Working with JavaScript Arrays: Storing and Accessing Data
Arrays in JavaScript serve as containers to hold multiple values like strings, numbers, and booleans within a single variable. They provide a more efficient way to store and manage data compared to using individual variables for each value. The guide covers creating arrays, initializing them, accessing elements using indexes, and displaying array contents. It also explains the significance of array indexes starting at 0 and methods to access the last element. By leveraging arrays effectively, developers can streamline data handling and manipulation in their JavaScript programs.
Uploaded on Jul 23, 2024 | 0 Views
Download Presentation
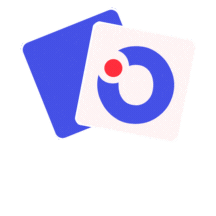
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Storing Data in Arrays JavaScript arrays are used to store multiple values (strings, numbers, booleans, etc.) in a single variable.
Empty Array var cars = [ ];
Arrays var cars = ["Saab", "Volvo", "BMW"];
3 Variables Longer Method var car1 = "Saab"; var car2 = "Volvo"; var car3 = "BMW";
Array [Index (position)] Begins at [0] Begins at [0] *NOTE [0] is the first index var cars = ["Saab", "Volvo", "BMW"]; 0 1 2 cars[0] Saab cars[1] Volvo cars[2] BMW cars
Array [Index (position)] Last [Index] Last [Index] **IMPORTANT arrayname[arrayname.length-1] is also another way to access the last index. var cars = ["Saab", "Volvo", "BMW"]; 0 1 2 cars[0] Saab cars[1] Volvo cars[2] BMW cars
<p id="demo"></p> OUTPUT: Entire array will be displayed. <script> var cars = ["Saab", "Volvo", "BMW"]; document.getElementById("demo").innerHTML = cars; </script>
Declaring and Initializing Arrays Syntax 1) Creates an Array, and 2) assigns values to it var array_name = [item1, item2, ...]; var cars = ["Saab", "Volvo", "BMW"]; **Preferred Method
Declaring and Initializing Arrays Syntax Spanning several lines Spanning several lines Creates an Array, and assigns values to it var cars = [ "Saab", "Volvo", "BMW" ];
ACCESSING ACCESSING Elements of an Array 0 1 2 Refer to their index number. cars[0] Saab cars[1] Volvo cars[2] BMW cars var name = cars[0]; What will the variable name hold in memory?
ACCESSING ACCESSING Elements of an Array 0 1 2 cars[0] Saab cars[1] Volvo cars[2] BMW cars <script> var cars = ["Saab", "Volvo", "BMW"]; document.getElementById("demo").innerHTML = cars[0]; </script> //OUTPUT: Saab
ACCESSING ACCESSING Elements of an Array at the END at the END 0 1 2 cars[0] Saab cars[1] Volvo cars[2] BMW cars <script> var cars = ["Saab", "Volvo", "BMW"]; document.getElementById("demo").innerHTML = cars[cars.length -1]; </script> //OUTPUT: BMW