Introduction to Shell Programming in Linux/Unix with Vim and Control Flow Commands
Explore the basics of shell programming in Linux/Unix, including Vim commands for editing files, control flow commands like if-then statements, and examples of executing commands with built-in utilities. Learn how to handle arguments, read input, and implement conditional logic in scripts.
Download Presentation
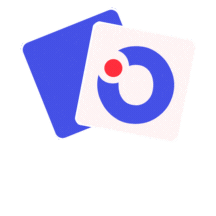
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Shell Programming (ch 10) IT244 - Introduction to Linux / Unix Instructor: Bo Sheng 1
Vim Commands vim filename Add contents: Back to command mode: Save the file: Delete: Quit: i/a ESC :w x :q 2
Control Flow The order of executing commands if..then 3
Control Flow The order of executing commands if..then Example ~it244/it244/scripts/if1 4
Control Flow The order of executing commands if..then Built-in utility test man test Example (-eq) ~it244/it244/scripts/chkargs $#: the number of arguments exit 1: terminate with a status code 1 5
Control Flow The order of executing commands if..then Read arguments Example ~it244/it244/scripts/read3args 6
Control Flow The order of executing commands if..then test (-f) and arguments Example ~it244/it244/scripts/is_ordfile Other options (Table 10-1) 7
Control Flow The order of executing commands if..then test ([ ]) and arguments Example ~it244/it244/scripts/chkargs2 White spaces [ $# -eq 0 ] Output error message: echo 1>&2 8
Control Flow The order of executing commands if..then..else 9
Control Flow The order of executing commands if..then..else Read arguments Example ( shift ) ~it244/it244/scripts/shift2args Example ( $@ ) ~it244/it244/scripts/allargs 10
Control Flow The order of executing commands if..then..else Optional arguments Example ~it244/it244/scripts/out 11
Control Structure if..then..elif 12
Control Structure if..then..elif Example: Compare 3 input words and find the match (word1, word2, word3) 1 & 2 & 3, 1 & 2, 1 & 3, 2 & 3, no match 13
Control Structure if..then..elif Example: Compare 3 input words and find the match (word1, word2, word3) if word1=word2 =word3 then elif word1=word2 then elif word1=word3 then elif word2=word3 then else fi 1 & 2 & 3 1 & 2 1 & 3 2 & 2 No match 14
Control Structure if..then..elif Example: Compare 3 input words and find the match (word1, word2, word3) Combine two conditions test condition1 -a condition2 test condition1 -o condition2 ~it244/it244/scripts/if3 15
Control Structure for loop for loop-index in argument-list do commands done Example ~it244/it244/scripts/fruit ~it244/it244/scripts/dirfiles 16
Control Structure for loop A range for argument-list {1..10}, {a..z} for loop-index in range do commands done Example ~it244/it244/scripts/for_range ~it244/it244/scripts/for_range2 17
Control Structure for loop Repeat operations Example ~it244/it244/scripts/for_print Nested loops Example ~it244/it244/scripts/for_print2 18
Control Structure for loop for loop-index (in $@) do commands done Example ~it244/it244/scripts/for_test 19
Control Structure while loop while test-command do commands done 20
Control Structure while loop while test-command do commands done Example: ~it244/it244/scripts/count Arithmetic evaluation: ((a=b+2)) ~it244/it244/scripts/for_while_print 21
Control Structure until loop until test-command do commands done 22
Control Structure until loop until test-command do commands done Example: ~it244/it244/scripts/guessnumber ~it244/it244/scripts/guessnumber2 23
Control Structure break and continue break: jump out of the loop continue: proceed to done Example: ~it244/it244/scripts/brk 24
Control Structure select select varname [in arg ] do commands done Example ~it244/it244/scripts/select1 25
Control Structure select select varname [in arg ] do commands done PS3: select prompt $REPLY: index of the selected item Terminate: exit, break Example: ~it244/it244/scripts/select2 26
Parameters and Variables Special parameters $#: number of arguments $1-$n: arguments $0: name of the script Example: ~it244/it244/scripts/abc ./abc ~it244/it244/scripts/abc basename: ~it244/it244/scripts/abc2 basename ~it244/it244/scripts/abc 27
Parameters and Variables Special parameters $$: PID number echo $$ ps Example: ~it244/it244/scripts/id2 ./id2 echo $$ 28
