Understanding Lists and Collections in Java
Discover how to utilize the List interface, ArrayLists, and LinkedLists in Java to efficiently store, manipulate, and iterate over collections of data. Learn the fundamental operations such as adding, getting, changing, and removing elements from a list, as well as exploring different methods provided by the Collections class. Gain insights into the nuances of List ADT and interfaces, and grasp the importance of choosing between ArrayList and LinkedList based on efficiency considerations.
Download Presentation
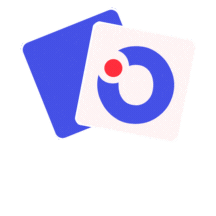
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Lists and Collections the List interface ArrayLists and LinkedLists Sets and the Collections class
Outcomes Use a List in place of an Array create an ArrayList or a LinkedList add, get, change, and remove elements loop thru it using for and for-each loops Loop thru a List using a ListIterator add and remove elements as you loop Recognize other kinds of Collections Use Collections class methods
Lists are for Listing Things What do you want to do with a list? add stuff to it print it out look to see what s on it check how long it is check if some particular thing s on it remove stuff from it List interface has methods for all those and lots more!
List ADT and Interface What is a list? sequence of things, all the same type (1stitem, 2nditem, 3rditem, ..., last item) java.util.List interface is parameterized say what kind of objects are allowed on it list of Strings: list of Files: list of integers: NOTE: List<int> is not allowed List<String> myWords; List<File> myFiles; List<Integer> myNumbers;
List Data Types List is an interface OK for variables but not for objects Two kinds of List objects (*) ArrayList LinkedList used exactly the same way! choose based on efficiency considerations explained in data structures course both from java.util List<String> var; var = new List<String>(); var = new ArrayList<String>(); var = new LinkedList<String>(); (*)There are actually more kinds of Lists, but those two are the usual ones to use
The Diamond Operator Actually, don t need to say String twice Java knows the second <> same as the first List<String> list1 = new ArrayList<>(); knows it s an array list of Strings List<Integer> list2 = new LinkedList<>(); knows it s a linked list of Integers List<Rectangle> list3 = new ArrayList<>(); knows it s an array list of Rectangles list4 = new ArrayList<>(); knows it s an array list of whatever list4 is a List of
Missing Diamond Do not forget to put in the diamond List<String> oops = new ArrayList(); for historical reasons it compiles (no squigglies) it will almost always work anyway but sometimes results will be different so always put in the diamond List<String> ok = new ArrayList<>();
Side-by-Side List<String> list; list = new ArrayList<>(); List<String> list; list = new LinkedList<>(); list.add("ten"); list.add("twenty"); list.add("thirty"); System.out.println(list); list.remove("twenty"); System.out.println(list); list.add("ten"); list.add("twenty"); list.add("thirty"); System.out.println(list); list.remove("twenty"); System.out.println(list); [ten, twenty, thirty] [ten, thirty] [ten, twenty, thirty] [ten, thirty]
Creating a List and Adding to it Normal variable + new object declaration List<String> myWords = new ArrayList<String>(); List starts out empty Add method to add items (to end of list) myWords.add("Ten"); myWords.add("Twenty"); can also say where to add to the list myWords.add(1, "Fifteen"); skip over 1 item, then add Fifteen myWords "Ten" "Ten", "Twenty" "Ten", "Fifteen", "Twenty"
List Objects Grow List objects start empty not like array objects array has a length when you create it array elements are initialized (to 0 if nothing else) Will grow as long as you keep adding not like array objects array has a length when you create it that s its length as long as it exists
Printing Out a List Lists can be printed directly! System.out (to screen) or a PrintWriter (to file) System.out.println("The list is " + myWords + "."); Output just like Arrays.toString( ) elements printed using their toString method The list is [Ten, Fifteen, Twenty].
Getting List Elements Lists use zero-based indexing just like arrays String firstWord = myWords.get(0); System.out.println("The first word is " + firstWord + "."); myWords firstWord "Ten", "Fifteen", "Twenty" "Ten" The list is [Ten, Fifteen, Twenty]. The first word is Ten.
