Understanding Loop Structures in Python Programming
This lecture covers Loop Structures, specifically focusing on the while statement and nested loops in Python programming. It discusses the Fibonacci sequence and demonstrates how to write a program to compute the nth Fibonacci number. Additionally, it explains the difference between definite and indefinite loops, highlighting the implementation of indefinite loops using the while statement.
Download Presentation
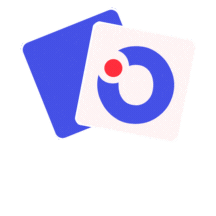
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
15-110: Principles of Computing Loop Structures- Part III Lecture 9, September 30, 2018 Mohammad Hammoud Carnegie Mellon University in Qatar
Today Last Session: Loop Structures- Part II Today s Session: Loop Structures- Part III: While Statement Nested Loops Various Examples Announcements: HA3 will be out by tonight; it is due on Saturday Oct 6 by 11:00AM Quiz I is on Sunday Oct 7
Recap: Fibonacci Sequence Suppose we want to write a program that computes and outputs the nth Fibonacci number, where n is a value entered by a user The Fibonacci sequence starts with 0 and 1 After these first two numbers, each number in the sequence is computed as simply the sum of the previous two numbers E.g., 0, 1, 1, 2, 3, 5, 8, 13, 21, 34,
Recap: Fibonacci Sequence def fibonacci(n): f_i = 0 f_j = 1 print(f_i, f_j, end = " ") for k in range(2, n+1): f_new = f_i + f_j print(f_new, end = " ") f_i = f_j f_j = f_new
Recap: Fibonacci Sequence n = eval(input("Enter a number that is larger than 1 >> ")) if n < 2: print("You can only enter a number that is larger than 1!") else: fibonacci(n)
Definite Loops vs. Indefinite Loops So far, we have considered only the case where the number of iterations is determined before the loop starts This kind of loops is called definite loops and for is used in Python to write definite loops But, what if we want to write loops, wherein we do not know the number of iterations beforehand? This kind of loops is denoted as indefinite loops
The While Statement In Python, an indefinite loop is implemented using a while statement while <condition>: <body> <condition> is a Boolean expression, just like in if statements <body> is, as usual, a sequence of one or more statements
The Flowchart of a While Loop No Is <condition> True? Yes <body>
Revisiting Average of a Series of Numbers Here is how we have done it before: def main(): n = eval(input("How many numbers do you have? ")) sum = 0.0 for i in range(n): x = eval(input("Enter a number >> ")) sum = sum + x print("\nThe average of the numbers is", sum/n) main()
Revisiting Average of a Series of Numbers Here is how we can do it now using a while statement: sum = 0.0 n = eval(input("How many numbers do you have? ")) count = 0 while count < n: x = eval(input("Enter a number >> ")) sum = sum + x count = count + 1 print("The average of the " + str(n) + " numbers you entered is ", sum/n)
Revisiting Average of a Series of Numbers Here is also another version that assumes no prior knowledge about the quantity of numbers the user will input sum = 0.0 count = 0 moreData = "yes" while moreData == "yes": x = eval(input("Enter a number >> ")) sum = sum + x count = count + 1 moreData = input("Do you have more numbers (yes or no)? ") print("The average of the " + str(count) + " numbers you entered is ", sum/count)
Printing Odd Numbers With Input Validation Suppose we want to print the odd numbers between two user-input numbers (inclusive), say, start and end The program assumes some conditions, whereby the start and end numbers shall be positive and end should be always greater than start Hence, we should continue prompting the user for the correct input before proceeding with printing the odd numbers This process is typically called input validation Well-engineered programs should validate inputs whenever possible!
Printing Odd Numbers With Input Validation 1. while True: 2. start = eval(input("Enter start number: ")) 3. end = eval(input("Enter end number: ")) 4. if start >=0 and end >= 0 and end > start: 5. break 6. else: 7. print("Please enter positive numbers, with end being greater than start") 8. 9. for i in range(start, end + 1): 10. if i % 2 == 0: 11. continue 12. print(i, end = " ") continues back at line 9. It breaks the loop; execution continues at line 8. It skips one iteration in the loop; execution
Nested Loops Like the if statement, loops can also be nested to produce sophisticated algorithms Example: Write a program that prints the following rhombus shape * * * * * * * * * * * * * * * *
The Rhombus Example One way (not necessarily the best way!) to think about this problem is to assume that the stars are within a matrix with equal rows and columns 1 2 3 4 5 6 7 8 9 1 * 2 * * Can you figure out the different relationships between rows and columns? 3 * * 4 * * 5 * * 6 * * 7 * * 8 * * 9 *
The Rhombus Example One way (not necessarily the best way!) to think about this problem is to assume that the stars are within a matrix with equal rows and columns 1 2 3 4 5 6 7 8 9 1 * 2 * * Print a star when: 1)Row + Column == 6 2)Row + Column == 14 3)Row Column == 4 4)Column Row == 4 3 * * 4 * * 5 * * 6 * * 7 * * 8 * * 9 *
The Rhombus Example Here is one way of writing the program in Python for i in range(1, 10): for j in range(1, 10): if ((i+j== 6) or (j-i==4) or (i+j == 14) or (i-j==4)): print("*", end = "") else: print(" ", end = "") print() Can you generalize this code?
The Rhombus Example What are 6, 14, 4, and 4 below? 1 2 3 4 5 6 7 8 9 1 * 2 * * Print a star when: 1)Row + Column == 6 2)Row + Column == 14 3)Row Column == 4 4)Column Row == 4 3 * * 4 * * 5 * * 6 * * 7 * * 8 * * 9 *
The Rhombus Example What are 6, 14, 4, and 4 below? 1 2 3 4 5 6 7 8 9 1 * 2 * * Print a star when: 1)Row + Column == 6 (i.e., Columns/2 +2) 2)Row + Column == 14 (i.e., Columns + ????/? ) 3)Row Column == 4 (i.e., Columns/2) 4)Column Row == 4 (i.e., Columns/2) 3 * * 4 * * 5 * * 6 * * 7 * * 8 * * 9 *
The Rhombus Example: A More General Version while True: rows = eval(input("Enter number of rows: ")) columns = eval(input("Enter number of columns: ")) if rows != columns or rows % 2 != 1 or columns % 2 != 1: print("Please enter odd and equal rows and columns") else: break rows = abs(rows) columns = abs(columns)
The Rhombus Example: A More General Version for i in range(1, rows+1): for j in range(1, columns+1): if ((i+j== (columns//2 +2)) or (j-i==(columns//2)) or (i+j == (columns+ math.ceil(rows/2))) or (i-j==(columns//2))): print("*", end = "") else: print(" ", end = "") print()
Next Lecture Problem Solving