Rust References and Memory Safety in System Software Development
In Rust programming, references play a crucial role in ensuring memory safety and concurrency. Understanding concepts like owning pointers, shared vs. mutable access, working with references, and reference handling is vital for developing robust and secure system software in Rust. The language enforces strict rules on reference usage to prevent memory issues. References in Rust are non-owning pointers that must not outlive what they point to, ensuring safe memory management.
Download Presentation
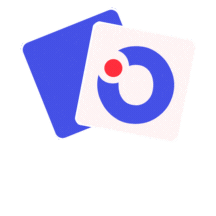
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
E81 CSE 542S: Concurrency and Memory Safe System Software Development References Department of Computer Science & Engineering Chris Gill cdgill@wustl.edu 1
References in Rust Owning pointers scope lifetime of what they point to, to theirs (unless it moves) E.g., Box<T> heap pointers E.g., internal pointers within strings and vectors, to their implementations References are non-owning pointers They must never outlive what they alias Borrow access from its owner, temporarily Rust checks and enforces all of that 2 CSE 542S Concurrency and Memory Safe System Software Development
Shared vs. Mutable Access Shared access is read-only access Once a shared reference (&T) has access, throughout the reference s lifetime what it aliases must be read-only from all accesses Mutable access is exclusive access Once a mutable reference (&mut T) has access, only it may be used to access what it aliases (unless it is mutable, and another mutable reference then borrows from it!) 3 CSE 542S Concurrency and Memory Safe System Software Development
Working with References I Passing shared references vs. moving E.g., declare as fn say(v: &VecType) rather than as fn say(v: VecType) E.g., call say(&v); vs. say(v); Passing mutable references is similar E.g., fn change(v: &mut VecType) E.g., call change(&mut v); Notice the strong typing on both the calling and receiving sides of the function signature 4 CSE 542S Concurrency and Memory Safe System Software Development
Working with References II Explicit dereference with * operator to assign E.g., let z = &mut x; *z = MAXVAL; // not C++ E.g., let mut s = hello".to_string(); {let r = &mut s; *r = world".to_string();} Implicit dereference with the . operator E.g., v.modify(); is equivalent to the longer syntax (&mut v).modify(); The . operator borrows a reference from v Can change where Rust references point E.g., let mut z = &x; z = &y; // not C++ 5 CSE 542S Concurrency and Memory Safe System Software Development
Working with References III References can refer to other references E.g., let b = &a; let c = &b; Comparison operators require same type but implicitly dereference to the value E.g., both b == *c and *b == **c are valid and equivalent comparisons (trivially true) References are never null or dangling Rust checks lifetimes (BOT pp. 109-115) 6 CSE 542S Concurrency and Memory Safe System Software Development
Working with References IV Rust also has two fat pointer types A reference to a slice has a length and a starting address as well as what it aliases Chapter 11 covers trait objects, each aliasing a value implementing a given trait References can be both passed into and returned from functions The tick mark ' lets you specify lifetimes explicitly, which Rust may require you to do 7 CSE 542S Concurrency and Memory Safe System Software Development
Structs Containing References A struct containing a reference may not outlive what the reference aliases and Rust won t tolerate ambiguity for that Lifetime parameters can help tell Rust how enclosing structs depend on references E.g., struct S<'a> {r: &'a i32} E.g., struct T<'a, 'b> {p: &'a i32, E.g., struct U<'a> {s: S<'a>} q: &'b i32} 8 CSE 542S Concurrency and Memory Safe System Software Development
Studio 4 Practice using shared and mutable references Explore how borrowing errors may be caused Examine when mutation is or is not allowed Understand dereference/assignment combined Try out using a block to scope lifetimes Studios 1 through 11 are due by 11:59pm on the night before Exam 1 Submit as soon as each is done so you get feedback and can resubmit any that may be marked incomplete 9 CSE 542S Concurrency and Memory Safe System Software Development