Understanding List Operations in Python
A comprehensive guide to list operations in Python, covering topics such as defining lists, performing functions on lists, indexing, slicing, and more. Learn about the basics of lists, initializing lists, updating elements within a list, deleting list elements, and common list operations like concatenation and repetition.
Download Presentation
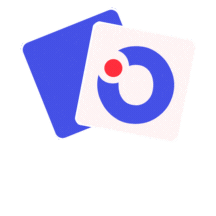
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Good morning (or good afternoon) Cat powered up https://forms.gle/zunuX4mP N1h43yud8
Open session with the class Questions for discussion
Abstraction Not the art, but to reduce to minimum details What is a list A list may comprise of numbers, strings, etc You may manually define a list using square brackets e.g. L = [a,b,c,d] Functions may be directly performed on a list using L.function_name() What are the die-die must know things? List operations forward/reverse sorting, positional indexing, appending, deleting, slicing, subsetting, etc For accessing elements via positional indexing, we may do so via the expression L[start:end] Note: Leave <end> blank if we want it to go all the way to the end of the list Note: the final index is not returned
A list is simply an ordered arrangement of elements (items) This Photo by Unknown Author is licensed under CC BY-SA
written as a list of comma- separated values (items) between square brackets. Initializing a list in python Items in a list need not be of the same type. list1 = ['physics', 'chemistry', 1997, 2000]; list2 = [1, 2, 3, 4, 5 ]; list3 = ["a", "b", "c", "d"]
List list1 = ['physics', 'chemistry', 1997, 2000]; Print print "Value available at index 2 : " Print print list1[2] Updating elements in list List list1[2] = 2001; Print print "New value available at index 2 : " Print print list1[2]
list1 = ['physics', 'chemistry', 1997, 2000]; print list1 Delete List Elements del list1[2]; print "After deleting value at index 2 : " print list1
Python Expression Results Description + and * operators mean concatenation and repetition len([1, 2, 3]) 3 Length [1, 2, 3] + [4, 5, 6] [1, 2, 3, 4, 5, 6] Concatenation Basic List Operations ['Hi!'] * 4 ['Hi!', 'Hi!', 'Hi!', 'Hi!'] Repetition 3 in [1, 2, 3] True Membership for x in [1, 2, 3]: print x, 1 2 3 Iteration
PYTHON EXPRESSION RESULTS DESCRIPTION L = ['spam', 'Spam', 'SPAM!'] L[2] SPAM! Offsets start at zero Indexing and Slicing L[-2] Spam Negative: count from the right L[1:] ['Spam', 'SPAM!'] Slicing fetches sections
Sr.No. Function with Description 1 cmp(list1, list2) #Compares elements of both lists. Python 2 only List functions 2 len(list) #Gives the total length of the list. list1 = ['spam', 'Spam', 'SPAM! ] list2 = [ spam , spanner , spammer ] list = [100,10, 1000,0] 3 max(list) #Returns item from the list with max value. 4 min(list) #Returns item from the list with min value. 5 list(seq) #Converts a tuple into list.
1 list.append(obj) #Appends object obj to list 2 list.count(obj) #Returns count of how many times obj occurs in list List methods 3 list.extend(seq) #Appends the contents of seq to list list = ['spam', 'Spam', 'SPAM! ] obj = spam seq = [ spammer , spanner , spin ] 4 list.index(obj) #Returns the lowest index in list that obj appears 5 list.insert(index, obj) #Inserts object obj into list at offset index
6 list.pop(obj=list[-1])Removes returns last object or obj from list and List methods 7 list.remove(obj)Removes object obj from list 8 list.reverse()Reverses objects of list in place 9 list.sort([func])Sorts objects of list, use compare func if given
Ready? Now let s start the tutorial proper
What do we know? A list is a ordered collection of elements Because it is ordered, position matters 1. Define the list 1. Define the list L = [34, 2, 45, 10, L = [34, 2, 45, 10, - -2, 6]. Then, do 2, 6]. Then, do the following the following actions: actions: We may therefore access elements in a collection by its positional index Functions may be directly performed on a list using L.function_name() Where function_name includes sort, reverse, append For accessing elements via positional indexing, we may do so via the expression L[start:end] You may retrieve the position index of an element by exact search using the L.index(<element name>) function Like a loan shark, once I know your address (position index), I can do something to you.
