Understanding Pointers and Arrays in C Programming
Variables in C programming have specific memory locations, and each variable occupies memory space. Arrays in C take up memory depending on the number of elements. Pointers are special variables that store memory addresses instead of values directly. They can point to other variables and be used to manipulate them indirectly.
Download Presentation
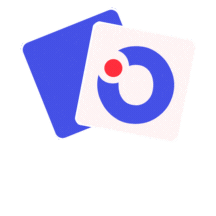
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Pointers and Arrays COP3223 INTRODUCTION TO PROGRAMMING WITH C DR. MATTHEW B. GERBER
Variables and Memory Whenever you declare a variable, it exists somewhere in the computer s memory That location must, eventually, be a number The compiler that translates your code into a program, and the program loader that runs that program on behalf of the operating system, take care of all of the processes of: Deciding where the variable should go Making space for it there Mapping the variable name to that location Keeping track of the location if the running program (or process) is moved around in memory What do you need to know out of this? Every variable exists in memory Therefore, every variable has a location in memory
Arrays and Memory When you declare a single variable, it always takes a small amount of memory int i; This takes somewhere between two and eight bytes depending on what you re compiling it on If you declare this array int my_array[100]; it obviously takes up 100 times that
Pointers A pointer is a kind of variable that doesn t have memory allocated to it by itself Instead, it points to another variable int *i_ptr; i_ptr is now a pointer to an integer (or simply pointer to integer) We can dereference it, and use it to modify whatever variable it points to
Pointers A pointer is a kind of variable that doesn t have memory allocated to it by itself Instead, it points to another variable int i; int *i_ptr; i_ptr = &i; // now points to i int *i_ptr; i_ptr is now a pointer to an integer (or simply pointer to integer) We can dereference it, and use it to modify whatever variable it points to
Pointers A pointer is a kind of variable that doesn t have memory allocated to it by itself Instead, it points to another variable int i; int *i_ptr; i_ptr = &i; // now points to i int *i_ptr; i = 3; // now i == 3 (*i_ptr) = 4; // now i == 4 i_ptr is now a pointer to an integer (or simply pointer to integer) We can dereference it, and use it to modify whatever variable it points to
Pointers A pointer is a kind of variable that doesn t have memory allocated to it by itself Instead, it points to another variable int i; int *i_ptr; i_ptr = &i; // now points to i int *i_ptr; i = 3; // now i == 3 (*i_ptr) = 4; // now i == 4 i_ptr is now a pointer to an integer (or simply pointer to integer) We can dereference it, and use it to modify whatever variable it points to if(i == 4) { // true! do_stuff(i); }
Pointers A pointer is a kind of variable that doesn t have memory allocated to it by itself Instead, it points to another variable int i; int *i_ptr; i_ptr = &i; // now points to i int *i_ptr; i = 3; // now i == 3 (*i_ptr) = 4; // now i == 4 i_ptr is now a pointer to an integer (or simply pointer to integer) We can dereference it, and use it to modify whatever variable it points to if(i == 4) { // true! do_stuff(i); } if((*i_ptr) == 4) { // also true! do_other_stuff(i_ptr); // Wait... }
Pointers and Functions The mechanics of pointers let us make variables declared in one function visible to another
Pointers and Functions The mechanics of pointers let us make variables declared in one function visible to another Normally, passed variables work like this void f(float fx) { fx = 3.0; // This variable is local to f } int main(void) { float x; f(x); // x is still uninitialized return 0; }
Pointers and Functions The mechanics of pointers let us make variables declared in one function visible to another Normally, passed variables work like this But by using pointers void f(float *fx) { (*fx) = 3.0; // Dereference and assign } int main(void) { float x; f(&x); // Now x = 3.0! return 0; }
Pointers and Pitfalls Pointers are dangerous Let s say that again Pointers are dangerous
Pointers and Pitfalls Pointers are dangerous Let s say that again Pointers are dangerous Anytime you pass a pointer, you re inviting a function to change the value of that variable We call these side effects of functions, and we don t like them
Pointers and Pitfalls Pointers are dangerous Let s say that again Pointers are dangerous Anytime you pass a pointer, you re inviting a function to change the value of that variable We call these side effects of functions, and we don t like them When a function only needs to return one value, you shouldn t pass a pointer x = f(x) is a better pattern than f(&x)
Pointers and Pitfalls Pointers are dangerous Let s say that again Pointers are dangerous Anytime you pass a pointer, you re inviting a function to change the value of that variable We call these side effects of functions, and we don t like them When a function only needs to return one value, you shouldn t pass a pointer x = f(x) is a better pattern than f(&x) There are two cases where you really have to pass pointers The first case is when a function needs to return more than one value The second is complicated
