Understanding Program Selection: If-Else Test Revisited with Switch Statements in C Programming
Discover the nuanced aspects of program selection in C programming through the exploration of if-else tests, switch statements, and logical operators. Dive into the implementation of a quadratic equation solver to understand how to calculate roots efficiently based on different conditions and user input validation.
Download Presentation
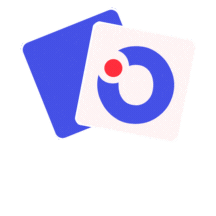
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Chapter 4 - Program Selection If-else test revisited switch statement standard form default break multiple cases
Standard if-else test if (expression) true_statement; else false_statement; next_statement;
logical operators (&&, ||, !) Partial evaluation when several logical operators appear in an expression, the evaluation begins on the left and continues only until the outcome of the whole expression is known. example: if (a==5 || c !=3) if a is equal to 5, the expression is true regardless of the value of c, thus the 2nd expression is skipped. careful arrangement can speed performance!
Program 4.1 - quadratic equation find the roots of a quadratic equation: ax2+ bx + c = 0 if a=0, x=-c/b else, disc=b*b-4*a*c, div = 2*a if disc>=0, x=(-b disc1/2)/div if disc<0, x=(-b i |disc|1/2)/div
Program 4.1 - specification start declare variables prompt for a, b, and c. read a, b, and c. if invalid data, print warning & stop if linear equation, print result calculate discriminant if discriminant >= 0 print real roots else, print complex roots stop
Start Program 4.1 Flowchart Declare Variables Prompt for and read a, b, c True True a=0 b=0 Print Error False False calculate discriminant Print single root False Print complex roots discr >= 0 True Print real roots Stop
Program 4.1 - part 1 /* calculate roots of quadratic equation */ #include <stdio.h> #include <stdlib.h> #include <math.h> int main(void) { char buff[BUFSIZ]; double a, b, c, div, descr, r1; printf("Enter Value for Coefficient 'a': "); gets(buff); a=atof(buff); printf("Enter Value for Coefficient 'b': "); gets(buff); b=atof(buff);
Program 4.1 - part 2 printf("Enter Value for Coefficient 'c': "); gets(buff); c=atof(buff); if (a==0) if (b==0) { printf("Invalid data...goodbye\n"); exit(0); } else printf("Only one solution for x= %g\n",-c/b); else { div=2.*a; descr=b*b-4.*a*c;
Program 4.1 - part 3 if (descr >= 0) { printf("roots are real\n"); printf("Root1 = %g\n",(-b+sqrt(descr))/div); printf("Root2 = %g\n",(-b-sqrt(descr))/div); } else { r1=sqrt(-descr)/div; printf("roots are complex\n"); printf("Root1 = (%g)+i(%g)\n",-b/div,r1); printf("Root2 = (%g)-i(%g)\n",-b/div,r1); } } return 0; }
else-if test (multi-way decision) if (expression1) statement1; else if (expression2) statement2; else if (expression3) statement3; else last_statement;
Switch statement - general form the general form is: switch (expression) case const_value: statement; switch and case are keywords the expression must be resolved to an integer the colon : indicates a label statement is executed if expression is equal to const_value (which must be a constant integer).
Switch statement - several cases Usually there are many cases: switch (expression) { case 1: statement1; case a : statementa; case 2: statement2; } Note: Execution begins at the first matched case and continues to the end of the statement! I.e. an a results in the execution of 2 statements.
Switch statement - break and default The break keyword can be used to allow only the execution of the expression immediately after the case , because it forces and immediate exit from the switch statement. The default keyword can be used to indicate an expression to be evaluated if none of the cases match the test expression. Multiple cases are achieved by cascading the labels.
Switch statement - example if (a==1) statement1; else if (a==2||a==3) statement23; else statement4; switch (a) { case 1: statement1; break; case 2: case 3: statement23; break; statement4; default: }
Program 4.2 - definition Write a code to read in the (real) value of a resistance and print out the resistor color code.
Program 4.2 - specification start declare variables prompt for resistance read resistance determine power of resistance determine first two digits of resistance look up and print digit colors look up and print color for power stop
Program 4.2 - part 1 /* print out resistor color code */ #include <stdio.h> #include <stdlib.h> int main(void) { char buff[BUFSIZ]; double value; int i,multiplier; void color_lookup(int); printf("Enter Resistor Value: "); gets(buff); value=atof(buff);
Program 4.2 - part 2 for(multiplier=-1;value>=10.;multiplier++) value=value/10.; for(i=0;i<2;i++) { if (buff[i]>='0'&&buff[i]<='9') color_lookup(buff[i]-'0'); else if (buff[i]=='.' && buff[i+1]>='0' && buff[i+1]<='9') color_lookup(buff[i+1]-'0'); else color_lookup(0); } color_lookup(multiplier); return 0; }
Program 4.2 - part 3 /* print color from table */ void color_lookup(int i) { switch(i) { case -1: printf("Gold "); break; printf("Black "); break; printf("Brown "); break; printf("Red "); break; printf("Orange "); break; case 0: case 1: case 2: case 3:
Program 4.2 - part 4 case 4: printf("Yellow "); break; printf("Green "); break; printf("Blue "); break; printf("Violet "); break; printf("Grey "); break; printf("White "); break; case 5: case 6: case 7: case 8: case 9: } return; }