Overview of C++ Strings and Predefined Functions
This content provides an in-depth look at C++ strings, comparing standard string handling to C-style strings. It covers topics such as declaring and initializing strings, predefined functions in and , and examples of functions like strcpy, strlen, toupper, and isalnum for string manipulation in C++. Helpful insights for understanding string operations in C++ programming.
Download Presentation
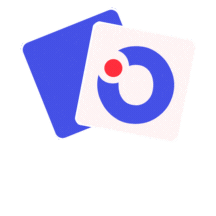
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
CSC 270 Survey of Programming Languages C++ Lecture 2 Strings Credited to Dr. Robert Siegfried
Goals Standard string vs cstring cstring functions std::string member methods Functions that use std::string cin / cout / character manipulation getline vs cin
Standard String and C Style String http://www.tutorialspoint.com/cplusplus/cpp_strings.htm Declare and initialize: char cstring[100]; char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'}; char a[] = "text"; #include <cstring> #include <cctype>
Predefined Functions in <cstring> Function strcpy(s, t) strncpy(s, t, n) Description Copies t into s Copies t into s but no more than n characters are copies Concatenates t to the end of s Concatenates t to the end of s but no more than n characters Returns the length of s (not counting \0 ) Returns 0 if s == t < 0 if s < t > 0 if s > t Same as strcmp but compares no more than n characters Caution No bounds checking Not implemented in all versions of c++ No bounds checking Not implemented in all versions of c++ strcat(s, t) strncat(s, t, n) strlen(s) strcmp(s, t) No bounds checking strncmp(s, t, n) Not implemented in all versions of c++
Functions in <cctype> Function toupper(c) Description Returns the upper case version of the character Returns the lower case version of the character Returns true if c is an upper case letter Returns true if c is an lower case letter Returns true if c is a letter Example c = toupper( a ); tolower(c) c = tolower( A ); isupper(c) if (isupper(c)) cout << upper case ; islower(c) if (islower(c)) cout << lower case ; isalpha(c) if (isalpha(c)) cout << it s a letter ; isdigit(c) if (isalpha(c)) cout << it s a number ; Returns true if c is a digit (0 through 9)
Functions in <cctype> (continued) Function isalnum(c) Description Returns true if c is alphanumeric Returns true if c is a white space character Returns true if c is a printable character other than number, letter or white space Returns true if c is a printable character Returns true if c is a printable character other an white space Example if (isalnum( 3 )) cout << alphanumeric ; isspace(c) while (isspace(c)) cin.get(c); ispunct(c) if (ispunct(c)) cout << punctuation ; isprint(c) isgraph(c) isctrl(c) Returns true if c is a control character
Standard String std::string include <string> or using namespace std http://en.cppreference.com/w/cpp/header = assigns and + concatenates and == tests 2 ways to create string str = "a string" string str("a string") str.length returns length as a property str[#] lets you get straight to character index as though it were an array (no protection on out of bounds) str.at(#) returns character in str at I (does have bounds checking) str.substr(position, length); - returns substring str.insert(position, str2); put str2 into str starting at position str.replace(position, length to be replaced, replacement string) str.find(str1, position) returns index of str1 in str (position optional) str.c_str() returns a cstring Helpful: getline(cin, str) : std::getline (std::cin,name); - input up to, not including null from cin to str
Palindrome wget http://home.adelphi.edu/~pe16132/csc270/note/S ampleCode/cplus/Palindrome.cpp logic ask user for string, test palindrome (isPal), report test palindrome: make entry lowercase, remove punctuation, and then reverse it. Is reversal = original? Send const string & as parms pass by ref (no copy) allow no change See string functions in use
Member Functions of the string class Example Constructors Remarks Default constructor creates empty string object str Creates a string object with data "string" Creates a string object that is a copy of aString, (which is a string object) string str string str("string"); string str(aString); Element Access str[i] Returns read/write reference to character in str at index i Returns read/write reference to character in str at index i Return the substring of the calling object starting at position and having length characters str.at(i) str.substr(position, length)
Member Functions of the string class Example Assignment/Modifiers Remarks Allocates space and initializes it to str1 s data, releases memory allocated to str1 and sets str1's size to that of str2. Character data of str2 is concatenated to the end of str1; the size is set appropriately Returns true if str is an empty string; returns false otherwise Returns a string that has str2 s data concatenated to the end of str1 s data. The size is set appropriately Inserts str2 into str beginning at position pos Removes a substring of size length beginning at position pos string str1 = str2; str1 += str2; str.empty(); str1 + str2 str.insert(pos, str2) str.remove(pos, length)
Member Functions of the string class Example Comparisons Remarks str1 == str2 str1 != str2; Compare for equality or inequality; returns a Boolean value. str1 < str2 str1 > str2 str1 >= str2 Four comparisons. All are lexicographical comparisons Returns index of the first occurrence of str1 in str. Returns index of the first occurrence of str1 in str; the search starts at position pos. Returns index of the first instance of any character in str1; the search starts at position pos. Returns index of the first instance of any character not in str1; the search starts at position pos str1 <= str2; str.find(str1) str.find(str1, pos) str.find_first_of(str1, pos) str.find_first_not_of(pos, length)
Converting string objects and C- Strings Sample String and cstring: char aCString[] = This is my C-string. ; std::string stringVariable; CString to String stringVariable = aCString; std::string stringVariable2 (aCString); NOT: strcpy(stringVariable, aCString); String to CString strcpy(aCString, stringVariable.c_str()); NOT: aCString = stringVariable; NOT: strcpy(ACString, stringVariable);
C-String: Input and Output In addition to cin >> and cout << , there are other input and output methods available when working with strings: getline()-- up to, not incl new line one char: get()-- get one char in put() -- put one char out putback () -- put one char back to input peek() -- look one char ahead many char: ignore() -- ignore # of char until match
more Versions of getline getline(cin, line); will read until the newline character. getline(cin, line, '?'); will read until the '?'. getline(cin, s1) >> s2; will read a line of characters into s1 and then store the next string (up to the next whitespace) in s2.
Summary Standard string vs cstring cstring functions std::string member methods Functions that use std::string cin / cout / character manipulation getline vs cin