Introduction to Basic Python Programming Concepts
In this Fall 2017 course, COSC 1306 - Computer Science and Programming by Jehan-François Périr, students delve into the fundamental concepts of Python programming. The course covers values, data types, strings, and identifying malformed strings in Python code.
Download Presentation
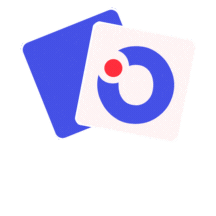
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
COSC 1306 COMPUTER SCIENCE AND PROGRAMMING Jehan-Fran ois P ris jfparis@uh.edu Fall 2017
THE ONLINE BOOK CHAPTER II BASIC PYTHON
Values Python programs manipulate values "Hello world!" "2+3" 5 Values have types
Value types (I) >>> type ("Hello world!") <class 'str'> >>> type ("5 + 3") Strings, integer and floating point are basic Python types <class 'str'> >>> type(24) <class 'int'> >>> type(3.1416) <class 'float'>
Value types (II) >>> type("3.1416") <class 'str'> >>> type('3.1416') <class 'str'> >>> Anything between matching quotes is a string
Strings Delimited by matching single quotes (' ') Can contain double quotes (") 'The subject was "touchy", she said' Delimited by matching double quotes (" ") Can contain signgle quotes (') "Let's go!" Delimited by three matching double quotes (""" """) Can contain almost anything
Find the malformed strings "Hello!" 'I'm busy!' "I like sugar cones.' "He asked for a "petit noir"." """I don't want a "petit noir".""" 'Cookies and cream'
Find the malformed strings "Hello!" 'I'm busy!' single quote inside ' ' "I like sugar cones.' mismatched quotes "He asked for a "petit noir"." """I don't want a "petit noir".""" 'Cookies and cream'
Integers 0, 1 ,2, 3, and-1, -2, -3 , No separating quotes It is 13000 and not 13,000 Can handle really huge large numbers Computations are always exact Nice feature of Python 3
Floating-point numbers 0.1, 3.1416, -5.75, But also .1, .25, .001 You could also meet later 1E3 (for 1 103), 1E-3 (for 0.001), Limited precision Fine for most applications
Find the malformed numbers 55,000 0.375 -32 3E3 -.5 3 000
Find the malformed numbers 55,000 0.375 -32 3E3 -.5 3 000
Numbers and strings '9' and 9 are two different values '9' means the digit "9" 9 means the number 9 They are not equal You cannot use one instead of the other
Converting numbers into strings >>> str(9) '9' >>> str(3.1416) '3.1416'
Converting strings into int >>> int("35") 35 >>>int("six") Does not work >>> int("3.1416") Does not work
Converting strings into float >>> float("3.1416") 3.1416
Converting numbers >>> float(3) 3.0 >>> int(3.1416) 3 >>> int(2.90) 2 >>> int(-2.90) -2 Truncates Does not round up
Variables Names that refer to a value pi 3.1416
Assigning a value name = 'Alice' Value assigned to variable can change result = 0 result = 3.1415927 result = 'undefined'
This is Python, not algebra (I) In Python, Ruby, C, C++, Java, Javascript, The = sign does not mean equality It means an assignment variable value When you see shirt_price = 24.99 think of shirt_price 24.99
This is Python, not algebra (II) Why? Using an equal sign for assignments started with FORTRAN in mid fifties Equal sign remains easier to type than left arrow The symbol for equality is the double equal sign a == b
Variable names Can consist of multiple characters: Must be letters, digits and underscore ( _ ) Cannot start with a digit Case sensitive Total, total and TOTAL are three different variable names
Prohibited variable names Some possible variable names are already used by Python Keywords Here is the full list and as assert def del elif finally for from in is lambda pass raise return yield True False break else global nonlocal not try None class except if continue exec import or with while
Variables have types The type of a variable is the type of the value it refers to >>> a = "hello!" >>> type(a) <class 'str'> >>> a = 5 >>> type(a) <class 'int'> >>> a = 3.5 >>> type(a) <class 'float'> It can change over time
Find the wrong variable names aleph Fall16 easy_money bigBrother 2step starts with a digit Texas2step raise is a reserved word
Find the wrong variable name aleph Fall16 easy_money bigBrother 2step Texas2step raise
