Python String Manipulations Explained with Examples
In this comprehensive guide, you will learn various string manipulation techniques in Python. From slicing and formatting strings to finding characters and their frequencies, this tutorial covers it all. Explore how to replace, split, join, and strip strings effectively. Dive into practical examples to understand concepts better.
Download Presentation
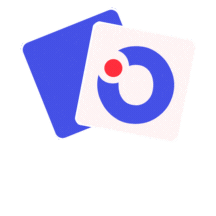
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Slicing seq[start] seq[start:end] seq[start:end:step] >>> word = "Let us kill some vampires!" >>> word[-1] '!' >>> word[-2] 's' >>> word[-2:] 's!' >>> word[:-2] 'Let us kill some vampire' >>> word[-0] 'L' >>> word[2:10] 't us kill'
Strings Format() >>> "{0} {1}".format("I'm the One!", "I'm not") "I'm the One! I'm not" >>> "{who} turned {age} this year!".format(who="Buffy", age=88) 'She turned 88 this year' >>> "The {who} was {0:.2f} last week".format(17.234, who="boy") 'Buffy turned 17.23 this year!
Count() str.count(value, start, end) >>> slayer = "Buffy is Buffy is Buffy" >>> slayer.count("Buffy", 0, -1) 2 >>> slayer.count("Buffy") 3 Find() str.find(value, start, end) >>> slayers = "Buffy and Faith" >>> slayers.find("y") 4 >>> slayers.find("k") -1 >>> slayers.index("y") 4 Replace() >>> slayer = "Buffy is Buffy is Buffy" >>> slayer.replace("Buffy", "who", 2) Split() string.split(sep, maxsplit) >>> S= Buffy-Slaying-Vamps-16' >>> job = S.split("- ,2) Buffy Slaying Vamps-16 Join() string.split(list) >>> slayer = ["Buffy", "Anne", "Summers"] >>> " ".join(slayer) 'Buffy Anne Summers' >>> "-<>-".join(slayer) 'Buffy-<>-Anne-<>-Summers' >>> "".join(slayer) 'BuffyAnneSummers' Strip() string.strip(character) >>> s= 999Buffy and Faith999" >>> s.strip("999") 'Buffy and Faith
Frequency of character in string ss='abracadabra def o(ch): return ord(ch)-ord('a') def freq2(ss): freq=[0]*(o('z')+1) for ch in ss: freq[o(ch)]+=1 for i in range(o('z')+1): if freq[i]!=0: print(chr(i+ord('a')),freq[i]) freq2(ss)
str='abracadabra' def freq(ss): s='abcdefghijklmnopqrstuvwxyz' freq=[0]*len(s) for ch in ss: for pos_ch2 in range(len(s)): if ch==s[pos_ch2]: freq[pos_ch2]+=1 def freq(ss): s='abcdefghijklmnopqrstuvwxyz' freq=[0]*len(s) for ch in ss: for ch2 in s: if ch==ch2: pos_ch2=s.find(ch2) freq[pos_ch2]+=1 for i in range(len(s)): if freq[i]!=0: print(s[i],freq[i]) for i in range(len(s)): if freq[i]!=0: print(s[i],freq[i]) freq(str)
Folosind count() Folosind dictionar def freq(ss): freq = {} for ch in ss: if ch in freq: freq[ch]+=1 else: freq[ch]=1 print(freq) def freq(ss): s='abcdefghijklmnopqrstuvwxyz' for ch in s: c=str.count(ch) if c: print(ch,c) Alta varianta def freq(ss): s='' freq=[] for ch in str: if not(ch in s): s += ch freq += [ss.count(ch)] for a, b in zip(s, freq): print(a, " : ", b) Folosind dictionar si count() def freq(ss): dic={} for ch in ss: dic[ch]=str.count(ch) print(dic) Folosind dictionar, set si count() print({ch : ss.count(ch) for ch in set(ss)})
