Introduction to Switch Statements in Programming
Switch statements in programming are control structures that allow for decision-making based on the number of choices available. This lecture covers the basics of switch statements, including syntax and examples. A practical programming problem involving triangle validation based on input angles is also discussed. The tutorial provides valuable insights into utilizing switch statements effectively in programming.
Download Presentation
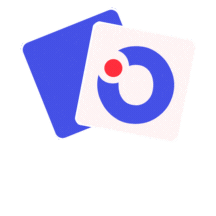
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Department of Computer Science & Engineering Air University Intro to Programming Week # 4 Switch Statement Lecture # 7 By: Saqib Rasheed
Quiz Time [20 Mins] Marks[10] Write a program to check whether a triangle is valid or not, when the three angles of the triangle are entered through the keyboard. A triangle is valid if the sum of all the three Angles is equal to 180 degrees CS 161 Intro To Programming
switch statement The control statement that allows us to make a decision from the number of choices is called a switch statement CS 161 Intro To Programming
switch statement switch ( variable name ) { case a : case b : case c : } statements; statements; statements; CS 161 Intro To Programming
switch statement switch ( grade) { case A : case B : case C : } cout << Excellent ; cout << Very Good ; CS 161 Intro To Programming
switch statement case A : cout << Excellent ; CS 161 Intro To Programming
Example switch ( grade) { } case A : case B : case C : case D : case F : cout << Excellent ; cout << Very Good ; cout << Good ; cout << Poor ; cout << Fail ; CS 161 Intro To Programming
break; CS 161 Intro To Programming
Example char grade; cin>>grade; switch ( grade ) { } case A : case B : case C : case D : case F : cout << Excellent ; break ; cout << Very Good ; break ; cout << Good ; break ; cout << Poor ; break ; cout << Fail ; break ; CS 161 Intro To Programming
default : default : cout << Please Enter Grade from A to D or F ; CS 161 Intro To Programming
Flow Chart of switch statement switch (grade) case A : Display Excellent case B : Display Very Good Default : .. CS 161 Intro To Programming
Example int grade; cin>>grade; switch ( grade ) { } case 1 : case 2 : case 3 : case 4 : case 5 : cout << Excellent ; break ; cout << Very Good ; break ; cout << Good ; break ; cout << Poor ; break ; cout << Fail ; break ; CS 161 Intro To Programming
Example # 1 int i = 2 ; switch ( i ) { case 1 : case 2 : case 3 : default : cout<<"I am in case 1 \n"; break; cout<<"I am in case 2 \n"; break; cout<<"I am in case 3 \n"; break; cout<<"I am in default \n"; } Output I am in case 2 CS 161 Intro To Programming
if-else Switch if (x == 1) { cout << "x is 1"; } else if (x == 2) { cout << "x is 2"; } else { cout << "value of x unknown"; } switch (x) { case 1: cout << "x is 1"; break; case 2: cout << "x is 2"; break; default: cout << "value of x unknown"; } CS 161 Intro To Programming
switch Versus if-else Ladder There are some things that you simply cannot do with a switch. These are: A float expression cannot be tested using a switch Cases can never have variable expressions (for example it is wrong to say case a +3 : Multiple cases cannot use same expressions. Thus the following switch is illegal: switch ( a ) { case 3.2 : case 4: case 1 + 2 : case 4: : CS 161 Intro To Programming
Example # 2 int day; cout<<"Eneter Day Number"; cin>>day; switch (day) { case 1 : cout << "\nSunday"; break; case 2 : cout << "\nMonday"; break; case 3 : cout << "\nTuesday"; break; case 4 : cout << "\nWednesday"; break; case 5 : cout << "\nThursday"; break; case 6 : cout << "\nFriday"; break; case 7 : cout << "\nSaturday"; break; default : cout << "\nNot an allowable day number"; break; } CS 161 Intro To Programming
