Mastering For Loops in Python: A Comprehensive Guide
Explore the power and versatility of for loops in Python through real-world examples and best practices. Learn how to efficiently iterate over lists, perform calculations, and leverage built-in functions for effective loop control. Discover common pitfalls and tricks to enhance your loop-writing skills.
Download Presentation
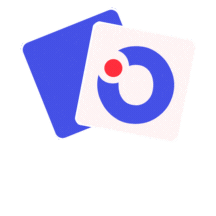
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
For Loops CMSC 201
Overview Today we will learn about: For loops
Motivation A lot of times, we have a pattern like this: myList = [1, 2, 3] a = 0 while a < len(myList): print(myList[a]) This is a way we can iterate over a list.
For Loops Equivalent for loop: myList = [1, 2, 3] for listItem in myList: print(listItem)
For Loops myList = [1, 2, 3] for listItem in myList: print(listItem) Loop control handled by for loop. The first time through the loop, listItem will be the first element of the list. The second time through the loop, it will be the second element, and so on until the list is done.
Example Finding the average using for loops: myList = [1, 2, 3, 4] sum = 0 for listItem in myList: sum = listItem + sum print( sum / len(myList) )
A Downside! What do you think this code does? myList = [1, 2, 3, 4] for listItem in myList: listItem = 4 print(myList)
A Downside! What do you think this code does? myList = [1, 2, 3, 4] for listItem in myList: listItem = 4 print(myList) Prints: [1, 2, 3, 4]
A Downside! What do you think this code does? myList = [1, 2, 3, 4] for listItem in myList: listItem = 4 print(myList) Changing listItem DOES NOT CHANGE THE ORIGINAL LIST! listItem is just a copy of each element.
For Loop Trick Frequently we want to do something like this: a = 0 while a < 10: print(a) a = a + 1 A lot of times, we want loops that count.
For Loop Trick Python built-in function for consecutive numbers! aList = range(0, 10) print(aList) Prints: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9] range(start, end) returns a list of numbers start, start+1, start+2, ..., end - 1
For Loop Trick aList = range(0, 10) print(aList) So now we can create a list of numbers. Any ideas what we can do with that?
For Loop Trick for num in range(0, 10): print(num) An easier way to make a counting loop! Prints: 0 1 2 3 4 5 6 7 8 9
For Loop Trick We can even count by whatever number we want! for num in range(0, 10, 2): print(num) Prints: 0 2 4 6 8
Summary: For loops: o Use for iterating over lists o Also use in situations where we want loops to count. While loops: o Use when we have some condition that doesn t fall into the previous two categories.