Understanding Loops and Arrays in JavaScript
Programming with loops in JavaScript allows commands to repeat for a specified number of iterations, providing a way to efficiently perform repetitive tasks. This concept is crucial in mastering JavaScript programming and forms the backbone of many algorithms and applications. The article delves into the syntax of writing for loops in JavaScript, practical examples, different loop structures, and provides insights into effectively using loops in programming.
Download Presentation
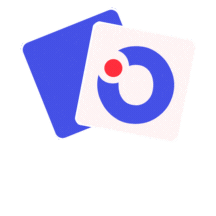
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Loops and Arrays in JavaScript MIS 2402 Department of MIS Fox School of Business Temple University
Agenda Syntax for writing for loops in JavaScript Some practical examples of loops 2
Whats a loop? In programming, a loop is a series of commands (called a block of code ) that repeats for a specified number of iterations. It s a programmatic way of doing the same thing over and over again. 3
Advisory JavaScript supports several different kinds of loop structures. for for/in for/of while do/while All those structures do similar things. In most situations, they are interchangeable! In this class, we will only use the first one: the for loop. For the new programmer, learning one general purpose loop structure and knowing it well, is better than trying to learn several different structures, each with a slightly different syntax! 4
The syntax of a JavaScript for loop for (counter initialization; condition; increment expression ) { statements } For example: Write the numbers 1 to 3 to the console. The loop continues while this expression is true. x is declared and assigned a value. A shorthand way of saying x = x + 1 The code block begins and ends. for (let x = 1; x <= 3; x++) { console.log(x); } 5
Lets see that with some animation The code The computer s memory console.log("Starting"); for (let x = 1; x <= 3; x++) { console.log(x); } x = 1 X 4 X 2 X 3 The console Starting 1 2 3 This code can be found in loopexamples.zip, loop01.html 6
Another (simple) example of a for loop This loop that adds the numbers from 1 through 3 let sumOfNumbers = 0; let numberOfLoops = 3; for ( let counter = 1; counter <= numberOfLoops; counter++ ) { sumOfNumbers = sumOfNumbers + counter; } console.log(sumOfNumbers); Thinking ahead! We establish sumOfNumbers here so we can use it later in the loop. We re using this variable to control the number of iterations. 7
Lets see that with some animation The code The computer s memory let sumOfNumbers = 0; let numberOfLoops = 3; for (let counter=1;counter <= numberOfLoops;counter++){ sumOfNumbers = sumOfNumbers + counter; } console.log(sumOfNumbers); sumOfNumbers = 0 numberOfLoops=3 counter=1 X 2 X 1 X 3 X 6 X 3 X 4 The console 6 This code can be found in loopexamples.zip, loop02.html 8
Another example of a for loop let investment = 10000; let annualRate = 7.0; let years = 10; let futureValue = investment; for ( let i = 1; i <= years; i++ ) { futureValue += futureValue * annualRate / 100; } console.log(futureValue.toFixed(2)); // displays 19671.51 Other ways that the future value calculation could be coded inside the code block //Either of these are good substitutes futureValue = futureValue + (futureValue * (annualRate / 100)); futureValue = futureValue * (1 + (annualRate / 100)) Let s see this loop at work! (see loop03.html in loopexamples.zip) 9
More examples Loops can be used to generate repetitive blocks of text. (see loop04.html in loopexamples.zip) Loops can be nested inside other loops (see loop05.html in loopexamples.zip) ALERT! In that last example we use the jQuery .append() method to add text to a message div tag. Appending text is different from replacing text! 10
So what are loops good for? Performing a calculation that requires iterations. -OR- Generating repetitive text. Sometimes that text is HTML. Tags like these can often be generated with a loop: <table> rows <select> options <ul> items <ol> items. NOTE! Loops are often used in combination with other loops, conditional statements, and functions! 11