Exploring Characters, Strings, and Cstring Library in C++
This content delves into the usage of characters, strings, and the cstring library in C++. Covering topics such as C-style strings, character literals, array functions, conversion functions, query functions, string I/O operations, and more, it provides a comprehensive overview essential for mastering object-oriented programming in C++.
Download Presentation
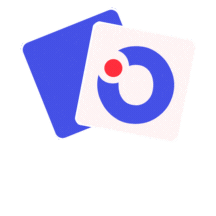
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Characters, Strings, and the cstring Library Andy Wang Object Oriented Programming in C++ COP 3330
Recap A C-style string is a char array that ends with the null character Character literals are in single quotes a , \n , $ String literals are in double quotes Hello world\n The null character is an implicit part of a string literal
Recap The name of an array points to the first element of an array When an array is passed into a function, the function can access the original array
The cctype Library Conversion functions return the ASCII value of a character int touper(int c) returns the uppercase version of c int tolower(int c) returns the lowercase version of c
Query functions Return true (non-zero) or false (0) to question posed by the function s name int isdigit(int c) int isalpha(int c) int isalnum(int c) // a digit or a letter int islower(int c); int isupper(int c);
Query functions int isxdigit(int c) int isspace(int c) int iscntrl(int c) int ispunct(int c) // hex digit (0-9, a-f)? // white space? // control character? // printing character other than space, // letter, digit? int isprint(int c) int isgraph(int c) // printing character (including )? // printing other other than (space)?
String I/O Insertion (<<) and extraction (>>) char greeting[20] = Hello, world ; cout << greeting; char lastname[20]; cin >> lastname; // prints Hello, world // reads a string into lastname // adds \0 automatically The insertion operator << will print the content of a char array, up to the first null character The extraction operator >> will read in a string, and stop at a white space
String I/O The cin examples reads one word at a time How to read a whole sentence into a string?
Reading strings: get and getline Two member functions in class istream (in the iostream library) char *get(char str[], int length, char delimiter = \n ); char *getline(char str[], int length, char delimiter = \n ); Note that the get function with only one parameter can only read a single character from the input stream char ch; ch = cin.get(); // extracts one char and returns it cin.get(ch); // extracts one char and stores in ch
get and getline Functions (with Three Parameters) Read and store a c-style string str is the char array where the data will be stored Note that it is passed by reference length is the size of the array delimiter (optional, with \n as default) specifies when to stop reading Automatically appends \0
Example Sample calls char buffer[80]; cin >> buffer; cin.get(buffer, 80, , ); // reads up to the first comma cin.getline(buffer, 80); // reads an entire line (up to endl) So, what is the difference between get and getline? get will leave the delimiter character on the input stream getline will extract and discard the delimiter // reads one word into buffer
Another Example Input Hello, world Joe Smith. He says hello. Code char greeting[15], name[10], other[20]; cin.getline(greeting, 15); cin.get(name, 10, . ); cin.getline(other, 20); // Hello, world // Joe Smith // . He says hello.
Example String Calls http://www.cs.fsu.edu/~myers/c++/examples/strin gs/io1.cpp
io1.cpp #include <iostream> using name space std; int main() { char string1[30], string2[30], string3[30], string4[30]; // print some instructions cin >> string1; // get one word, append it with \0 cin.getline(string2, 30, , ); // get line, drop , cin.get(string3, 30, e ); // get up to e cin.get(string4, 30); // get 30 chars
io1.cpp cout << \n\nOutputs:\n ; cout << string1 = << string1 << endl; cout << string2 = << string2 << endl; cout << string3 = << string3 << endl; cout << string4 = << string4 << endl; return 0; }
Program Execution > To be, or not to be, that is the question.
Program Execution > To be, or not to be, that is the question. Outputs: string1 = To string2 = be string3 = or not to b string4 = e, that is the question.
Standard C String Library To use it, #include <cstring> int strlen(const char str[]); Takes a string argument, returns its length (excluding \0 ) Sample calls char phrase[30] = Hello, world ; cout << strlen( Greetings, Earthling! ); // prints 21 int length = strlen(phrase); // stores 12
strcpy char *strcpy(char str1[], const char str2[]); Takes two string arguments, and copies the contents of the second string into the first string Sample calls char buffer[80], firstname[30], lastname[30] = Smith ; strcpy(firstname, Billy Joe Bob ); strcpy(buffer, lastname); cout << firstname; // prints Billy Joe Bob cout << buffer; // prints Smith
strcat char *strcat(char str1[], const char str2[]); Takes two string arguments, and concatenates the second one onto the first Sample calls char buffer[80] = Dog ; char word[] = food ; strcat(buffer, word); strcat(buffer, breath ); // buffer is now Dogfood breath // buffer is now Dogfood
strcmp int strcmp(const char str1[], const char str2[]); Takes two string arguments Returns 0 if they are equal Returns a negative number if str1 comes before str2 (based on ASCII code) Returns a positive number if str2 comes before str1
strcmp Sample Calls char word1[30] = apple ; char word2[30] = apply ; if (strcmp(word1, word2) != 0) cout << The words are different\n ; strcmp(word1, word2); // < 0, word1 comes first strcmp(word1, apple ); // 0, words are the same strcmp( apple , Zebra ); // > 0, uppercase first
strcmp Note strcmp relies on \0 at the end of each string No built-in bound checking in C++
strncpy, strncat, strncmp Same as strcpy, strcat, strcmp Takes an additional integer N as an argument Processes up to \0 or N characters
strncpy, strncat, strncmp Examples char buffer[80]; char word[11] = applesause ; char bigword[] = supercalifragilisticexpialidocious ; strncpy(buffer, word, 5); // buffer = apple strncat(buffer, piecemeal , 4); // buffer = apple pie strncmp(buffer, apple , 5); // returns 0 strncpy(word, bigword, 10); // word = supercalif