1. Define the list L = [34, 2, 45, 10, -2, 6]. Then, do the following actions: Data abstraction composite types in Python (slide 23). (a) sort and print the list Solution: L.sort() print(L) (e) remove the element 10 and print the list Solution: del L[L.index(10)] print(L) (b) add element 13 at the end of the list and print the list Solution: L.append(13) print(L) (f) display the sub-list from the 2nd to the 3rd element included Solution: print(L[1:3]) (c) reverse the order of the element in the list and print the list Solution: L.reverse() print(L) (g) display the sub-list from the 3rd to the last element included Solution: print(L[2:]) (d) display the index of the element 34 Solution: my_index = L.index(34) print(my_index)
2. What is printed on the screen when you execute the following commands? Data Abstraction - Strings in Python.pdf (slide 13) Sa = 'abcdefghij' Sb = Sa[:4]*2 + Sa[6:]*2 + Sa[5] What do we know? In Python, a string may also be treated as a collection of elements. In this case, the string is a collection of characters We may perform manipulation on a string via position indexing and even arithmetic operations Addition in string manipulation terms means to append (e.g. abc + efg = abcefg ) Multiplication in string manipulation terms means to duplicate (e.g. abc * 2 = abcabc )
2. What is printed on the screen when you execute the following commands? Data Abstraction - Strings in Python.pdf (slide 13) Sa[:4] is abcd Sa[:4]*2 is abcdabcd Sa[6:] is ghij Sa[6:]*2 is ghijghij Sa[5] is f Sa = 'abcdefghij' Sb = Sa[:4]*2 + Sa[6:]*2 + Sa[5] Solution: Sa[:4]*2 + Sa[6:]*2 + Sa[5] is abcdabcdghijghijf
2. What is printed on the screen when you execute the following commands? Data Abstraction - Strings in Python.pdf (slide 13) Sa[:4] is abcd Sa[:4]*2 is abcdabcd Sa[6:] is ghij Sa[6:]*2 is ghijghij Sa[5] is f Sa = 'abcdefghij' Sb = Sa[:4]*2 + Sa[6:]*2 + Sa[5] Solution: Sa[:4]*2 + Sa[6:]*2 + Sa[5] is abcdabcdghijghijf
As biologists, you will realize that string manipulation is very important since gene function is determined by the sequence of ATGCs. Additional Unfortunately, not all the functions that work on a list necessarily works on a string. For example, you cannot direct reverse a string using string.reverse(). Instead, we have to rely on creative use of indexing. The expression below takes the first to 3rd positions of Sa, and then reverses it using the call [::-1] Sa[:4][::-1]
What do we know? The range function can be used to specify a start, end, and interval in the form range(start, end, interval) It is important to note that the end is not included in the return The interval can be position or negative. If positive, end > start, and it is an incremental series If negative, start > end, and it is a decremental series 3. Using the 3. Using the range range function(s), function(s), create the create the following lists: following lists:
3. Using the 3. Using the range range function(s), function(s), create the create the following lists: following lists: Solution: (a) [3, 4, 5, 6] list(range(3,7))
Solution: 3. Using the 3. Using the range range function(s), function(s), create the create the following lists: following lists: (b) [18, 16, 14, 12] Solution: list(range(18,10,-2)) (c) [50, 45, 40, 35, 30, 5, 10, 15, 20, 25, 30]
3. Using the 3. Using the range range function(s), function(s), create the create the following lists: following lists: Solution: (c) [50, 45, 40, 35, 30, 5, 10, 15, 20, 25, 30] Solution: list(range(50,25,-5)) + list(range(5,35,5))
4. Assume you are given two lists, myList1 and myList2, both of unknown sizes (for testing purposes, you can create your own). Do the following: (a) Using only methods from lists, remove the last element from myList1, remove the first element from myList2, concatenate these two new lists while adding a string element 'Hello' in between. (Composite types slide 23)
4. Assume you are given two lists, myList1 and myList2, both of unknown sizes (for testing purposes, you can create your own). Do the following: (a) Using only methods from lists, remove the last element from myList1, remove the first element from myList2, concatenate these two new lists while adding a string element 'Hello' in between. (Composite types slide 23) Solution: myList1.pop() myList2.pop(0) myList1.append('Hello') myList1.extend(myList2) (b) Same question, without using any list method. Indexing only!
4. Assume you are given two lists, myList1 and myList2, both of unknown sizes (for testing purposes, you can create your own). Do the following: (a) Using only methods from lists, remove the last element from myList1, remove the first element from myList2, concatenate these two new lists while adding a string element 'Hello' in between. (Composite types slide 23) (b) Same question, without using any list method. Indexing only! Solution: myList1[:len(myList1)-1] + ['Hello'] + myList2[1:]