Understanding Façade Design Pattern in Structural Design Patterns
Façade design pattern simplifies the interface of a complex system by providing a unified and straightforward interface for clients to access the system's functionalities. It helps in isolating the clients from the complexities of underlying components, offering a more user-friendly experience. The pattern is illustrated through examples from a compiler package, showcasing how Façade can streamline interactions with different components. This pattern is implemented through a layered structure of classes like Scanner, Parser, ProgramNodeBuilder, CodeGenerator, and Compiler. The compilation process is detailed step by step, emphasizing the role of Façade in organizing and presenting functionalities to clients.
- Structural Design Patterns
- Façade Design Pattern
- Simplified Access Interface
- Complex System
- Compiler Package
Download Presentation
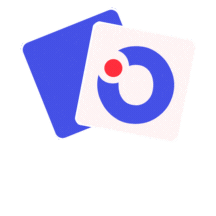
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Structural Design Patterns Yaodong Bi September 21, 2024
Structural design patterns Fa ade Decorator Composite Proxy Adapter Bridge Flyweight
Faade Design Purpose Provide a single and simple interface to a package of classes Design Pattern Summary Define a single and simple interface for clients to use the functionality of the package
Faade - examples A compiler package It normally contains many classes/subpackages like Scanner, Parser, etc. Most clients only want to compile their programs, i.e., they don t care about functions of individual components in the package Use Fa ade to provide a simple default interface to most clients.
Faade - Structure Client Use op1(), op2(), op3(), and op4() Facade op1() op2() op3() op4() ClassA op3_a() ClassC op4_c() ClassB op1_b() op2_b()
Sequence Diagram Client Facade B:ClassB A:ClassA C:ClassC op1() op1_b() op3() op3_a() op4() op4_c() op2() op2_b()
Faade - Structure Client Compiler.compile( test.c ) Compiler compile() ProgramNodeBuilder Scanner Parser CodeGenerator
Faade Sample code Class Scanner { public Scanner(InputStream sourcecode) public Token scan() { } } Class Parser { public parse(Scanner s, ProgramNodeBudiler p) { } } Class ProgramNodeBuilder { public ProgramNode newVariable( ) { } public ProgramNode newAssignment( ) { } public ProgramNode newReturnStmt( ) { } } Class CodeGenerator { public void visitStatementNode( ) { } public void visitExpressionNode( ) { } } Class Compiler { public void compile(InputStream sc, OutputStream bytecode) { Scanner sc = new Scanner(sc); ProgramNodeBuilder pnb = new ProgramNodeBuilder(); Parser parser = new Parser(); parser.parse(sc, pnb); IntelCodeGenerator cg = new IntelCodeGenerator(bytecode) ProgramNode nodetree = pnb.getRootNode(); parsetree.traverse(cg); }
Faade - Examples framework Customer getCustomerName() getNumAccounts() getPersonalNote() getAccount( int ) AccountException Account getAccountNum() deposit( int ) getBalance() CustomerException BankCustomers 1..n BankCustomer BankAccount Client BankCustomers doDeposit( int amt, Customer cust, Account acc ) getBankAccount( Customer cust, int accNum ) getBankCustomer( String custName )
Faade - comments Fa ade can reduce the degree of dependency between packages Packages are dependent on each other only through their facades, not individual classes Use Fa ade to provide a simple default view of the package that is enough for most clients Fa ade does not try to encapsulate/hide the components in the package since there may be clients who need to access individual components in the package
Decorator Design Purpose Add responsibilities to an object at runtime. Design Pattern Summary Provide for a linked list of objects, each encapsulating responsibility.
Decorator - examples The word processor example A text view may have a border and a scroll bar and maybe other bells and whistles attached to it How can those bells and whistles be added to the text view? Inheritance?