Parameters and Variables Special parameters $?: exit status ls; echo $? ls xyz; echo $? cat yyy; echo $? Example: ~it244/it244/scripts/es ./es echo $? echo $? 29
Parameters and Variables Initialize command-line arguments set arg1 arg2 ~it244/it244/scripts/set_it date ~it244/it244/scripts/dateset 30
Some More Examples Get file sizes ~/it244/it244/scripts/filesize $ls -l -rwxr-xr-x 1 it244 libuuid -rwxr-xr-x 1 it244 libuuid -rwxr-xr-x 1 it244 libuuid -rwxr-xr-x 1 it244 libuuid 50 2010-11-04 00:09 abc 62 2010-11-04 00:10 abc2 79 2010-11-04 00:49 bb1 79 2010-11-04 00:49 bb2 for i in * do set -- $(ls -l "$i") echo $i: $5 bytes done 31
File Descriptors An index number of a open file 3 system file descriptors 0(std input), 1(std output), 2(std err) Create a file descriptor exec n > outfile exec m < infile exec n<>file exec n>>outfile 32
File Descriptors Duplicate a file descriptor exec n<&m exec n>&m Close a file descriptor exec n<&- Example: ~it244/it244/scripts/exec_read ~it244/it244/scripts/exec_write 33
File Descriptors Example: ~it244/it244/scripts/exec_read exec 3<"$1" while read <&3 myline do echo "*** $myline ***" done File descriptor is 3 ($1 is the first argument) &3 represents the input file in the program (a pointer) read <&3 myline read a line from the file and assign it to variable myline (pointer &3 moves to the next line) 34
File Descriptors Example: ~it244/it244/scripts/exec_read exec 3<"$1" while read <&3 myline do echo "*** $myline ***" done Command as a condition True: if the exit status is 0 (successfully executed) False: if the exit status > 0 (sth went wrong) read gets an error when EOF is reached 35
Built-in Commands Do not fork a new process type cat echo who if read user input read firstline echo $firstline ~it244/it244/scripts/read1 ~it244/it244/scripts/read1_no_quote type in this is * 36
Built-in Commands read user input REPLY : default variable for read ~it244/it244/scripts/read1a -p option: with prompt read -p Enter a command: cmd ~it244/it244/scripts/read2 read multiple variables ~it244/it244/scripts/read3 type in this is sth else 37
Built-in Commands read user input read from files read line1 < names echo $line1 read line2 < names echo $line2 (read line1; echo $line1; read line2; echo $line2) < names 38
Built-in Commands read user input read from files ~it244/it244/scripts/readfile ./readfile < names -u option exec 3<names read u3 line1 (read <&3 line1) echo $line1 read u3 line2 echo $line2 exec 3<&- 39
Built-in Commands trap : catch a signal Signal: a report about a condition (Table 10-5) kill -l, trap -l, man 7 signal trap [ commands ] [signal] is not required but highly suggested Example: ~it244/it244/scripts/inter 40
Built-in Commands trap : catch a signal trap signal: ignore the signal Example 2: CTRL+C, 20: CTRL+Z ~it244/it244/scripts/locktty stty (input secret/passwd) ~it244/it244/scripts/locktty2 Multiple traps ~it244/it244/scripts/cleanfile 41
Built-in Commands kill : abort a process kill [-signal] PID The KILL signal (9) kill -9 %1 42
Expressions Arithmetic evaluation a=10; b=5; let a=a*10+b; echo $a No path expansion (*) ((a=a*10+b)) let a=a+1 b=a*b ((a=a+1, b=a*b)) 43
Expressions Logic expression (( )), [[ ]] In [[]], must precede a variable name with $ >, <, -lt, -gt, && (and), || (or) ~it244/it244/scripts/age2 String comparison [[ aa < bbb ]] [[ artist = a* ]]; echo $? [[ a* = artist ]]; echo $? 44
Expressions Arithmetic operators +,-,*,/,% a=5;b=3;echo $((a%b)) var++, var--, ++var, --var echo $((a++)); echo $a echo $((--a)); echo $a echo $((a+++3)) echo $((a++---b)) ~it244/it244/scripts/printstar 45
Expressions Logical operators true, false true; echo $?; false; echo $? !, ==, != ((1==1)); echo $?; echo $((1==1)) ((1!=1)); echo $?; echo $((1!=1)) &&, || true && false; echo $? true || false; echo $? ls l testop && cat testop; echo $? ls l testop || cat testop; echo $? 46
Expressions Logical operators &&, || Short-circuiting: Cond1 && Cond2: does not evaluate Cond2 if Cond1 is false Cond1 || Cond2: does not evaluate Cond2 if Cond1 is true Example mkdir bkup && cp testop bkup rm bkup/* mkdir bkup && cp testop bkup; ls bkup mkdir bkup || echo mkdir failed a=1;b=2;echo $((a||b++));echo $b 47