Introduction to Arrays in Computer Science
Explore the concept of arrays in computer science, a special type of variable that can hold collections of elements of the same type. Learn about the characteristics of arrays, how to declare and initialize them, and see examples of specifying array sizes. Develop a foundational understanding of arrays through practical insights and humor.
Download Presentation
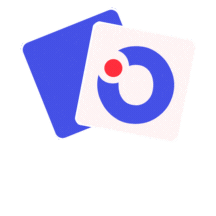
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Chapter 9 - Arrays CITA/CSCI 140
Introductory Approach Open & examine some files Copy/Paste & remix Hands-on with some
Arrays Humor Why did the programmer Why did the programmer quit his job? quit his job? He didn't get arrays...
What is an Array An array is a special variable, which can hold a collection of elements of the same type. Examples: A list of colors A list of objects, such as rain drops or cars A list of players in a game A sequential list of numbers to associate with other variables such as x, y, w, h, color, etc.
Characteristics of Arrays Arrays start with 0. Arrays have a fixed size. Each element in an array has a unique position, in which the order is crucial. Each index/position of an array is matched with an element. E.g. array of your siblings you might be #2. The size can be: - A number - A variable of int type - An expression that calculates an integer
Declaring and Initializing Arrays Declaring: int [] myArray; String [] myWord = new String[6]; Declaring and initializing with values : float[] myNums = { 1.0, 3.2, 6, 9.5};
from from processing.org/tutorials/arrays processing.org/tutorials/arrays, , an easier way to remember array declarations an easier way to remember array declarations // Declare int[] data; // Declare & create int[] data = new int[5]; // Create data = new int[5]; // Assign data[0] = 19; data[1] = 40; data[2] = 75; data[3] = 76; data[4] = 90; // Assign data[0] = 19; data[1] = 40; data[2] = 75; data[3] = 76; data[4] = 90; // Declare, create, assign int[] data = { 19, 40, 75, 76, 90 };
Examples of specifying size color [] swatches = new color[4]; int [] storeLoc = new int [12]; int cities = 5; String [] mycities = new String [cities]; Sidenote, let s try println for mycities.length Next, let s your 5th city at 20x and 30y Using variable value int num=60; Car[] cars = new Car [num];
Three ways to fill with value (initialize) 1. Hard code the values in each spot. 2. Type out a list in curly brackets separated by commas. 3. Iterate through the elements in an array. See next slide
Table: Three ways to initialize Hard code the values in each spot String [] stuff = new String [4]; stuff[0] = "Buick"; stuff[1] = "Nissan"; stuff[2] = "Toyota"; stuff[3] = "Ford"; Type out a list in curly brackets separated by commas. String [] siblings = {"Ernest", "Mary", "Therman"}; The above two methods are inefficient for large arrays. The third option is most widely used. Also, there are more advanced ways such as filling with data from a text file. Iterate through the elements in an array. This also allows you to access each index value. for(int i = 0; i < stuff.length; i++ { }
Accessing elements in Arrays Iterating through each element is a popular method. See examples 9-5, 9-6, and 9-7. (You should do remix) Let s try this: size(600,200); background(255); stroke(#cc0000,30); int n = 1000; //reason: to make easy to change float[] xTop = new float[n]; float[] xBottom=new float[n]; for(int i = 0; i<n; i++) { xTop[i] = random(50,550); xBottom[i] = random(50,550); line(xTop[i], 25, xBottom[i],175); //random horz but same vert }
Time for Fun Programming again #54 https://www.funprogramming.org A bit of a remix with names and descriptions of favorite music band or performer.
Create a String array with about 5 names of family members. Iterate through each. Do It If time permits, use a for() to place a rectangle around each name. Yourself println() or text() to see results http://moorec.people.cofc.edu/fam.txt (See solution at http://moorec.people.cofc.edu/fam.txt )
Create a Bar Chart Look at bar chart example at:https://processing.org/tutorials/arrays Then remix your own way. PImage tiger ; int [] clem = {37, 49, 41 ,42, 73, 47, 34, 40 }; void setup() { size(600,315); tiger = loadImage("clemson.jpg"); } void draw() { //Tinted and added image tint(150); image(tiger, 0, 0 ); //black rectangle at top fill(0); rect(0,0, width, 40); //for aesthetics, move remainder of items over 100px //then added text translate(100, 0); fill(#9900CC); textSize(22); text("Clemson's 2020 Scores", 0, 25); //Loop for the bars for (int j = 0; j < clem.length; j++) { fill(#E57417); //orange rect(j*50, 300-clem[j], 40, clem[j] ); //how to get the scores in there? fill(255); textSize(11); text(clem[j] , j*50+10, 290); //how to space this same as rect? println(j); } }
Array with Objects Example 9-9, lacks visual appeal, but perfect example of how to use object as array. CHALLENGE: o How much of that old Car class can you create without looking in 5 minutes? o I ll give you some particulars.
Interactive Objects Rollover effects: - Example 9-10; lots of possibilities. - Popular in software interfaces of all kinds. - Can be used for appearance or functionality. Let s copy and discuss Example 9-10. Remix by making stripe red when mouse hovers over it.
Example 9-10 partial class Let s do incremental dev on this class Stripe { float x; // horizontal location of stripe float speed; // speed of stripe float w; // width of stripe // A boolean variable keeps track of the object's state. boolean mouse; // state of stripe (mouse is over or not?) Stripe() { // All stripes start at 0 x = 0; // All stripes have a random positive speed speed = random(1); w = random(10,30); mouse = false; } // Draw stripe void display() { noStroke(); rect(x,0,w,height); } // Move stripe void move() { x += speed; if (x > width + 20) x = -20; } }//end class
Revisit a design I shared in chap 6 //inspired by adult coloring books int xloc= 0; int yloc= 0; int size= 160; int spacer = size/2; //Program will randomly pick from these colors. //swatches better than totally random because you can better target your choices. color [] c = {#0ff0ff, #00ff22, #ff6609, #ccff00, #660033}; void setup() { size(660,390); background(255); frameRate(10); } void draw() { //noLoop() ; stroke(#ff6633); for(int xloc=0 ; xloc<=width ; xloc += spacer) { for(int yloc = 25; yloc <= height; yloc += spacer) { fill(c[int(random(0,4) )], 30); //fill with array "c" ellipse(xloc, yloc, size, size); stroke(#993366); ellipse(xloc, yloc, size*.5, size*.9); //smaller ellipse is oval fill(c[4]); ellipse(xloc, yloc, size/10, size/10); } //end of nested for } } This is my remix.
Wormy Bugs Jitterbug[] bugs = new Jitterbug[1000]; class Jitterbug { void setup() { fullScreen(); background(0); for( int i = 0; i< bugs.length; i++) { bugs[i] = new Jitterbug(random(width), random(width), i/80); } float x; float y; int diameter; float speed = 1; Jitterbug(float tempX, float tempY, int tempDiameter) { x = tempX; y = tempY; diameter = tempDiameter; } } void draw() { fill(#CCD300); strokeWeight(.25); stroke(#AB0ACB); for(int i = 0; i<bugs.length; i++){ bugs[i].display(); bugs[i].move(); } } void move() { x += random(-speed, speed); y += random(-speed, speed); x = constrain(x, 0, width); y = constrain(y, 0, height); } void display() { ellipse(x, y, diameter, diameter) ; } }