def freq(ss): s=ss for ch in s: print('==',ch,s, ch in s) f,i=0,0 while i!=-1: s=s.replace(ch,'',1) i=s.find(ch) f +=1 print(ch,f, s) def freq(ss): s=ss while len(s)>0: ch=s[0] print('==',ch,s, ch in s) f,i=0,0 while i!=-1: s=s.replace(ch,'',1) i=s.find(ch) f +=1 print(ch,f, s) == a abracadabra True a 5 brcdbr == b brcdbr True b 2 rcdr == r rcdr True r 2 cd == a cd False a 1 cd == c cd True c 1 d == a d False a 1 d == d d True d 1 == a abracadabra True a 5 brcdbr == b brcdbr True b 2 rcdr == r rcdr True r 2 cd == c cd True c 1 d == d d True d 1 The ONLY completely safe loop to use which will work whatever you do to the lstring without making a copy is a while loop The iterator ch in s is evaluated once, which means that if you then modify your string, the iterator could potentially get lost
#ss=input('introdu numerele ') ss='0 1 2 3 4 5 6 7 8 9' l1=ss.split() print(l1) s1=''.join(l1) print(s1,s1[9],s1[-1] )#s1[10] print('s1[:3]{0},s1[3:]{1},s1[:] \ .format(s1[:3],s1[3:],s1[:])) print(s1[3:7],s1[-7:-3]) l2=[int(x) for x in l1] print(l2,sum(l2),len(l2), min(l2),max(l2)) s='abracadabra' print(s.find('d'),s.find('k'),s.find('ra')) print(s.count('a')) cod=[ord(x) for x in s] decod=[chr(x) for x in cod] print(cod,'\n',decod) mesaj=''.join(decod) print(mesaj) #toti indicii la care apare 'a' indici_a=[i for i in range(len(s)) if s[i]=='a'] print(indici_a) #nr consoane cons=[c for c in s if 'aeiou'.find(c)==-1] print(cons,len(cons)) Lab2 #codificare1 cod=[s[(i+ord(s[i]))%len(s)] for i in range(len(s))] f=[(i+ord(s[i]))%len(s) for i in range(len(s))] print(cod,'\n',f) decod=['0']*len(cod) #cod[j]=s[i] j=f[i] for i in range(len(cod)): decod[f[i]] =cod[i] print(''.join(decod)) #codificare2 def cod2(i): while i<0 or i>=len(s): if i>=len(s): i=len(s)-(i-(len(s)-1)) if i<0: i=-i return(i) cod=[s[cod2(i+ord(s[i]))] for i in range(len(s))] f=[cod2(i+ord(s[i])) for i in range(len(s))]
a=[1,-2,3] b=[1,2,1] c=[3,2,1] #produs scalar,vectorial,mixt,suma,modul sum([x*y for x,y in zip(a,b)]) [x+y for x,y in zip(a,b)] #trecere coord.polare <-> cartesiene 2D #nr de munti, insule #volum apa de ploaie #sum of diagonal elements [[1,3,5],[1,4,6],[7,6,9] #separate lists of string and nubers gadgets = ["Mobile", "Laptop", 100, "Camera", 310.28, "Speakers", 27.00, "Television", 1000, "Laptop Case", "Camera Lens"] str_items = [] num_items = [] for item in gadgets: if isinstance(item, str): str_items.append(item) elif isinstance(item,int) or \ isinstance(item, float): num_items.append(item) str_items.sort(key=str.lower, reverse=True) #Get first, second best scores from the list. [86,86,85,85,85,83,23,45,84,1,2,0] => 86, 85 #cod numeric personal S AA LL ZZ JJ NNN C 1730610155203 #check first and last words are same. #frecventa cuvinte Apples should compared with Apples #function encrypt abcaaadddbvvsscccc => abca3d3bv2c4
