Explore Definite Loops in Java
Dive into the world of definite loops in Java, understanding their structure and behavior through practical examples. Learn about for loops, loop initialization, conditions, incrementing, and common pitfalls to avoid. Discover the concept of looping to execute code a specific number of times and enhance your understanding of iteration in Java programming.
Uploaded on Sep 24, 2024 | 0 Views
Download Presentation
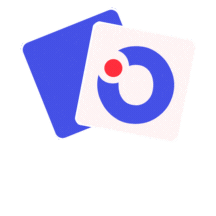
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Definite Loops A repetition structure that executes code exactly N times We know how many times we want it to loop int sum = 0; for (int i = 0; i < 10; i++) { sum += i; } System.out.println(sum);
For loop: int i = 10; for ( ; i > 0 ; i-=2) { System.out.println(i); } What does i start at? What happens to i each time the loop loops? When does this stop?
For loop for (int i = 0; ; i++) { System.out.println(i); } What does i start at? What happens to i after each loop? When does this stop (what is the stopping condition)?
Note If the loop-continuation-condition in a for loop is omitted, it is implicitly true. Thus the statements given below in (a) and (b), which are infinite loops, are correct. for ( ; ; ) { // Do something } while (true) { // Do something } Equivalent (a) (b) 4
For loop: for (int j = 30; j >=5; j -=5) { j = j - 1; System.out.println(j); } What does j start at? What gets printed out?
For loop: for (int p = 0; p < 10; p++) { System.out.print('*'); } What gets printed out? Why is this?????
For loop: int k = 0; for (int j = 30; j >=5; j -=5) { k+= j; } System.out.println(j); What gets printed out? Why is this?????
Try: Write a class with a method that computes the harmonic sum, which is calculated as follows: Notes: N is an integer, and will be the input to your method. What type is returned? Should you use a while or a for loop? Make one method run from 1 to 1/n Then make another method that runs from 1/n to 1. Are the results different? Wanna take a guess at why?
public class HarmonicSum { public static void main (String[] args) { System.out.println(HSLeftToRight(50000)); System.out.println(HSRightToLeft(50000)); } public static double HSLeftToRight(int maxDenom) { // for left-to-right double sumL2R = 0.0; for (int n = 1; n <= maxDenom; n++) { sumL2R += (double)(1.0/n); } return (sumL2R); } public static double HSRightToLeft(int maxDenom) { // for right-to-left double sumR2L = 0.0; for (int n = maxDenom; n >= 1; n--) { sumR2L += (double)(1.0/n); } return (sumR2L); } }
Simple guessing game Given an integer between 1 and 100 as an input parameter, to a method, how many many guesses must the computer make before it guesses the correct number? What kind of loop would you most likely use? Can you write this with all three loops?
import java.util.Random; public class Hello2 { public static void main (String[] args) { System.out.println("While " + whileGuess(72)); System.out.println("Rec " +recGuess(42)); System.out.println("for " +forGuess(27)); } public static int whileGuess(int g) { Random r = new Random(); int ct = 1; int x = r.nextInt(100); while (x != g) { x = r.nextInt(100); ct ++; } return(ct); } public static int recGuess(int g) { Random r = new Random(); int x = r.nextInt(100); if (x == g) { return(1); } else { return(1 + recGuess(g)); } } public static int forGuess(int g) { Random r = new Random(); int ct = 1; for (int x=r.nextInt(100);x != g; x = r.nextInt(100), ct++); return(ct); }