Pointers and Arrays Remember how declaring array variables works int my_array[100];
Pointers and Arrays Remember how declaring array variables works int my_array[100]; Once you declare my_array, it is treated as a pointer
Pointers and Arrays Remember how declaring array variables works int my_array[100]; Once you declare my_array, it is treated as a pointer The compiler creates space for a hundred ints, creates a pointer to them, and names it my_array
Pointers and Arrays Remember how declaring array variables works int i; int my_array[100]; int *my_array_ptr; int my_array[100]; Once you declare my_array, it is treated as a pointer The compiler creates space for a hundred ints, creates a pointer to them, and names it my_array This means a few things
Pointers and Arrays Remember how declaring array variables works int i; int my_array[100]; int *my_array_ptr; int my_array[100]; // These do the same thing i = my_array[0]; // Element 0 of my_array i = (*my_array); // Dereference my_array Once you declare my_array, it is treated as a pointer The compiler creates space for a hundred ints, creates a pointer to them, and names it my_array This means a few things
Pointers and Arrays Remember how declaring array variables works int i; int my_array[100]; int *my_array_ptr; int my_array[100]; // These do the same thing i = my_array[0]; // Element 0 of my_array i = (*my_array); // Dereference my_array Once you declare my_array, it is treated as a pointer The compiler creates space for a hundred ints, creates a pointer to them, and names it my_array This means a few things my_array_ptr = my_array;
Pointers and Arrays Remember how declaring array variables works int i; int my_array[100]; int *my_array_ptr; int my_array[100]; // These do the same thing i = my_array[0]; // Element 0 of my_array i = (*my_array); // Dereference my_array Once you declare my_array, it is treated as a pointer The compiler creates space for a hundred ints, creates a pointer to them, and names it my_array This means a few things my_array_ptr = my_array; i = my_array_ptr[0]; // Still element 0 of // my_array i = (*my_array_ptr); // Dereference my_array_ptr
Pointers and Arrays Remember how declaring array variables works int i; int my_array[100]; int *my_array_ptr; int my_array[100]; // These do the same thing i = my_array[0]; // Element 0 of my_array i = (*my_array); // Dereference my_array Once you declare my_array, it is treated as a pointer The compiler creates space for a hundred ints, creates a pointer to them, and names it my_array This means a few things my_array_ptr = my_array; i = my_array_ptr[0]; // Still element 0 of // my_array i = (*my_array_ptr); // Dereference my_array_ptr // This works but you don t need to do it. my_array_ptr = &(my_array[0]);
Pointers and Arrays Remember how declaring array variables works int i; int my_array[100]; int *my_array_ptr; int my_array[100]; // These do the same thing i = my_array[0]; // Element 0 of my_array i = (*my_array); // Dereference my_array Once you declare my_array, it is treated as a pointer The compiler creates space for a hundred ints, creates a pointer to them, and names it my_array This means a few things my_array_ptr = my_array; i = my_array_ptr[0]; // Still element 0 of // my_array i = (*my_array_ptr); // Dereference my_array_ptr // This works but you don t need to do it. my_array_ptr = &(my_array[0]); // Don t do this. my_array_ptr = &my_array;
Pointers and Arrays Remember how declaring array variables works int i; int my_array[100]; int *my_array_ptr; int my_array[100]; // These do the same thing i = my_array[0]; // Element 0 of my_array i = (*my_array); // Dereference my_array Once you declare my_array, it is treated as a pointer The compiler creates space for a hundred ints, creates a pointer to them, and names it my_array This means a few things But here s the important one my_array_ptr = my_array; i = my_array_ptr[0]; // Still element 0 of // my_array i = (*my_array_ptr); // Dereference my_array_ptr // This works but you don t need to do it. my_array_ptr = &(my_array[0]); // Don t do this. my_array_ptr = &my_array;
Functions, Pointers and Arrays When you declare a pointer in a function header, you can pass an array into that pointer void fill_array(int *array, int count)
Functions, Pointers and Arrays When you declare a pointer in a function header, you can pass an array into that pointer The function can then access and work with that array void fill_array(int *array, int count) { int i; for(i = 0; i < count; i = i + 1) { array[i] = i; } Remember, it s working with the same array as the function that called it } The value isn t copied the pointer is given to the function instead int main(void) { int my_array[100]; fill_array(my_array, 100); return 0; }
Functions, Pointers and Arrays When you declare a pointer in a function header, you can pass an array into that pointer The function can then access and work with that array void get_input(char *header, char *buf, int n) { printf(header); fgets(buf, n, stdin); Remember, it s working with the same array as the function that called it } The value isn t copied the pointer is given to the function instead This is also how fgets() and almost all string functions work int main(void) { char input_string[80]; char *header = Hi. Enter something: ; get_input(header, input_string, 79); return 0; }