Understanding Statement Atoms and Basic Syntax in Programming
The atomic components of a statement include delimiters, keywords, identifiers, literals, and operators. Basic syntax involves single-line statements, inline and block comments, and compound statements categorized by control flow markers. Different languages have distinct ways of structuring code, such as using braces or indentation. Python, for instance, relies on indentation for grouping statements. Consistent formatting is crucial to maintain code clarity and prevent errors in compound statements.
Download Presentation
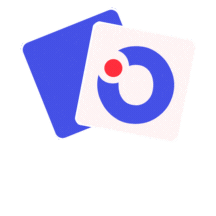
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Statement atoms The 'atomic' components of a statement are: delimiters (indents, semicolons, etc.); keywords (built into the language); identifiers (names of variables etc.); literals (values like 2 or "hello world") operators (maths symbols that do stuff).
Basic syntax Each statement is generally a single line (statements can be stacked on a line with semicolons but this is unusual). Standard 'inline' comments (text for humans) start # # This is an inline comment. 'Block' comments across multiple lines start """ and end """, or ''' and end ''' """ This is a block comment. """ You can have as many blank lines as you like to structure code.
Compound statements In many languages, lines have some kind of end of line marker (e.g. a semi- colon) and code is divided into control flow blocks by braces/curly brackets: if (a < 10) { alert (a); } alert("done"); // JavaScript But this allows errors (a missing bracket or semicolon can change the meaning), and requires coders to indent code inside the sections to make it clear where the control flow is.
Compound statements In Python, lines are generally one statement per line and are grouped in sections by indenting and dedenting. You can use spaces or tabs, and as many as you like of either, but be consistent. We recommend using 4 spaces. In theory, this means formatting and syntax are one. In practice, the interpreter basically looks at whether indenting is more or less than previous lines, this works in some versions of Python: if a < 10 : print (a) # Using a mix of tabs and spaces print ("done") But it isn't good practice. Align sections at the same flow control: if a < 10 : print (a) print ("done")
Compound statements Overall, this causes less bugs, but you need to make sure your indenting is correct or the control flow changes. This: if a < 10 : print (a) print ("done") Is completely different to this: if a < 10 : print (a) print ("done")
Delimiters Newlines and indenting/dedenting at the start of a line are special kinds of delimiter-ish things, as are ' " # \ Others (some of which also act as operators): ( ) [ ] { } , : . ; @ = -> += -= *= /= //= %= @= &= |= ^= >>= <<= **=
Enclosing delimiters Python especially uses three style of enclosing delimiters. These are what the Python documentation calls them: {} braces [] brackets () parentheses # Sometimes called curly brackets elsewhere. # Sometimes called square brackets elsewhere. # Sometimes called curved brackets elsewhere.
Line breaks Lines can be joined together into a single line with a backslash, \ provided this isn't part of a piece of non-comment text or followed by a comment. Lines inside {} () [] can also be split (and commented); though non-comment text needs quotemarks around each line. Triple quoted text may be split across lines, but the formatting (spaces; linebreaks) will be kept in the text when used (i.e. if it's not a comment). print \ (""" Daisy, Daisy, Give me your answer, do... """)
Keywords The following are reserved for special uses and can t be used for anything else: False class finally is return None continue for lambda try True def from nonlocal while and del global not with as elif if or yield assert else import pass break except in raise
Example: import Import adds libraries of code (modules in Python) so we can use them. Usually at the top of the file we add: import module_name For example: import builtins From then on we can use code inside the library. We'll come back to imports when we look at the standard library.
Identifiers These are labels stuck on things. The main use of identifiers is in constructing variables. But they are also used for naming, for example, procedures, or as Python calls them functions.
Builtins As well as keywords, there are some functions (like print()) built into the standard distribution. These are in a module called builtins. The shell loads this up automatically, but hides it with the name __builtins__ . This double-underscore notation is used in Python to hide things so they aren't accidentally altered. It is called 'dunder' notation It's easier to deal with if you import it normally. To see what's inside, enter: >>> import builtins >>> dir(builtins) dir() is one of the builtin functions. Used with a module name it tells you what functions and variables are available inside the module. Used on its own, it tells you what identifiers are currently being used.