Understanding Arrays of Objects in Programming
This lecture delves into the concept of arrays of objects, showcasing multi-dimensional arrays and discussing the steps involved in handling arrays of primitive types versus arrays of objects in Java programming. It includes insights on declaring, creating, and populating arrays, along with a practical example involving a Student class and creating a unit list. The tutorial emphasizes the importance of using arrays of objects effectively in programming tasks.
Download Presentation
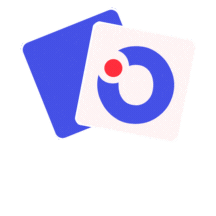
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
ARRAYS II CITS1001
2 Scope of this lecture Arrays of objects Multi-dimensional arrays The Game of Life (next lecture)
3 Arrays of objects The arrays we have seen so far had elements of a primitive type int[], double[], boolean[], etc. But an array can also hold objects e.g. Term[] Arrays can also be two-dimensional e.g. int[][] Arrays can also be three-dimensional, or more But we won t get to that in CITS1001
4 Arrays of objects When using an array of primitive type, there are two steps involved Declare the variable to refer to the array Create the space for the array elements using new When using an array of object type, there are three steps involved Declare the variable to refer to the array Create the space for the array elements using new Populate the array with objects by repeatedly using new, often in a loop
5 A Student class public class Student { private String studentNumber; private int mark; public Student(String studentNumber, int mark) { this.studentNumber = studentNumber; this.mark = mark; } public String getStudentNumber() { return studentNumber; } A skeleton version of a possible Student class in a student records system public int getMark() { return mark; } }
6 Creating a unit list Declare Student[] unitList; Create unitList = new Student[numStudents]; unitList[0] = new Student( 042371X ,64); unitList[1] = new Student( 0499731 ,72); unitList[2] = new Student( 0400127 ,55); unitList[numStudents-1] = new Student( 0401332 ,85); Populate
7 The three steps Declare Create Using new Populate (repeatedly) Each object needs new to be executed null null null null null null unit unit[0] unit[1] unit[2] unit[3] unit[4]
8 Using arrays of objects Using an array of objects is the same as using any array The syntax is the same, and elements can be used in the same ways You just need to remember that the elements are objects! private Student top(Student[] unitlist) { Student top = unitlist[0]; for (Student si : unitlist) if (si.getMark() > top.getMark()) top = si; return top; }
9 Compare with max private int max(int[] a) { int max = a[0]; for (int i : a) if (i > max) max = i; return max; }
10 Method signatures int max(int[] a) Student top(Student[] unitList)
11 Initialisation int max = a[0]; Declare a variable to hold the best so far , and initialise it to the first element in the array Student top = unitList[0];
12 Processing for (int i : a) if (i > max) max = i; Check each element in turn, compare it with the best so far, and update the best if necessary for (Student si : unitList) if (si.getMark() > top.getMark()) top = si;
13 Return return max; Finally return the extreme element the highest intor the Studentwith the best mark return top;
14 What if we want to find the highest mark? We could copy-and-paste top, and edit the details to create a new method private int highestMark(Student[] unitlist) { int max = unitlist[0].getMark(); for (Student si : unitlist) if (si.getMark() > max) max = si.getMark(); return max; } No!!
15 Much better to use the existing method! Do not write another looping method! Use the already-written functionality private int highestMark(Student[] unitlist) { return top(unitlist).getMark(); }
16 2D arrays We sometimes need arrays with more than one dimension int[][] a = new int[4][3]; 0 0 0 This creates an array with four rows and three columns a[0][2] a[0][0] a[0][1] 0 0 0 a[1][1] a[1][2] a[1][0] The row index ranges from 0 3 and the column index from 0 2 0 0 0 a[2][0] a[2][1] a[2][2] 0 0 0 a[3][0] a[3][1] a[3][2]
17 2D arrays contd. This is really an array where each element is itself an array a is a valid variable, representing the whole thing a[0] is a valid variable, representing the first row a[0][2] is a valid variable, representing the last element in the first row
18 2D arrays contd. 0 0 0 a[0] a[0][0] a[0][1] a[0][2] 0 0 0 a[1] a[1][0] a[1][1] a[1][2] a 0 0 0 a[2] a[2][0] a[2][1] a[2][2] 0 0 0 a[3] a[3][0] a[3][1] a[3][2]
19 2D arrays contd. Given that an array is an object, this is really just a special case of an array of objects The single statement int[][] a = new int[4][3]; declares the array variable a, creates the array, and populates it!
20 2D arrays examples A 2D array is normally processed with two nested for-loops private int sum(int[][] a) { int sum = 0; for (int i = 0; i < a.length; i++) for (int j = 0; j < a[i].length; j++) sum = sum + a[i][j]; return sum; }
21 2D arrays examples Suppose we want to return the index of the first row containing any element that is true private int firsttrue(boolean[][] a) { for (int i = 0; i < a.length; i++) for (int j = 0; j < a[i].length; j++) if (a[i][j]) return i; return -1; }
22 2D arrays example We will illustrate the use of 2D arrays further with a case study of The Game of Life http://en.wikipedia.org/wiki/Conway s_Game_of_Life In the next lecture And there s plenty of practice in the lab too