Enhancing Python Programs with Comments and Programming Concepts
Understanding the importance of code readability through comments, Python programming concepts, conditional statements, and encryption/decryption. Explore tips for making code more readable, such as using booleans, if-elif-else statements, and while loops. Consider the significance of code appearance and simplicity in effective programming.
Download Presentation
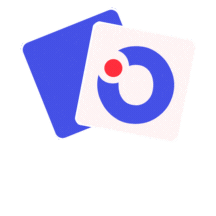
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Introduction to Python Module #4 Comments, Programming concepts, Conditional statements Lois Delcambre a little discussion a little more Python encryption and decryption programs
Plan making your programs more readable: comments coding style booleans if elif else while in Python additional Python instruction: Python console (aka Python interpreter) subscripts and decryption/encryption
Who is going to read code? When? How often?
Food for thought code is read much more often than it is written1 The visual appearance of source code is important. We want to require less human cognitive effort to understand a program.2 A program is a human-readable essay on problem solving that also happens to execute on a computer.3 1 Python Style guide from Guido van Rossum, the developer of Python 2 http://en.wikipedia.org/wiki/Programming_style 3 Punch and Enbody, The Practice of Computing using Python, p. 10, 2013, Pearson.
You can add comments to your program use the # symbol for simple comments You put comments in to your program so that the next programmer can read your code more easily. Python interpreter ignores all comments.
Comments are ignored by Python an example program with comments (guess_my_number3) Comments at the top indicate who wrote the program and when. Provides a brief summary. Comment here explains one line of code.
the way you type your program matters excerpt from Google Python Style Rules Line length Maximum line length is 80 characters. Parentheses Use parentheses sparingly. Indentation Indent your code blocks with 4 spaces. Blank Lines Two blank lines between top-level definitions, one blank line between method definitions. Imports formatting Imports should be on separate lines. Statements Generally only one statement per line.
Programming concept #1: Simplicity If something is less complicated, it s less likely to have problems and easier to troubleshoot and fix. easier to read! easier to maintain! easier to test! therefore it is more likely to work as intended and it is much more likely to be secure.
note: functions/methods are like limousines with tinted windows the outside program CAN T see any of the variables in the function/method and ... if you put functions/methods in a separate file (and then import them when you want them), even the programmer can t see the code (or variables)
lets try it out: variable inside a function The calling program can t see this variable called y. add_one_function 10
example showing that y (inside function) is not seen from outside program (try this) y is defined only inside the function code block The outer program tries to print y but Python tells us that y is not defined. add_one_function
Programming concept #2: Abstraction Each function/method introduces an abstraction This is what Chris Bosh explained to us. The function name/parameters gives you all that you need to know. all that you are allowed to know. The implementation details are hidden inside. You should introduce nice abstractions (functions) in your programs.
Programming concept #3: Modularity Modules can be inserted or removed from a project; each module code blocks can be changed to make it run faster, to make it more readable, and so on. Just make sure that the module still performs the same task. a function/method introduces modularity a group of functions/methods in a Python module is a module (e.g., the turtle module, the random module)
Programming concept #4: Information Hiding Information hiding is any attempt to prevent people from being able to see information. The code for functions and methods are not visible to the program that invokes them. The code inside a module (that is imported in Python) is not visible even to the programmer.
Plan making your programs more readable: comments coding style booleans if elif else while in Python additional Python instruction: Python console (aka Python interpreter) subscripts and decryption/encryption
New data type: Boolean (you already know integer and string data types) Exactly two constants; written exactly this way: True False Six comparators always return True or False: < less than > greater than <= (Note: you must put the < first) less than or equal to >= (Note: you must put the > first) greater than or equal to == (Note: you MUST use two equal signs) equal to != (can also be written as <>) not equal to 17
A program with a while loop This condition compares two variables from this program. If they are not equal (if the condition returns True), then the block is executed. guess_my_number1
Also count and print the number of guesses Initialize a new variable to 0 using an assignment statement. Replace num_of_guesses with the current value of num_of_guesses plus 1. guess_my_number2 This program will add 1 to num_of_guesses every time the code block in the while loop is executed. Here, we print the num_of_guesses
use a while True loop with break use an if/else in loop to make sure user input is valid (1) Using the in predicate here. will return True if the value of user_guess is equal to one of the values in this list. guess_my_number3
use a while True loop with break use an if/else in loop to make sure user input is valid (2) The constant True always evaluates to True. This loop will run forever. An if statement has a condition. If the condition evaluates to True, the code block is executed. The break statement exits the loop. else block is executed if user_guess was not in [1, 2, 3, 4, 5] guess_my_number3 The statement executed after break is this one.
