Mastering Nested For Loops: Tips and Tricks for Efficient Code Structure
Explore strategies for utilizing nested for loops effectively in your programming tasks. Learn how to control the number of lines printed, manage repeating patterns, and adjust output length using incrementing and decrementing outer loops. Enhance your coding skills with examples and best practices for nested for loops.
Download Presentation
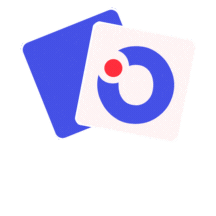
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
For Loop Tips And Tricks 1. The outermost loop typically controls the number of lines printed. 2. individual output line. Nested loops typically control repeating patterns within each Consider the following desired output: ***** ***** ***** and its corresponding nested for loop structure for (int i = 1; i <=3 ; i++) { 3 output lines for (int j = 1; j <=5; j++) { Repeated * System.out.print( * ); } System.out.println(); }
3. If the length of each subsequent output line repeating pattern is getting longer, try an outermost loop that INCREMENTS. If the length of each subsequent output line repeating pattern is getting shorter, try an outermost loop that DECREMENTS. Then use the value of the outermost loop counter to affect the inner loop repeating pattern. Consider the following 2 examples: Decreasing Output Length Increasing Output Length *** ** ** *** * **** **** *
Decreasing Output Length (Outer Loop Is Decrementing) (Outer Loop Is Incrementing) Increasing Output Length **** * *** ** ** *** * **** for (int i = 4; i >= 1; i--) { for (int i = 1; i <= 4; i++) { for (int j = 1; j <= i; j++) { for (int j = 1; j <= i; j++) { System.out.print( * ); System.out.print( * ); } } System.out.println(); System.out.println(); } }
4. There is typically one nested for loop inside the outer-most for loop for each repeating pattern in the lines of output. Consider the following two outputs: 1 ***1 *2 **22 **3 *333 ***4 4444 There is one repeating pattern (stars) in the first output. The number is not a repeating pattern because there is never more than one number on any given output line. There are 2 repeating patterns (stars and numbers) in the second output.
Considering the output with 1 repeating pattern (stars): 1 *2 **3 ***4 Based on the tips/tricks that we ve learned we can expect: 1. An outer-most INCREMENTING (because the output line size is getting larger) for loop that counts to 4 (number of output lines). 2. A single nested for loop for the repeating stars ( * ) that uses the value of the outermost loop counter to affect the inner repeating pattern because the number of stars is changing. 3. A print statement after the nested for loop to output the numeric.
Code: for (int i = 1; i <= 4; i++) { 4 output lines for (int j = 1; j < i; j++) { repeating * pattern System.out.print( * ); } System.out.println(i); non-repeating numeric } Output: 1 *2 **3 ***4
Now considering the output with 2 repeating patterns (stars & numbers): ***1 **22 *333 4444 Based on the tips/tricks that we ve learned we can expect: 1. An outer-most for loop that counts to 4 (number of output lines). You can either increment or decrement the outer-most loop control variable because the total number of character output per line (4) isn t changing. 2. 2 nested for loops, 1 for the repeating stars ( * ) and one for the repeating numerics.
Code: for (int i = 1; i <= 4; i++) { 4 output lines for (int j = 4; j > i; j--) { Repeating * pattern System.out.print( * ); } for (int k = 1; k <= i; k++) { Repeating numeric pattern System.out.print(i); } System.out.println(); } Output: ***1 **22 *333 4444
5. There are typically multiple nested for loops when there is a pattern of patterns in an individual output line. Consider the following desired output: ****!****!****! ****!****!****! We have a repeating pattern ( * ) which is probably a nested for loop, followed by an exclamation point ( ! ) that is probably printed after exiting the nested for loop. However, we also see a pattern of the pattern ****! . This is an indication that an additional level of nested for loops is present. There are 3 repetitions of this pattern ****! per output line, so we expect this additional nested for loop to increment from 1 to 3.
Code: for (int i = 1; i <= 2; i++) { 2 output lines for (int j = 1; j <=3; j++) { 3 repetitions of ****! for (int k = 1; k <= 4; k++) { Repeating * pattern System.out.print( * ); } System.out.print( ! ); Non-repeating ! } System.out.println(); } Output: ****!****!****! ****!****!****!