Checking its Length Method called size instead of length not like arrays int size = myWords.size(); System.out.println("Last is " + myWords.get(size-1) + "."); myWords size "Ten", "Fifteen", "Twenty" 3 The list is [Ten, Fifteen, Twenty]. The first word is Ten. Last is Twenty.
Looking for Particular Items Is it there at all? contains Where exactly is it? indexOf if (myWords.contains("Twenty")) { System.out.println("We have a Twenty!"); System.out.println("It s at location " + myWords.indexOf("Twenty") + "."); } We have a Twenty! It s at location 2.
Looking for Particular Items Is it there at all? contains Where exactly is it? indexOf if (myWords.contains("Hundred")) { System.out.println("We have a Hundred!"); } System.out.println("The location of the Hundred is " + myWords.indexOf("Hundred") + "."); We have a Twenty! It s at location 2. The location of the Hundred is -1.
Removing Stuff What thing to remove, or which position? int argument remove from that position myWords.remove(1); System.out.println(myWords); object argument remove that object myWords.remove("Ten"); System.out.println(myWords); [Ten, Twenty] [Twenty]
Changing List Elements Use the set method to change a value give the location and the new value System.out.println(myWords); myWords.set(0, Thirty ); System.out.println( The list is now + myWords + . ); [Twenty] The list is now [Thirty].
Looping thru a List Multiple ways to loop thru a list can use the usual for loop for (int i = 0; i < myWords.size(); i++) can use this simplified for loop (for-each loop) for (String word : myWords) They work if you re just looking at the list can cause trouble if you re adding or removing from the list at the same time!
Usual for Loop for (int i = 0; i < allMyWords.size(); i++) { System.out.println("\t" + i + ") " + allMyWords.get(i)); } allMyWords "Ten", "Fifteen", "Twenty", "Thirty", "Fifty" 0) Ten 1) Fifteen 2) Twenty 3) Thirty 4) Fifty
Simplified for Loop for (String word : allMyWords) { System.out.println("\t" + word); } allMyWords "Ten", "Fifteen", "Twenty", "Thirty", "Fifty" Ten Fifteen Twenty Thirty Fifty
Why Use Arrays/Lists? Lists are better than arrays if: don t know how many elements are needed will be adding/removing list elements Arrays are better than Lists if: you know how many elements you ll need or a good upper bound you won t be adding/removing elements except at the end
In Particular... When reading data, you often don t know how many elements there will be user may not know, either! use List + while instead of array + for List<String> words = new ArrayList<String>(); data = kbd.next(); while (!data.equals(".")) { words.add(data); data = kbd.next(); } int num = kbd.nextInt(); String[] arr = new String[num]; for (int i = 0; i < num; ++i) { arr[i] = kbd.next(); }
Arrays.asList Arrays.asList makes a List from values List<String> limited = Arrays.asList("a", "b", "c"); or from an array String[] strArr = new String[]{"a", "b", "c"}; List<String> limited = Arrays.asList(strArr); But it s a fixed-length List cannot add or remove elements program will crash if you add/remove elements so not as useful as an ArrayList or a LinkedList
Exercise Write a code fragment that reads a single line of words and adds them all to a List, then prints out the list Enter a line of words below: This is the line of words I entered. The words you entered were: [This, is, the, line, of, words, I, entered.]