use a while True loop with break use an if/else in loop to make sure user input is valid (3) one equal sign (=) in an assignment statement two equal signs (==) in a condition that is checking for equality. guess_my_number3
the 4 blocks guess_my_number3
Practice with while loops and if statements trinket.io Learn/Start Learning/If-Else Statements (3 lessons) Learn/Tutorials (down near the bottom of the page) click on the Learn button in the upper right corner scroll down to the tutorials choose the one on Conditionals (for if statements) choose the one on Loops (for while loops) you can also practice with the conditional expressions (used in if and while) with the tutorial on Logic Expressions
Plan for Day 4 making your programs more readable: comments coding style cybersecurity first principles booleans if elif else while in Python additional Python instruction: Python console (aka Python interpreter) subscripts and decryption/encryption
You can choose to run the Python console (also called the interpreter) Go to My Trinkets; choose New Trinket (Python) On this screen, Click on this button and then choose >_ Console.
The Python Console (interpreter) will be on the right side of the screen
If you use turtle graphics, the Console is on the lower right side of the screen turtle canvas here: python console here:
The console runs one Python statement at a time (immediately when you type it) As soon as I typed t1.forward and hit return, the turtle immediately went forward. Enter another command, and it will be executed immediately.
The console runs one Python statement at a time (immediately when you type it) Don t write entire programs this way. Use the left window in trinket to write programs and then save and run them. You know you re in the interpreter when you see >>> on the line.
Encrypting in Python Simplified versions of code that Dr. Wu-chang Feng used to generate the crypto puzzles.
Demo of transposition encoding (09_SUBSTITUTION_simple on trinket)
Python program for a substitution cypher What needs to happen? # s is the original message s = "the key for number nine is xxxxx" alphabet = "abcdefghijklmnopqrstuvwxyz " scramble = "zyxwvutsrqponmlkjihgfedcba "
Take the first letter in s s = "the key for number nine is xxxxx" alphabet = "abcdefghijklmnopqrstuvwxyz " scramble = "zyxwvutsrqponmlkjihgfedcba "
find it in the alphabet s = "the key for number nine is xxxxx" alphabet = "abcdefghijklmnopqrstuvwxyz " scramble = "zyxwvutsrqponmlkjihgfedcba "
replace it with the corresponding letter in scramble s = "the key for number nine is xxxxx" alphabet = "abcdefghijklmnopqrstuvwxyz " scramble = "zyxwvutsrqponmlkjihgfedcba "
put the letter from scramble into the new msg we are building s = "the key for number nine is xxxxx" alphabet = "abcdefghijklmnopqrstuvwxyz " scramble = "zyxwvutsrqponmlkjihgfedcba " encrypted_s = "g"
Take the second letter in s s = "the key for number nine is xxxxx" alphabet = "abcdefghijklmnopqrstuvwxyz " scramble = "zyxwvutsrqponmlkjihgfedcba " encrypted_s = "g"
find it in the alphabet s = "the key for number nine is xxxxx" alphabet = "abcdefghijklmnopqrstuvwxyz " scramble = "zyxwvutsrqponmlkjihgfedcba " encrypted_s = "g"
replace it with the corresponding letter in scramble s = "the key for number nine is xxxxx" alphabet = "abcdefghijklmnopqrstuvwxyz " scramble = "zyxwvutsrqponmlkjihgfedcba " encrypted_s = "g"
put the letter from scramble into the new msg we are building s = "the key for number nine is xxxxx" alphabet = "abcdefghijklmnopqrstuvwxyz " scramble = "zyxwvutsrqponmlkjihgfedcba " encrypted_s = "gs"
final result s = "the key for number nine is xxxxx" alphabet = "abcdefghijklmnopqrstuvwxyz " scramble = "zyxwvutsrqponmlkjihgfedcba " encrypted_s = "gsv pvb uli mfnyvi mrmv rh ccccc"
Several runs of the program Talk to your neighbor; does it look correct? alphabet = "abcdefghijklmnopqrstuvwxyz " scramble = "zyxwvutsrqponmlkjihgfedcba "
Setting things up 09_subsitution_simple
Find out how long alphabet is 09_subsitution_simple
Start out with an empty encrypted message 09_subsitution_simple
Process each letter in s using a for loop 09_subsitution_simple
Check each letter in alphabet 09_subsitution_simple
check to see if current character matches position i in alphabet (alphabet[i]) 09_subsitution_simple