Exploring Texture Mapping in Computer Graphics
Delve into the world of texture mapping as a solution to the smoothness of polygons in computer graphics. Learn how to create textured scenes using techniques such as bump mapping and environmental mapping. Discover the process of representing textures, working with curved surfaces, and mapping onto different geometries like rectangles and cylinders.
Download Presentation
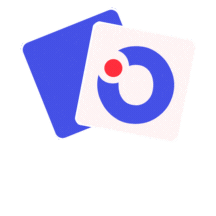
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Graphics, Fall 2023 Lecture 17: Texture Mapping The Problem with Polygons: To date, we have learned how to create objects using multiple polygonal surfaces. We could use this technique to create complex scenes with many objects, for example a field of grass. Problem: It would take huge amounts of time to render. Polygons tend to look too smooth for some purposes. Many surfaces have lots of variation in them (e.g. carpet, oranges, wood grain). 1
Creating Textured Scenes To create scenes with lots of variation, we can use a variety of techniques. Among them are: Texture Mapping: Create a texture or pattern and map it onto a surface. Bump Map: Perturb the surface of an object by slightly varying the surface normals. Environmental Map: Map the environment onto a surface (e.g. reflections. 2
Texture Mapping With texture mapping, we map a texture or image onto an object. Creating the texture: Write program to create regular or random texture. OR Load in an image map and use that as the texture. Textures can be in 1, 2 or 3 dimensions: 1-D textures: Map onto a line or curve 2-D textures: Map onto a surface 3-D textures: Map into a volume. Will see texture if take slices through the volume. We will examine only 2-D textures. 3
Representing a Texture A 2D texture is represented as a 2D pattern: T(s, t) s and t are called texture coordinates. T is stored in a 2D array (n x m) of texture elements, called texels. Textures are rendered as discrete elements. A texture map associates a unique point of T with each point on a geometric object. The object is then mapped to screen coordinates. y y y x z projection x x t z s 4
Working with Curved Surfaces When mapping texture onto a curved surface, it is useful to represent the surface in parametric form: x(u,v) y(u,v) z(u,v) p(u,v) = We can relate the texture coordinates to the image coordinates as follows: u = as + bt + c v = ds + et + f where ae does not equal bd 5
Mapping onto a rectangle Mapping onto a rectangle is like a simple coordinate switch: (smax, tmax) t (umax, vmax) v s u (smin, tmin) (umin, vmin) s -smin smax-smin t -tmin tmax-tmin ( ) u = umin+ umax-umin ( ) v=vmin+ vmax-vmin 6
Mapping a square onto a cylinder Define texture on a unit square: 0 t 1 0 s 1 Parametric representation of cylinder: x =rcos(2pu) 0 u,v 1 y=rsin(2pu) z =vh Map with u = s and v = t: t v s u p(x, y, z) 7
Problems in mapping 2D to 3D For some 3D surfaces, it is hard to map from 2D to 3D. For example, imagine trying to wrap a piece of paper around a soccer ball! Mapping a rectangular texture onto a sphere is difficult to do without generating distortions in the texture. 8
Methods for Mapping 2D to 3D One solution is to unfold the 3D object into a 2D surface: Unfolded cube: Map the 2D texture onto the unfolded object, then fold it again. This does not work as well for a sphere. Flattening a sphere leads to distortions (e.g. world maps). 9
Mapping to an intermediate surface Another solution is to map the texture onto an intermediate surface (a sphere, a box or a cylinder) and then map from there onto the 3D object. Ways of mapping: Normals of intermediate object to final object Normals of final object to intermediate object Mapping from center of final object Different choices give different results. 10
Aliasing Because we are mapping discrete points (texels) onto discrete points (pixels) there can be problems if the relative sizes and resolutions do not match. Aliasing occurs in repeated textures, when sampling from the texture skips over pieces of the texture. t v Disappearing Stripes s u Aliasing can cause texture elements to disappear and can also cause moire patterns. Area averaging: One way to deal with aliasing is to assign the average of the texel with the nearest neighboring texels to the point on the surface. This limits the moire patterns, but does not help with the disappearing stripes (we just see gray instead of white).
