Exploring Text Manipulation in MATLAB
Learn how to work with strings, characters, and concatenate text in MATLAB. Discover how to convert between characters and ASCII codes, select and modify string elements, and create matrices of strings. Dive into essential string operations and make the most out of text manipulation capabilities in MATLAB.
Download Presentation
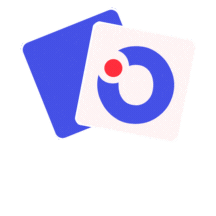
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Characters and Text You can assign text strings to MATLAB variables using single quotes. For example, c = 'Hello'; The result is not a numeric matrix or array but a 1-by-5 character array. Internally, the characters are stored as numbers The statement a = double(c) converts the character array to a numeric matrix containing floating-point representations of the ASCII codes for each character. The result is a = 72 101 108 108 111 The statement c = char(a) reverses the conversion.
Characters and Text Concatenation with square brackets joins text variables together into larger strings. The statement h = [c, ' world'] joins the strings horizontally The statement v = [c; 'world'] joins them vertically this vertical concatenate can only work if the number of characters is the same in each string. help char or help double for more information
Working with strings Strings in Matlab are vectors of characters Always use single quotes to define strings >> name = 'Jonas' name = Jonas >> name(1) ans = J >> name(1:3) ans = Jon
Working with strings >> x = 'abc' x = abc >> y = 'def' y = def >> x + y ans = 197 199 201 >> double('a') ans = 97 >> double('d') ans = 100 >> char(97) ans =a >> strcat(x,y) ans = abcdef >> newstring = strcat(x,y) newstring = abcdef
Working with strings Just as with arrays containing numbers, values in string arrays can be selected using round ( ) brackets. For example: >> a = Hello world! ; >> a(7:11) ans = 'world Or the values can be overwritten: >> a(7:end) = 'earth!' a = 'Hello earth!' Or the values can be removed: >> a(1:6) = [] a = 'earth!'
Working with strings It is possible to create a matrix of strings (of the same length) >> a = 'Hello world!' >> b = 'Hello Peter!' >> x = [a ; b] See what happens if the strings are of different lengths: >> c = 'Goodbye' >> x = [a; c] Just like with numeric matrices, matrix elements sizes must match. It is possible to get around this by padding strings with spaces: >> c = 'Goodbye ' >> x = [a; c] (Need to have exactly 5 spaces here after final 'e )
Working with strings Matlab has an inbuilt function to do this without you having to work out exactly how many spaces to pad each string with. The function is called strvcat and it automatically pads each string with spaces. In order to form a valid matrix: >> a = 'Hello' >> b = 'Goodbye' >> c = 'OK' >> x = strvcat(a,b,c) This is (almost) the same as: >> x = ['Hello '; 'Goodbye'; 'OK '] In strvcat each text input parameter can itself be a string matrix: >> y = strvcat(x,'Yes',x,'No')
Working with strings There are many functions that work on strings. For example: strfind(S1, S2) will search inside string S1 and return the starting indices of any occurrences of the search string S2 >> s = 'How much wood would a woodchuck chuck?'; >> strfind(s,'wood') ans =10 23 findstr(S1, S2) is similar to strfind(S1, S2), but initially it finds the shorter of S1 and S2, before returning the starting indices of it inside the longer string. This means the order of the arguments does not matter: >> findstr(s,'wood') ans = 10 23 >> findstr('wood',s) ans = 10 23
Working with strings >> a = 'Good day ; >> b = Hallo ; >> a ==b >> b = 'good day ; >> a ==b strcmp will compare two strings, case-sensitively and return logical 1 (true) if they are identical or logical 0 (false) otherwise: >> strcmp(a,b) ans = 0 strcmpi will compare two strings, ignoring case, and return true if they are identical or false otherwise: >> strcmpi(a,b) ans = 1
Working with strings strncmp(s1,s2,n) will compare the first n chars of two strings, case-sensitively and return true if they are identical or false otherwise: >> a = 'Good day ; >> b = 'good dog ; >> strncmp(a,b,4) ans = 0 strncmpi(s1,s2,n) will compare the first n chars of two strings ignoring case and true if they are identical or false otherwise: >> strncmpi(a,b,4) ans = 1
Formatting strings What do we typically do with strings? Printing out messages to the workspace Printing out data or messages to files Using them as stimuli in an experiment Using them as filenames, codes, or identifiers
Formatting strings Several ways to print a string out to the workspace: type the name of the variable without a trailing semicolon disp is almost the same as above, except it does not print out the variable name fprintf is for formatting text and printing out to a file or other device, such as the workspace sprintf is for formatting text in order to create new string variables
Formatting strings fprintf is a very powerful command for formatting strings, combining them, and printing them out >> help fprintf fprintf Write formatted data to text file. fprintf(FID, FORMAT, A, ...) applies the FORMAT to all elements of array A and any additional array arguments in column order, and writes the data to a text file. FID is an integer file identifier. Obtain FID from FOPEN, or set it to 1 (for standard output, the screen) or 2 (standard error). fprintf uses the encoding scheme specified in the call to FOPEN. fprintf(FORMAT, A, ...) formats data and displays the results on the screen.