Exercise Make this program using Lists N heats; top 2 advance What order did they finish in heat 1? Jill Anne Leslie Freida What order did they finish in heat 2? Carol Louisa Judith Annette What order did they finish in heat 3? Carla Giselle Lois Rachel What order did they finish in heat 4? Yvonne Darla Brenda The people who advanced are: [Jill, Anne, Carol, Louisa, Carla, Giselle, Yvonne, Darla] Not advancing were: [Leslie, Freida, Judith, Annette, Lois, Rachel, Brenda]
List Iterators To add or remove items while looping List Iterator goes thru list one item at a time like a Scanner going thru a file: next and hasNext can remove the item you just looked at can add item beside the one you just looked at Can also use it for changing items or just to look at the items
Creating a List Iterator Parameterized, just like List & ArrayList just ask the list for one ListIterator<String> it = allMyWords.listIterator(); no new ListIterator<String>() type needs to be the same as the List s type allMyWords "Ten", "Fifteen", "Twenty", "Thirty", "Fifty" it &
Looping thru the List hasNext: is there is another item? next: get the next item (and advance) while (it.hasNext()) { System.out.println(it.next()); } allMyWords Ten Fifteen Twenty Thirty Fifty "Ten", "Fifteen", "Twenty", "Thirty", "Fifty" it &
Removing with an Iterator Delete items that start with F while (it.hasNext()) { String word = it.next(); if (word.startsWith("F")) it.remove(); } allMyWords word word word word word word "Ten" "Fifteen" "Twenty" "Thirty" "Fifty" "Ten", "Fifteen", "Twenty", "Thirty", "Fifty" it &
ListIterators Can Go in Reverse Start at the last element use previous & hasPrevious public static void writeListReversed(List<String> list) { ListIterator<String> it = list.listIterator(list.size()); while(it.hasPrevious()) { System.out.println("\t" + it.previous()) } } not as clumsy as the for loop version
Removing with an Iterator Going backwards is the same! while (it.hasPrevious()) { String word = it.previous(); if (word.startsWith("F")) it.remove(); } allMyWords word word word word word word "Fifty" "Thirty" "Twenty" "Fifteen" "Ten" "Ten", "Fifteen", "Twenty", "Thirty", "Fifty" it &
Removing with an Iterator Which item did you just look at? That s the one that gets removed. General policy check to see if there is a next/previous save the next/previous into a variable check the variable to see if it needs removed if so, use the iterator s remove method to remove it
Changing with an Iterator Same as removing but we re just changing instead of removing General policy check to see if there is a next/previous save the next/previous into a variable check the variable to see if it needs changed if so, use the iterator s set method to change it
Changing with an Iterator Change words starting with F to upper case! while (it.hasPrevious()) { String word = it.previous(); if (word.startsWith("F")) it.set(word.toUpperCase()); } allMyWords "FIFTEEN", word word word word word word "Fifty" "Thirty" "Twenty" "Fifteen" "Ten" "FIFTY" "Ten", "Fifteen", "Twenty", "Thirty", "Fifty" it &
Adding with an Iterator Adds towards the front of the list if going forward, then iterator will not see them "Ten", "Fifteen", "Twenty", "Thirty", "Fifty" "Ten", "A", "Fifteen", "Twenty", "Thirty", "Fifty" "Ten", "A", "B", "Fifteen", "Twenty", "Thirty", "Fifty" it & if going backward. iterator will see them "Ten", "Fifteen", "Twenty", "Thirty", "Fifty" "Ten", "Fifteen", "Twenty", "Thirty", "A", "Fifty" "Ten", "Fifteen", "Twenty", "Thirty", "B", "A", "Fifty" it &
Exercise Use an iterator to go thru a List<Integer>, removing every negative number and changing every 0 to a 42 go from front to back How would it change if we wanted to go from back to front?