Picking good variable names Using mnemonic names helps making programs more readable by humans instructor_name, student__name Instead of nm1, nm2 Keep in mind that Python does not understand their meaning
Statements and expressions (I) A statement specifies an action that the Python interpreter can execute print("Hello world!") count = 0 Typically occupies a line Basic element of a program
Statements and expressions (II) An expression is Python's equivalent of an algebraic expression Three differences They are always evaluated They use somewhat different conventions ?2 + 2?? + ?2becomes a**2 + 2*a*b + b**2 They are more general
Statements or expressions? >>> name = "Mike" >>> print(name) Mike >>> len(name) 4 >>> nameLength = len(name) >>> 10 + 3 13 >>> total = 10 + 3
Statements or expressions? >>> name = "Mike"Statement >>> print(name) Mike >>> len(name) 4 >>> name_length = len(name)Statement >>> 10 + 3 13 >>> total = 10 + 3Statement Statement Expression Expression
Operators and Operands Most of the operators are the same as those you encountered in HS Algebra but Multiplication sign is * Multiplication is never explicit Must distinguish between max and m*a*x Exponentiation is ** Operators obey the same precedence rules as in HS algebra
Examples >>> 5 - 2.5 2.5 >>> 1*2*3 6 >>> 25/2 12.5 >>> 16/2 8.0 In Python the division operator always returns a float
Integer division (//) >>> 5//4 1 >>> 8//4 2 >>> 6.0//4 1.0 >>> 3.5//2 1.0 (and not 1.25) (and not 2.0) (and not 1.5) (and not 1.75)
The modulus operator (%) a%b returns the remainder of the integer division of a by b >>> 9//4 2 >>> 9 - (9//4)*4 1 >>> 9%4 1
The modulus operator (%) Also work for floating-point numbers >>> 4.6//2 2.0 >>> 4.6%2 0.5999999999999996 Floating-point computation was not accurate but fairly close
Our friend the input() function (I) Specific to Python xyz = input("Blablabla: ") Will print Blablabla:_ Will store in xyzthe characters you will enter
Our friend the input() function (II) The value returned by input will always be a string price = enter("Enter a price: ") If you want an int or a float you must convert the string price = float(enter("Enter a price: ")) Note the two closing parentheses
Wishing a happy birthday (I) We want to print a personalized birthday wish Happy birthday Michael User will enter the name of the person Program will do the rest Will also ask the program to prompt the user for a name Enter a name:
Wishing a happy birthday (II) name = input("Enter a name:") print("Happy birthday", name) When you specify several items as the arguments of a print function, print separates them with spaces >>> print ("Hello", "world!") Hello world!
The concatenation operator The same + as for addition "U" + "H"returns"UH" "How" + "are" + you" + "?"returns "Howareyou?" No spaces are added! "How_" + "are_" + you_" + "?"returns "How are you?" It works only with strings!
Order of operations Consider a*b**2 +c*d/4 e/f In which order are the operations performed? In the same order as in algebra First exponentiationsright to left Then multiplications and divisions left to right Finally additions and subtractions left to right Parentheses override these priorities
Precedence rules ** *, / and // + and -
With or without parentheses? Which expressions are equivalent? a + (b c) a + b c (a*b)**2 a*b**2 (a + b)/2 a + b/2 a/(b*c) a/b*c (a*b)/c a*b/c (a**b)**c a**b**c a**(b**c) a**(b**c)
The answers Which expressions are equivalent? a + (b c) a + b c (a*b)**2 a*b**2 = a*(b**2) (a + b)/2 a + b/2 = a + (b/2) a/(b*c) a/b*c = (a/b)*c (a*b)/c a*b/c (a**b)**c a**b**c = a**(b**c) a**(b**c) a**b**c
My take Know the rules For the quiz For deciphering complex expressions Use wisely parentheses in your code When it is needed When it clarifies dubious cases (a*b)/c a**(b**c)
Remember Nobody ever failed a test because he or she used a few extra parentheses The reverse is not true
Reassignment Including variables At the beginning of the season shirt_price = 24.99 Then discounted shirt_price = 12.99 Finally red-tagged shirt_price = 7.99
Updating a variable (I) Coffeehouse tab Open tab: tab = 0 Add a latte: tab = tab + 4.95 Add sales tax tab = tab + 4.95*0.0825
A short cut Rather than typing tab = tab + 4.95 you can type tab += 4.95 It works for +, -, * and / price /= 2