Scaling the texture Problems arise when the texture scale does not match the image scale. We may have several texels mapping to a single pixel (or vice versa). t v s u Solutions: Use averaging: Use the nearest texel value; Or Use Mipmapping Mipmapping works by creating a set of texture arrays for different resolutions. E.g. 64 x 64, 32 x 32, 16 x 16, etc. OpenGL then chooses the appropriate mipmap for a given object. The one with the closest matching resolution will be used. 12
Texture Mapping in WebGL Texture mapping in WebGL is done once the primitives are rasterized as each fragment is processed. Texture mapping acts as part of the shading process, as the fragment color is computed. Texture mapping uses the coordinate transformation between the texture (s, t) and surface (u, v) to compute the texel value to place at a given pixel position. 13
Specifying a Texture Texture is specified as an array of texels, of type Uint8: var myTexels = new Uint8Array(4 * 256 * 256); One byte for each of red, green, blue, and a. Width is power of 2 Height is power of 2 Create texture by either: 1) Write a program to generate the texture OR 2) Read it in from an image file. 14
Reading from an image file 1) We can read in an image within the JavaScript file: var image = new Image(); image.src = "myPicture.gif"; 2) Read in the texture in the html file: <img id = "texImage" src = "myImage.gif" hidden></img> and access it in the JavaScript file: var image = document.getElementById("texImage"); 15
Configuring the Texture texture = gl.createTexture(); gl.bindTexture( gl.TEXTURE_2D, texture ); //Flip the Y values to match the WebGL coordinates gl.pixelStorei(gl.UNPACK_FLIP_Y_WEBGL, true); //Specify the image as a texture array: gl.texImage2D( gl.TEXTURE_2D, 0, gl.RGB, gl.RGB, gl.UNSIGNED_BYTE, image ); //Link texture to a sampler in fragment shader gl.uniform1i(gl.getUniformLocation(program, "texture"), 0); 16
Specifying that an array holds a texture We specify that a given array contains texel values with the following WebGL function: gl.texImage2D(gl.TEXTURE_2D, 0, gl.RGBA, width, height, 0, gl.RGBA, gl.UNSIGNED_BYTE, imageData); Generally: gl.texImage2D(target, level, iformat, width, height, border, format, type, texelArray); 17
Set up Texture Coordinates var texCoord = [ vec2(0, 0), vec2(0, 1), vec2(1, 1), vec2(1, 0) ]; 1 2 (0, 1) (1, 1) t 0 3 (0, 0) (1, 0) s 18
Assigning a Texture to a Polygon function quad( a, b, c, d) { pointsArray.push(vertices[a]); texCoordsArray.push(texCoord[0]); pointsArray.push(vertices[b]); texCoordsArray.push(texCoord[1]); pointsArray.push(vertices[c]); texCoordsArray.push(texCoord[2]); pointsArray.push(vertices[a]); texCoordsArray.push(texCoord[0]); pointsArray.push(vertices[c]); texCoordsArray.push(texCoord[2]); pointsArray.push(vertices[d]); texCoordsArray.push(texCoord[3]); } 19
Passing Texture Coordinates to the Vertex Shader var tBuffer = gl.createBuffer(); gl.bindBuffer( gl.ARRAY_BUFFER, tBuffer ); gl.bufferData( gl.ARRAY_BUFFER, flatten(texCoordsArray), gl.STATIC_DRAW ); var vTexCoord = gl.getAttribLocation( program, "vTexCoord" ); gl.vertexAttribPointer( vTexCoord, 2, gl.FLOAT, false, 0, 0 ); gl.enableVertexAttribArray( vTexCoord ); 20
Specifying Texture Parameters Specify Texture parameters with gl.texParameteri( ) a) Specifying Texture wrapping: What happens if the texture coordinates go out of bounds? Can specify wrapping (start at 0 again) Or, can clamp the value (with the value of the last texel). gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_WRAP_S, gl.REPEAT); or gl.TEXTURE_WRAP_T or gl.CLAMP_TO_EDGE 21
Dealing with Mismatched Scales Magnification is when each texel is larger than each pixel. Minification is when each texel is smaller than each pixel. In these cases, you can choose to use the nearest texel, or you can choose to use a weighted average of neighboring texels (linear filtering). gl.texParameteri(gl.TEXTURE_2D, gl.TEXTURE_MIN_FILTER, gl.LINEAR); glTexParameteri(gl.TEXTURE_2D, gl.TEXTURE_MAG_FILTER, gl.LINEAR); Or gl.NEAREST 22
The vertex shader attribute vec4 vPosition; attribute vec4 vColor; attribute vec2 vTexCoord; // Input texture coordinates varying vec4 fColor; varying vec2 fTexCoord; // output to fragment shader void main() { // Code for transformations, if any fColor = vColor; fTexCoord = vTexCoord; gl_Position = vPosition; } 23
The fragment shader precision mediump float; varying vec4 fColor; varying vec2 fTexCoord; uniform sampler2D texture; //Mechanism to sample texture void main() { //Blend texture and color gl_FragColor = fColor * texture2D( texture, fTexCoord ); //If want texture alone, use //gl_FragColor = texture2D(texture, fTexCoord); } 24