List Interface More List interface methods isEmpty() clear() addAll(otherList) containsAll(otherList) removeAll(otherList) retainAll(otherList) subList(from, to) check if list is empty make list empty add all these check if contains these remove all these keep only these get part of the list
Check if a List is Empty Can use myList.size() == 0 but this is a bit easier and clearer S.o.p("List is now " + myList); if (myList.isEmpty()) else S.o.p("My list is empty"); S.o.p("My list isn't empty"); List is now [Ten, Fifteen, Twenty, Thirty, Fifty] My list isn't empty
Clear a List Make this list empty again removes every element from the list if (myList.isEmpty()) else myList.clear(); if (myList.isEmpty()) else S.o.p("My list is empty"); S.o.p("My list isn't empty"); S.o.p("Now my list is empty"); S.o.p("My list still isn't empty"); My list isn't empty Now my list is empty
Add Elements from Another List For adding many elements at once combine two lists S.o.p("Section A: " + sectionA); S.o.p("Section B: " + sectionB); List<String> combined = new ArrayList<String>(); combined.addAll(sectionA); combined.addAll(sectionB); S.o.p("Combined: " + combined); Section A: [Bill, Carol, Laura] Section B: [Ann, Don, Ed, Fran] Combined: [Bill, Carol, Laura, Ann, Don, Ed, Fran]
Check for Several Elements Are these all there or not? S.o.p("Combined: " + combined); if (combined.containsAll(sectionA)) S.o.p("All section A s in the combined list"); if (sectionA.containsAll(combined)) S.o.p("Everyone from the combined list is in section A"); else S.o.p("Someone in the combined list is not in section A"); Combined: [Bill, Carol, Laura, Ann, Don, Ed, Fran] All section A s in the combined list Someone from the combined list is not in section A
Remove Multiple Elements Take these away, if they re there no problem if they re not there S.o.p("Combined: " + combined); sectionA.add("Gil"); S.o.p ("Section A: " + sectionA); combined.removeAll(sectionA); S.o.p ("Combined: " + combined); Combined: [Bill, Carol, Laura, Ann, Don, Ed, Fran] Section A: [Bill, Carol, Laura, Gil] Combined: [Ann, Don, Ed, Fran]
Retain Multiple Elements Keep only these, if they re there no problem if they re not there S.o.p("Combined: " + combined); List<String> keepers = new LinkedList<String>(); keepers.add("Ann"); keepers.add("Louise"); combined.retainAll(keepers); S.o.p ("Combined: " + combined); keepers.add("Bill"); keepers.add("Ed"); Combined: [Ann, Don, Ed, Fran] Combined: [Ann, Ed]
Getting Parts of Lists Choose first element of list (by position), and first element not on the list (by position) List<String> letters = new ArrayList<String>(); letters.add("b"); letters.add("i"); letters.add("y"); letters.add("o"); S.o.p("Letters: " + letters); S.o.p("Letters 1 to 3:" + letters.subList(1, 3)); letters.add("e"); Letters: [b, i, e, y, o] Letters 1 to 3: [i, e]
Sublists Select part of the list letters.subList(1, 3) [i, e] [b, i, e, y, o] b i e y o 0 1 2 3 4 5 Actually part of the larger list (not a copy) can be used to modify parts of the list letters.subList(1, 3).set(1, "x"); letters.subList(1, 3).clear(); [b, i, x, y, o] [b, y, o] 2 0 1 b i e x y o 0 1 2 3 4 5
Exercise Recall the race program from last time several heats, top 2 runners advance Add drugs testing: get a list of runners failing their drug tests disqualify them from advancing what method do we use?
the Collection interface List interface extends Collection interface Collection is an object that holds other objects Collections have base types (<String>, ...) Sets are another kind of Collection as are Queues Collection<E> List<E> Set<E> Queue<E> Collection, List, Set & Queue are interfaces; ArrayList & LinkedList are classes ArrayList<E> LinkedList<E>
Collection Methods Common to Lists, Sets and Queues add(T), remove(T), clear() contains, equals, isEmpty, size, toArray addAll, containsAll, removeAll, retainAll hashCode, iterator List and Deque add several methods different methods for each Set adds no more methods
Lists vs. Sets List elements allow duplicates; Sets do not list1 [a, b, c, a, b, d, a, a, a, a, b, z] set1 Client puts List elements in order; computer chooses order for Set elements list1.add("e"); [a, b, c, a, b, d, a, a, a, a, b, z, e] set1.add("e"); Set interface implemented by (e.g.) TreeSet [a, b, c, d, z] [a, b, c, d, e, z]
the Collections class the Collections (note the s) class has several static methods for working with Collection objects (like Lists and Sets) Collection is an interface implemented by class such as ArrayList Collections is a class has methods that are useful for Collection objects (such as an ArrayList)