android.app.Activity.RESULT_OK) { // Your app is now the call screening app } else { // Your app is not the call screening app } } }
The service. public class myCallScreeningService extends CallScreeningService { public myCallScreeningService() {} //constructor @Override public void onScreenCall(@NonNull Call.Details callDetails) { if (callDetails.getCallDirection() == Call.Details.DIRECTION_INCOMING) { String num = callDetails.getHandle().getSchemeSpecificPart(); String phonenumber = PhoneNumberUtils.formatNumber(num, Locale.getDefault().getCountry()); //now make a decision on how to responsetocall if (callDetails.getCallDirection() == Call.Details.DIRECTION_OUTGOING) { //you can't change out going calls, but you can look the numbers and stuff. } else { //it's an unknown direction?
respondToCall(callDetails, ); The callDetails, must match what you sent. So now the reponse: CallResponse.Builder myCallReponse = new CallResponse.Builder(); myCallReponse .setSilenceCall(true/false) don't ring. Stand alone option. API 29+, the rest are API 24+ .setDisallowCall(true/false) disallow the call this one seems to need to be true for the rest below to work. .setRejectCall(true/false) reject the call, with disallow, ends the call. .setSkipCallLog(true/false) With disallow, also don't log the call .setSkipNotification(true/false); with disallow, also no notification of the call (which really doesn't show if reject). respondToCall(callDetails, myCallReponse.build() );
Task lists RunningApp in the Demo shows how to get the Running Applications. It s pretty simple. Get the ActivityManager from the service You can get RunningServices, RunningAppProcesses, RunningTasks, and RecentTasks. My example will list the package name for each one in the list. Reference: http://stackoverflow.com/questions/5446565/android- how-do-i-check-if-activity-is-running
Reorder Tasks(2) While facebook uses the reorder_tasks permission, I can t find a single example for it. What I did find an interesting article on how all the activities (tasks) work on the back to front of the stack. And managing how your activities are launched with the launch modes.
Wallpaper Get the instance of the wallpaper manager. Then use setBitmap, setResource, or setStream methods to change the wallpaper The example (setwallpaper) is very simple and uses a wallpaper in the resource directory.
Read/Write profile As of JellyBean (Api 14+) you can have several user profiles. And we have methods to read and write these profiles. It s handled through the Contracts, so you will need at least READ_CONTACTS permission as well and READ_PROFILE and WRITE_PROFILE
Read/Write profile The profile is handled with a ContentProvider. So to get the entire profile (I think every profile) you need the following query: Cursor c = getContentResolver().query( ContactsContract.Profile.CONTENT_URI, null, null, null, null);
Read profile example The readPofile project reads all the profiles on the device and displays the information to the screen, using the query before. My example is heavy modified, but based on http://android- codelabs.appspot.com/resources/tutorials/contactsprovider/e x1.html
QA &