PHP File Handling Functions Overview
The PHP file handling functions discussed in the content include mkdir, rmdir, unlink, reading contents from remote files, file_get_contents, rename, strtotime, and gmdate. Each function serves a specific purpose in file manipulation, such as creating directories, removing files, reading contents from URLs, and manipulating file names. The examples provided illustrate how to use these functions effectively in PHP programming.
Download Presentation
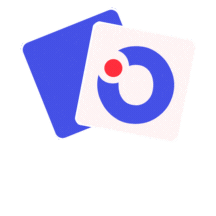
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
MKDIR AND RMDIR FUNCTION. <?php echo "<br>" . "OUTPUT:" . "<br>"; mkdir('gfg'); $dirname = "gfg"; if(rmdir($dirname)) { echo ("$dirname successfully removed"); } else { echo ($dirname . "couldn't be removed"); } ?> OUTPUT: gfg successfully removed.
UNLINK FUNCTION. <?php echo "<br>" . "OUTPUT:" . "<br>"; $file = "test1.txt"; if (!unlink($file)) { echo ("Error deleting $file"); } else { echo ("Deleted $file"); } ?> OUTPUT: Deleted test1.txt
READ CONTENTS FROM REMOTE FILE <?php echo "<br>" . "OUTPUT:" . "<br>"; $data=file("http:www.google.com"); foreach($data as $i) { echo $i; } ?> OUTPUT: ASHU
FILE_GET_CONTENTS FUNCTION. OBJECTIVE: To read contents from a file. PROGRAM: DESCRIPTION: The file_get_contents() function in PHP is an inbuilt function which is used to read a file into a string. The function uses memory mapping techniques which are supported by the server and thus enhances the performances making it a preferred way of reading contents of a file <?php echo "<br>" . "OUTPUT:" . "<br>"; $data=file_get_contents("ab.txt"); echo $data; Syntax: ?> file_get_contents($path, $include_path, $context, OUTPUT: SM $start, $max_length)
RENAME FUNCTION. RENAME FUNCTION. OBJECTIVE: To illustrate use of rename function. DESCRIPTION: The rename() function in PHP is an inbuilt function which is used to rename a file or directory. It makes an attempt to change an old name of a file or directory with a new name specified by the user and it may move between directories if necessary\ PROGRAM: <?php echo "<br>" . "OUTPUT:" . "<br>"; rename("pic.txt","rib.txt"); echo "Renamed successfully"; ?> OUTPUT: Renamed successfully.
STRTOTIME() function <?php $timestamp = strtotime( "February 15, 2019" ); echo "<br>" . "OUTPUT:" . "<br>"; print date( 'Y-m-d', $timestamp ); ?> OUTPUT: 2019-02-15
GMDATE() FUNCTION GMDATE() FUNCTION <?php echo "<br>" . "OUTPUT:" . "<br>"; echo date("M d Y H:i:s", mktime(0, 0, 0, 1, 1, 1998)) . "<br>"; echo gmdate("M d Y H:i:s", mktime(0, 0, 0, 1, 1, 1998)); ?> OUTPUT: Jan 01 1998 00:00:00 Dec 31 1997 23:00:00
SCANDIR() FUNCTION. OBJECTIVE: To illustrate use of scandir function. $dir = "C: xampp\htdocs\mywork"; $a = scandir($dir); DESCRIPTION: The scandir() function returns an array of files and directories of the specified directory. $b = scandir($dir,1); print_r($a); SYNTAX: scandir(directory,sorting_order,context); print_r($b); ?> PROGRAM: <?php echo "<br>" . "OUTPUT:" . "<br>";
COPY()FUNCTION OBJECTIVE: To copy contents of one file to another. echo "Contents Copied"; } DESCRIPTION: The copy() function copies a file. else This function returns TRUE on success and FALSE on failure. { echo "Contents not copied"; SYNTAX: copy(file,to_file) } PROGRAM: ?> <?php OUTPUT: Contents Copied. if(copy("ab.txt","a.txt")) {
FILE_PUT_CONTENTS() FUNCTION OBJECTIVE: To write contents in a file. <?php DESCRIPTION:The file_put_contents() writes a string to a file. echo "<br>" . "OUTPUT:" . "<br>"; $data="A fish in a Pond"; -This function follows these rules when accessing a file: if(file_put_contents("ab.txt",$data)) { -If FILE_USE_INCLUDE_PATH is set, check the include path for a copy of *filename* echo "Contents Written in file"; -Create the file if it does not exist } -Open the file else -Lock the file if LOCK_EX is set { -If FILE_APPEND is set, move to the end of the file. Otherwise, clear the file content echo "Contents not written in file"; } -Write the data into the file ?> -Close the file and release any locks OUTPUT: Contents Written in file. -This function returns the number of character written into the file on success, or FALSE on failure.