Understanding Loops in Python Programming
The concept of loops in Python programming is explained in detail, covering for and while loops, the range function, break, continue, and pass statements, and examples to demonstrate their usage. Learn how to iterate over sequences, handle loop control statements, and efficiently execute repetitive tasks using loops in Python.
Download Presentation
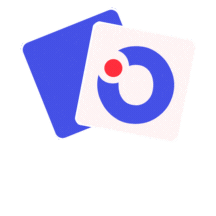
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Loops in python programming For while
1. For loop 2. Range function 3. Break, continue, and Pass statement 4. Booleans 5. While loop 6. Nested loop Outline
For Loop The for loop in python iterates over the items of any sequence be it a list or a string. Moreover, using for loop in the program will minimize the line of code. Points to keep in mind while using for loop in python: It should always start with for keyword and should end with a colon(:). The statements inside the loop should be indented(four spacebars). Syntax: For iterator_variable in sequence: statement(s)
Example This is actually a temporary variable which store the number of iteration Code: for i in range(6): print( Hello World! ) Number of times you want to execute the statement Output: print( Hello World! ) print( Hello World! ) print( Hello World! ) print( Hello World! ) print( Hello World! )
Range function To iterate over a sequence of numbers, the built-in function range() comes in handy. It generates arithmetic progressions. For example, if we want to print a whole number from 0 to 10, it can be done using a range(11) function in a for loop. In a range() function we can also define an optional start and the endpoint to the sequence. The start is always included while the endpoint is always excluded. Starting point Example: Ending point for i in range(1, 6): print("Hello World!") Output: Prints Hello World! five times
cont. Range function The range() function also takes in another optional parameter i.e. step size. Passing step size in a range() function is important when you want to print the value uniformly with a certain interval. Step size is always passed as a third parameter where the first parameter is the starting point and the second parameter is the ending point. And if the step_size is not provided it will take 1 as a default step_size. Step size Example: for i in range(0,20, 2): print(i) Output: prints all the even numbers till 20
1 Sum of numbers -Write a program to find the sum of all the numbers less than a number entered by the user. Display the sum. Expected Output:
Break, Continue, & Pass Statement (Loop control statement) A break statement is used to immediately terminate a loop. A continue statement is used to skip out future commands inside a loop and return to the top of the loop. A Pass is a null operation when it is executed, nothing happens. It is useful as a placeholder when a statement is required syntactically when no code needs to be executed. These statements can be used with for or while loops.
Break, Continue, & Pass Statement (Loop control statement) Example: Break Continue Pass for i in range(10): if i == 3: break print(i) for i in range(5): if i == 3: continue print(i) Output: 0 1 2 4 for i in range(10): Pass Output: 0 1 2 Output: Nothing will be printed
2 Magic Number Write a program that makes the user guess a particular number between 1 and 100. Save the number to be guessed in a variable called magic_number. If the user guesses a number higher than the secret number, you should say Too high!. Similarly, you should say Too low! if they guess a number lower than the secret number. Once they guess the number, say Correct! Expected Output:
Boolean A boolean is a value that can be either True or False. Example: brought_food = True brought_drink = False - - Boolean variables don't have quotation marks. The values must start with capital letters.
While Loop while loop repeatedly carries out a target statement while the condition is true. The loop iterates as long as the defined condition is True. When the condition becomes false, program control passes to the line immediately following the loop. Example: while True: print("I am a programmer") break while False: print("Not printed because while the condition is already False") break Output: I am a programmer
While Loop Example: x = 1 while x > 0: print ("Hello!") x = x - 1 print ("I like Python.") Output: Hello! I like Python.
3 Guess the number Write a program where the user enters a number. If the number matches the number, acknowledge the user and stop the program. If the number does not match, keep asking for the next number. Expected Output:
Nested Loop Loop within a loop is called a nested loop. For loop within for loop is called a nested for loop. While loop within while loop is called as nested while loop. Example: Nested for loop Example: Nested while loop for i in range(4): for j in range(3): print ("Hello!") i = 1 j = 5 while i < 4: while j < 8: print(i,",", j) j = j + 1 j i = i + 1
Nested Loop(Example) Output: 5 4 3 2 5 4 3 2 5 4 3 2 5 4 3 2 for i in range(4): x = 5 while x > 1: print (x) x = x - 1
4 Rolling a dice Write a program that will print out all combinations that can be made when 2 dice are rolled. And should continue until all values up to 6, and 6 are printed. (Hint: You should have a space after each comma!) Expected Output:
1 Multiplication Table: Write a program to print a multiplication table to 12 of a given number. Expected Output:
2 Printing pattern: Write a program to print the following two patterns implementing the nested loop concept.