switch (day) { case 1 : case 7 : cout << "This is a weekend day"; break; case 2 : case 3 : case 4 : case 5 : case 6 : cout << "This is a weekday"; break; default : cout << "Not a legal day"; break; } Break 1 Break 2 CS 161 Intro To Programming
Example # 3 char ch ; cout<< "Enter any of the alphabet a, b, or c "; cin>>ch; switch ( ch ) { case 'a' : case 'A' : cout<<"a is an apple" ; break ; case 'b' : case 'B' : cout<<"b as in brain"; break ; case 'c' : case 'C' : cout<<"c as in cookie"; break ; default : cout<<"wish you knew what are alphabets" ; } CS 161 Intro To Programming
Is switch a replacement for if? Yes and no. Yes, because it offers a better way of writing programs as compared to if, and no because in certain situations we are left with no choice but to use if. The disadvantage of switch is that one cannot have a case in a switch which looks like: case i <= 20 : CS 161 Intro To Programming
switch works faster than an equivalent if-else ladder. compiler generates a jump table for a switch during compilation during execution it simply refers the jump table to decide which case should be executed . if-elses are slower because they are evaluated at execution time CS 161 Intro To Programming
Question # 1 Make a calculator using switch statement It should take two numbers from user. Ask the user the function to perform Display the result CS 161 Intro To Programming
Question # 2 Que Write a menu driven program program in C++ using switch statement that contain option as under.( The Menu should ) Enetr 1--> To Find Largest Number Among Three Variables. Enetr 2--> To Find ODD or EVEN Enetr 3--> To Find Condition of Water Enetr 4--> To Find Grade Of Student CS 161 Intro To Programming
Question # 2 (Code) #include<iostream.h> Void main () { int option; cout<<"\n\t\tEnter the option\n"; cout<<"Enetr 1--> To Find Largest Number Among Three Variables.\n"; cout<<"Enetr 2--> To Find ODD or EVEN\n"; cout<<"Enetr 3--> To Find Condition of Water\n"; cout<<"Enetr 4--> To Find Grade Of Student\n"; cin>>option; CS 161 Intro To Programming
Question # 2 (Code) switch (option) { case 1: int a,b,c, larg; cout<<"Enter First Integer="; cin>>a; cout<<"Enter Second Integer="; cin>>b; cout<<"Enter Third Integer="; cin>>c; CS 161 Intro To Programming
Question # 2 (Code) if (a > b) else if (larg > c ) else break; larg = a; larg = b; cout<<"Largest is ="<<larg<<endl; cout<<"Largest is ="<<c<<endl; CS 161 Intro To Programming
Question # 2 (Code) case 2: int value; cout<<"Enter an Interger value "; cin>>value; break; if (value % 2 == 0) cout<<"Your number is EVEN\n"; else cout<<"Your number is ODD\n"; CS 161 Intro To Programming
case 3: int t; cout<<"Temperature Less than 0 = ICE \n" <<"Temperature Greater than 0 & " <<"Temperature Less than 100 = Water\n" <<"Temperature Greater than 100 = STEAM\n"; break; cout<<"\n\n\t\tPlease enter the Temperature="; cin>>t; if ( t <= 0 ) cout<<"Form of water is \"ICE\""<<endl; else if( t > 0 && t < 100 ) cout<<"Form is \"WATER\"\n"; else if ( t >= 100 ) cout<<"Form of water is \"steam\"\n ; CS 161 Intro To Programming
case 4: int grade; cout<<"Enter the grade of student="; cin>>grade; if ( grade >= 90 ) cout<< " Grade A \n"; else if (grade >= 80 ) cout<<" Grade B \n"; else if ( grade >=70 ) cout<<" Grade C \n"; else if (grade >=60) cout<<" Grade D \n"; CS 161 Intro To Programming
else { cout<<" Grade F \n"; cout<<" You have to take the classes again\n"; cout<<" Work Hard To Get Good Grade\n"; } break; default: cout<<"You Entered Invalid Option\n"; cout<<"Enetr A Valid Option\n"; break; } //End Of Switch } //End Of Main CS 161 Intro To Programming