Understanding Inheritance and Polymorphism in Object-Oriented Programming
Inheritance allows classes to form hierarchical relationships, facilitating code sharing and reuse. Superclasses are extended by subclasses, which inherit their behavior. This relationship creates an "is-a" connection, such as Tiger extending Cat. Polymorphism, demonstrated through a set of classes like MusicPlayer, TapeDeck, iPod, and iPhone, showcases how methods can be overridden to exhibit varying behaviors based on the specific class instance. Through examples and explanations, the concepts of inheritance and polymorphism are explored in detail in the context of object-oriented programming.
Download Presentation
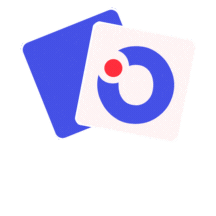
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Inheritance + Polymorphism Hitesh Boinpally Summer 2023
Agenda Final Logistics Inheritance Different Errors Polymorphism Lesson 13 - Summer 2023 2
Inheritance Inheritance: Forming hierarchial relationships between classes Allows for sharing / reusing of code between classes Superclass: The class being extended Subclass: The class that inherits behavior from superclass Gains copy of every method Lesson 13 - Summer 2023 4
Inheritance Inheritance: Forming hierarchial relationships between classes Allows for sharing / reusing of code between classes Superclass: The class being extended Subclass: The class that inherits behavior from superclass Gains copy of every method Inheritance forms an is-a relationship Tiger extends Cat Means that Tiger is-a Cat Cat Tiger Lesson 13 - Summer 2023 5
public class MusicPlayer { public void m1() { S.o.pln("MusicPlayer1"); } } public class TapeDeck extends MusicPlayer { public void m3() { S.o.pln("TapeDeck3"); } } public class IPod extends MusicPlayer { public void m2() { S.o.pln("IPod2"); m1(); } } public class IPhone extends IPod { public void m1() { S.o.pln("IPhone1"); super.m1(); } m1() m2() m3() public void m3() { S.o.pln("IPhone3"); } MusicPlayer } TapeDeck IPod IPhone Lesson 13 - Summer 2023 7
public class MusicPlayer { public void m1() { S.o.pln("MusicPlayer1"); } } public class TapeDeck extends MusicPlayer { public void m3() { S.o.pln("TapeDeck3"); } } public class IPod extends MusicPlayer { public void m2() { S.o.pln("IPod2"); m1(); } } public class IPhone extends IPod { public void m1() { S.o.pln("IPhone1"); super.m1(); } m1() m2() m3() public void m3() { S.o.pln("IPhone3"); } / / MP1 MusicPlayer } / MP1 TD3 TapeDeck IPod2 m1() / MP1 IPod IPhone1 MP1 IPod2 m1() IPhone3 IPhone Lesson 13 - Summer 2023 8
m1() m2() m3() MusicPlayer var1 = new TapeDeck(); MusicPlayer var2 = new IPod(); MusicPlayer var3 = new IPhone(); IPod var4 = new IPhone(); Object var5 = new IPod(); Object var6 = new MusicPlayer(); / / MP1 MusicPlayer / MP1 TD3 TapeDeck IPod2 m1() / MP1 IPod var1.m1(); IPhone1 MP1 IPod2 m1() IPhone3 IPhone var3.m1(); var4.m2(); var3.m2(); var5.m1(); Lesson 13 - Summer 2023 9
m1() m2() m3() MusicPlayer var1 = new TapeDeck(); MusicPlayer var2 = new IPod(); MusicPlayer var3 = new IPhone(); IPod var4 = new IPhone(); Object var5 = new IPod(); Object var6 = new MusicPlayer(); / / MP1 MusicPlayer / MP1 TD3 TapeDeck IPod2 m1() / MP1 IPod var1.m1(); MusicPlayer1 var3.m1(); IPhone1 / MusicPlayer1 var4.m2(); IPod2 / IPhone1 / MusicPlayer1 var3.m2(); Compiler Error (CE) var5.m1(); Compiler Error (CE) IPhone1 MP1 IPod2 m1() IPhone3 IPhone Lesson 13 - Summer 2023 10
m1() m2() m3() MusicPlayer var1 = new TapeDeck(); MusicPlayer var2 = new IPod(); MusicPlayer var3 = new IPhone(); IPod var4 = new IPhone(); Object var5 = new IPod(); Object var6 = new MusicPlayer(); / / MP1 MusicPlayer / MP1 TD3 TapeDeck IPod2 m1() / MP1 IPod ((TapeDeck) var1).m2(); IPhone1 MP1 IPod2 m1() IPhone3 IPhone ((IPod) var3).m2(); ((IPhone) var2).m1(); ((TapeDeck) var3).m2(); Lesson 13 - Summer 2023 11
m1() m2() m3() MusicPlayer var1 = new TapeDeck(); MusicPlayer var2 = new IPod(); MusicPlayer var3 = new IPhone(); IPod var4 = new IPhone(); Object var5 = new IPod(); Object var6 = new MusicPlayer(); / / MP1 MusicPlayer / MP1 TD3 TapeDeck IPod2 m1() / MP1 IPod ((TapeDeck) var1).m2(); Compiler Error (CE) ((IPod) var3).m2(); IPod2 / IPhone1 / MusicPlayer1 ((IPhone) var2).m1(); Runtime Error (RE) ((TapeDeck) var3).m2(); Compiler Error (CE) IPhone1 MP1 IPod2 m1() IPhone3 IPhone Lesson 13 - Summer 2023 12
The Rules Lesson 13 - Summer 2023 13