Formatting strings >> employee = 'Fred'; >> age = 32; >> score = 88.432; >> fprintf('Employee: %s is %d years old and scored %f',employee,age,score); Employee: Fred is 32 years old and scored 88.432000>> These symbols that start with % are substitution points ( conversion characters ). Matlab will insert the subsequent variables into the text, in order. The number of variables listed must match the number of conversion characters. %s string %d integer/digit %i integer/digit %f floating point number %c single character
Formatting strings >> fprintf('%s\t%d\n',employee,age) Fred 32 There are many special characters to control formatting that begin with the backslash: \t tab \n newline \v vertical tab
Working with numbers in strings >> fprintf('Score: %f\n',score); Score: 88.432000 >> fprintf('Score: %.2f\n',score); Score: 88.43 >> fprintf('Score: %.0f\n',score); Score: 88 >> fprintf('Score: %.5f\n',score); Score: 88.43200 Specifies the number of decimal places in a floating point number >> fprintf('Age: %d\n',age) Age: 32 >> fprintf('Age: %.4d\n',age) Age: 0032 Or the number of total digits in an integer
Special characters >> fprintf ('Score was %.2f%%\n',score) Score was 88.43% >> fprintf('Name is ''%s''\n',employee) Name is 'Fred' If you want to print the actual character instead of invoking its special meaning: to print a single-quote %% to print a percent sign
Creating string variables >> help sprintf sprintf Write formatted data to string. STR = sprintf(FORMAT, A, ...) applies the FORMAT to all elements of array A and any additional array arguments in column order, and returns the results to string STR. [STR, ERRMSG] = sprintf(FORMAT, A, ...) returns an error message when the operation is unsuccessful. Otherwise, ERRMSG is empty. sprintf is the same as FPRINTF except that it returns the data in a MATLAB string rather than writing to a file.
Creating string variables >> subject = 'SXF32'; >> logfileName = sprintf('data_%s.txt',subject); >> logfileName logfileName = data_SXF32.txt Make your variable names as informative as possible. Someone reading your code should know what a variable contains by looking at its name. That person might be Future You or a colleague.
Creating string variables Try these: >>Name='Peter' >> Age=39 >> sprintf('My name is %s', Name) >> sprintf('I am %d years old', Age) >> sprintf('I am %d years old. Next year I will be %d', Age, Age+1) >> sprintf('My name is %s and my age is %d', Name, Age) >> tmp=sprintf('My name is %s and my age is %d', Name, Age); >> disp(tmp)
String formating Field and width and precision can be specified like this: >> sprintf('%f', pi) % Default formatting >> sprintf('%5.3f', pi) % The 3 here refers to the no of digits to show to the right of the decimal point and the 5 refers to the minimum no of digits to show overall (including the decimal point) Padding of the output with leading 0's can be done like this: >> sprintf('%8.3f', pi) >> sprintf('%08.3f', pi) % A single 0 is used here to signify to Matlab to use padding. Two or more 0's do not mean pad with 2 >> help num2str >> help str2num >> help double >> help char
Useful videos https://www.youtube.com/watch?v=V8kmahQ-kd4 (introduction to data types 20 min) https://www.youtube.com/watch?v=g7juXCBCgHM&feature=youtu.be (strings 29 min)