Understanding Scope and Control Links in C Programming
This content delves into the intricacies of scoping rules, static vs. dynamic scope, activation records, access, and control links in C programming. It covers topics such as global and local variables, parameter passing styles, control flow, and the behavior of different variables within blocks. The slides offer a detailed explanation with examples to help learners grasp these fundamental concepts effectively.
Download Presentation
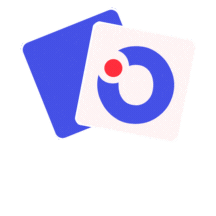
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
CS314 Section 5 Recitation 8 Long Zhao (lz311@rutgers.edu) Access and Control Links Parameter Passing Style Slides available at http://www.ilab.rutgers.edu/~lz311/CS314
Scoping Rules Global and local variables x, y are local to outer block z is local to inner bock x, y are global to inner block { int x=0; int y=x+1; { int z=(x+y)*(x-y); }; }; Static scope Global refers to declaration in closest enclosing block Dynamic scope Global refers to most recent activation record slide 2
Static vs. Dynamic Scope Example var x=1; function g(z) { return x+z; } function f(y) { var x = y+1; return g(y*x); } f(3); x 1 outer block y 3 f(3) x 4 z 12 g(12) Which x is used for expression x+z ? static scope dynamic scope slide 3
Activation Record For Static Scope Control link Link to activation record of previous (calling) block Depends on dynamic behavior of the program Access link Link to activation record of closest lexically enclosing block in program text Depends on the static program text Control link Access link Return address Return result addr Parameters Local variables Intermediate results Environment pointer Is this needed in C? (why?) slide 4
Static Scope with Access Links var x=1; function g(z) { return x+z; } function f(y) { var x = y+1; return g(y*x); } f(3); outer block x 1 control link access link g control link access link f
Dynamic Scope with Control Links var x=1; function g(z) { return x+z; } function f(y) { var x = y+1; return g(y*x); } f(3); x outer block x 1 f(3) control link outer block access link y 3 1 x 4 control link access link g(12) g control link access link control link access link z 12 f slide 6
Access & Control Links (Exercise) 1. 2. proc Main() { proc A() { call B() } proc B() { proc C() { proc D() { ... } call D() } call C() } call A() } int x; Access and control links of calling main()? int main() { x = 14; f(); g(); } void f() { int x = 13; h(); } void g() { int x = 12; h(); } void h() { printf("%d\n",x); }
Access & Control Links (Exercise) outer block 1. int x; x int main() { x = 14; f(); g(); } control link access link main control link access link void f() { int x = 13; h(); } f control link access link void g() { int x = 12; h(); } g control link access link void h() { printf("%d\n", x); } h
Access & Control Links (Exercise) outer block 1. x main() x = 14 // x = 14 (global) f() int x = 13 // x = 14 (global) h() printf // x = 14 (global) g() int x = 12 // x = 14 (global) h() printf // x = 14 (global) int x; main int main() { x = 14; f(); g(); } control link access link f() control link access link h() void f() { int x = 13; h(); } control link access link g() void g() { int x = 12; h(); } control link access link h() void h() { printf("%d\n", x); } control link access link
Access & Control Links (Exercise) outer block 1. proc Main() { proc A() { call B() } proc B() { proc C() { proc D() { ... } call D() } call C() } call A() } Main control link access link A control link access link B control link access link C control link access link D
Access & Control Links (Exercise) outer block 1. proc Main() { proc A() { call B() } proc B() { proc C() { proc D() { ... } call D() } call C() } call A() } Main() call A() call B() call C() call D() Main A() control link access link B() control link access link C() control link access link D() control link access link
Parameter Passing By value By reference By value-result By result By name slide 12
Pass by Value Caller passes r-value of the argument to function Compute the value of the argument at the time of the call and assign that value to the parameter Reduces aliasing Aliasing: two names refer to the same memory location Function cannot change value of caller s variable All arguments in C and Java (basic type) are passed by value To allow caller s variables to be modified, pointers can be passed as arguments in C Example: void swap(int *a, int *b) { } slide 13
Pass by Reference int h, i; void B(int* w) { int j, k; i = 2*(*w); *w = *w+1; } void A(int* x, int* y) { bool i, j; B(&h); } int main() { int a, b; h = 5; a = 3; b = 2; A(&a, &b); } Caller passes l-value of the argument to function Compute the address of the argument and assign that address to the parameter Increases aliasing (why?) Function can modify caller s variable via the address it received as argument slide 14
Two Ways To Pass By Reference C or C++ C++ only void swap (int *a, int *b) { int temp = *a; *a = *b; *b = temp; } void swap (int& a, int& b) { int temp = a; a = b; b = temp; } int x=3, y=4; swap(&x, &y); int x=3, y=4; swap(x, y); Which one is better? Why? slide 15
Pass by Value-Result Pass by value at the time of the call and/or copy the result back to the argument at the end of the call (copy-in-copy-out) Reference and value-result are the same, except when aliasing occurs Same variable is passed for two different parameters slide 16
Pass by Result The formal parameter acts as an uninitialized local variable within the called procedure. The parameter is then assigned a value during execution. This value is then passed back and assigned to the actual parameter.
Exercise What is output when function Print is called? (pass by value or reference) 3 0 2 2 0 2
Exercise (Pass by Result) c : array[1 10] of integer; m, n: integer; procedure r(i, j : integer) begin i := 0; j := 0; i := i + 1; j := j + 2; end r; m := 2; n := 3; r(m , n); write m, n; // 1, 2
Exercise (Pass by Value Result) int x = 1; // global x Void func1(int a) { print(a); print(x); a = 3; print(a); print(x); x = 4; print(a); print(x); } // 1 // 1 // 3 // 1 // 3 // 4 Void main() { print(x); func1(x); print(x); } // 1 // 3