Decorator structure Component operation() Client ConcreteComp operation() Decorator Operation() comp void operation() { // do actions of the decorator comp.operation(); // do actions of the decorator }
Decorator structure VisualComponent draw() Client TextView draw() Decorator draw() comp comp.draw() ScrollDecorator draw() scrollTo() scrollPosition BorderDescrotor draw() drawBorder() borderWidth super.draw() this.drawBorder()
Decorator examples Client :Decorator1 :Decorator2 :ConcreteComp
Decorator - Sequence Diagram Client Component :Decorator1 :Decorator2 :ConcreteComp operation() operation() operation() operation() return return return return
Decorator examples :Reader 1 : BufferedStreamReader :InputStreamReader System.in:InputStream
Decorator examples : BufferedStreamReader :InputStreamReader System.in:InputStream
Decorator key concept allows addition to and removal from objects at runtime
Decorator sample in Java interface VisualComponent { public void draw(); } public class Decorator implements VisualComponent { private VisualComponent component; public Decorator(VisualComponent comp) { this.component = comp; } public void draw() {component.draw(); } } public class Border extends Decorator { int width = 0; public Border(VisualComponent comp, int width) { super(comp); this.width = width; } public void draw() { System.out.print("Border["+ width + "]"); super.draw(); System.out.print("[" + width + "]Border"); } } public class TextView implements VisualComponent { private String text = null; public void setText(String text) { this.text = text; } public void draw() { System.out.print("--" + text + "--"); } } public class Scroll extends Decorator { public Scroll(VisualComponent stream) { super(stream); } public void draw() { System.out.print("Scroll["); super.draw(); System.out.print("]Scroll"); } }
Decorator sample in Java public class Window { private VisualComponent component; public Window(VisualComponent comp) { this.component = comp; } public void setComponent(VisualComponent comp) { this.component = comp; } public void draw() { component.draw(); } } public class Driver { public static void main(String[] args) { TextView textView = new TextView(); textView.setText("Hello World!"); System.out.println("\n\TEXIVIEW ONLY "); Window window = new Window(textView); window.draw(); System.out.println("\n\nWITH SCROLL "); VisualComponent comp = new Scroll(textView); window.setComponent(comp); window.draw(); System.out.println("\n\nSCROLL + BORDER"); comp = new Border(new Scroll(textView), 3); window.setComponent(comp); window.draw(); } }
Decorator sample in C++ class VisualComponent { virtual void Draw(); virtual void Resize(); }; Class TextView: public VisualComponet { void draw(0 { // draw } void resize() { // resize }; } class Decorator : public VisualComponent { Decorator(VisualComponent*); void Decorator::Draw () { _component->Draw(); } VisualComponent* _component; }; class BorderDecorator : public Decorator { BorderDecorator(VisualComponent*, int borderWidth); void Draw(){ Decorator::Draw(); DrawBorder(_width); } private void DrawBorder(int); private int _width; }; Class Window { void SetContents(VisualComponent* contents) { // ... } Class Driver { public static void main() { Window* window = new Window(); TextView* textView = new TextView; window->SetContents(textView); Window.draw(); // textview only window->SetContents( new BorderDecorator( new ScrollDecorator(textView), 1 ) ); Window.draw(); // textview with a border // and scrolls. } }
Composite Design Purpose Represent a Tree of Objects Design Pattern Summary Use a Recursive Form in which the tree class aggregates and inherits from the base class for the objects.
Composite - Example My PPT Presentation Powerpoint Presentation 1. 2. 3. Item A Item B Item C item A Item B Item C slide Right panel Left panel Text title Photo grid image Bulleted list Numbered list 4 photos
Composite - structure Objects non-leaf node leaf node
Composite - structure Component add( Component ) Remove(component) doIt() 1..n Client comp Composite doIt() Add(component) Remove(component) LeafNodeA doIt() for all elements e in comp e.doIt() LeafNodeB doIt()
Composite A Class Diagram :Client N0:NonLeafNode N1:NonLeafNode N2:NonLeafNode L3:LeafNode L2:LeafNode L1:LeafNode
Composite sequence diagram :Client N0:NonLeafNode N1:NonLeafNode L1:LeafNode L2:LeafNode L3:LeafNode N2:NonLeafNode doIt() doIt() doIt() doIt() doIt() doIt()
Composite examples Component 1..n Composite in java.awt Container component . . Window Canvas
Proxy Design Purpose Avoid the unnecessary execution of expensive functionality in a manner transparent to clients. Design Pattern Summary Interpose a substitute class which accesses the expensive functionality only when required.