Numar numar roman Scrabble score word F I F T Y S T A T E S+ ---------------- A M E R I C A S E N D M O R E + ----------- M O N E Y #n spiral matrix nxn Spiral matrix of size 3 1 2 3 8 9 4 7 6 5 Spiral matrix of size 4 1 2 3 4 12 13 14 5 11 16 15 6 10 9 8 7 #fraction sum a/b+c/d=e/f 1/2+1/6=2/3 9 5 6 7 1 0 8 5 + ----------- 1 0 6 5 2 #toate tripletele pitagoreice sub 25
append() - Add an element to the end of the list extend() - Add all elements of a list to the another list insert() - Insert an item at the defined index remove() - Removes an item from the list pop() - Removes and returns an element at the given index clear() - Removes all items from the list index() - Returns the index of the first matched item count() - Returns the count of number of items passed as an argument sort() - Sort items in a list in ascending order reverse() - Reverse the order of items in the list copy() - Returns a shallow copy of the list
>>> people = ["Buffy", "Faith"] >>> people.remove("Buffy") >>> people ['Faith'] >>> people = ["Buffy", "Faith"] >>> people.pop() 'Faith' >>> people ['Buffy'] >>> people = ["Buffy", "Faith"] >>> people.index("Buffy") 0 >>> people = ["Buffy", "Faith", "Buffy"] >>> people.count("Buffy") 2 The sort() Method: Sorts the items of the list, in place: >>> people = ["Xander", "Faith", "Buffy"] >>> people.sort() >>> people ['Buffy', 'Faith', 'Xander'] >>> people.reverse() >>> people ["Xander", "Faith", "Buffy"] >>> people = ["Buffy", "Faith"] >>> people.append("Giles") >>> people ['Buffy', 'Faith', 'Giles'] >>> people[len(people):] = ["Xander"] >>> people ['Buffy', 'Faith', 'Giles', 'Xander'] >>> people = ["Buffy", "Faith"] >>> people.extend("Gil") >>> people ['Buffy', 'Faith', 'G', 'i', 'l'] >>> people += "Will" >>> people ['Buffy', 'Faith', 'G', 'i', 'l', 'W', 'i', 'l', 'l ] >>> people += ["Xander"] >>> people ['Buffy', 'Faith', 'G', 'i', 'l , 'W', 'i', 'l', 'l , 'Xander'] >>> people = ["Buffy", "Faith"] >>> people.insert(1, "Xander") >>> people ['Buffy', 'Xander', 'Faith']
List Comprehension pow2 = [2 ** x for x in range(10)] # Output: [1, 2, 4, 8, 16, 32, 64, 128, 256, 512] >>> odd = [x for x in range(20) if x % 2 == 1] >>> odd [1, 3, 5, 7, 9, 11, 13, 15, 17, 19] >>> [x+y for x in ['Python ','C '] for y in ['Language','Programming']] ['Python Language', 'Python Programming', 'C Language', 'C Programming']
import math G= 6.6738e-11 M= 1.9891e30 #Earth #L1 = 1.4710e11 #v1 = 3.0287e4 #Halley L1= 8.7830e10 v1 = 5.4529e4 y=2*G*M/L1 a=1 b=-y/v1 c=-(v1**2-y) v2=(-b-math.sqrt(b**2-4*a*c) )/2 L2=L1*v1/v2 A=(L1+L2)/2 B=(L1*L2)**0.5 T=2*math.pi*A*B/(L1*v1) print('L2={0:.4e}m v2={1:.4e}m/s T={2:3.4}years' .format(L2,v2,T/3600*1/24*1/365)) Halley L2=5.2822e+12 m v2=9.0668e+02 m/s T=76.08 years
L1=2e-9 me=7.29e-32 mh=5.47e-31 m=me*mh/(me+mh) #m=9.10938356e-31 h=6.62607004e-34 c=2.99792458e8 E=(h*h/(8*m))*(1/(L1*L1)) lambda0=(h*c/E)*1.0e9 E_eV=E/1.602176565e-19 print('lambda0={0:.0f}nm E={1:.2f}eV'.format(lambda0,E_eV)) lambda0=931nm E=1.33eV