Proxy - example The proxy only has info of the extent of the image. You are seeing this part of doc now !!! The picture is not loaded into RAM until the user scrolls the windows to show it.
Proxy examples Instantiate with Proxy object BaseActiveClass expensiveMethod() anotherMethod() Client Proxy RealActiveClass expensiveMethod() anotherMethod() expensiveMethod() anotherMethod() if ( realActiveObject == null ) { // not loaded yet realActiveObject = getRealActiveObject(); } realActiveObject.expensiveMethod();
Proxy sequence diagram BaseActiveClass Proxy RealActiveClass Client expensiveMethod() create() if needed expensiveMethod()
Proxy examples Instantiate with Proxy object TexDoc graphics Graphics Display() Image display() bitmap ImageProxy display() fileName image if ( image == null ) { // not loaded yet image = new Image(fileName); } Image.display();
Proxy Sample code Class TextDoc { graphics g; TextDoc(Graphics ip) { g = ip; } void display() { g.display(); } } Class ImageProxy implements Graphics { FileName fileName; Image image; ImageProxy(FileName fn) { fileName = fn; } void display() { if (image == null) image = new Image(fileName); image.display(); } } Interface Graphics { display(); } Class Image Implements Graphics { Bitmap bitmap; Image(FileName fn) { bitmap = readImage(fn); } display() { // draw the bitmap } readImage(FileName fn) { // read from the file(fn) // create a bitmap } }
Adapter Design Purpose Allow an application to use external functionality in a retargetable manner. Design Pattern Summary Write the application against an abstract version of the external class; introduce a subclass that aggregate the external class.
Adapter - examples Interact with legacy systems When you design a new system which has to interact with a legacy system, you may not want to the new system tightly coupled with (or dependent upon) the legacy system since the legacy system may be replaced in the future. Using 3rd party systems You may want to be able to easily substitute the current 3rd party system with another one.
Object Adapter - Structure Target Client Adaptee +requestedMethod(); +request() adaptee Adapter request(TargetIn): TargetOut adapteeIn = convert(targetIn) adapteeOut = adaptee.requestedMethod(adapteeIn) targetOut = convert(adapteeOut) return tartgetOut;
Adapter sequence diagram Client Target Adapter Adaptee request(TargetIn):TargetOut request(TargetIn):TargetOut convert(TargetIn):AdapteeIn requestedMethod(AdapteeIn):AdapteeOut convert(AdapteeOut):TargetOut Return TargetOut Return TargetOut
Object Adapter sample code Interface Target { public TargetOut request(TargetIn); } Class Adapter implements Target { private Adaptee adaptee; public Adapter(Adaptee ad) { adaptee = ad; } public TargetOut request(TargetIn ti) { AdapteeIn ai = convert(ti); AdapteeOut ao = adaptee.requestedMethod(ai); return convert(ao); } private AdapteeIn convert(TargetIn ti) { // convert TargetIn to AdapteeIn } private TargetOut convert(AdapteeOut ao) { // convert AdapteeOut to TargetOut } } Class Adaptee { public AdapteeOut requestedMethod(AdapteeIn) { // produce AdapteeOut; return AdapteeOut; } }
Object Adapter - Example Shape DrawingTool TextView +getExtent(); +boundingBox() +createManipulator() text TextShape +boundingBox() +createManipulator() text.getExtent() return new TextManipulator()
Design for Adaption Pluggable Adapters A small set of operations is specified for adapter & adaptee to implement Using Abstract Operations Client and target are the same entity Adapter overrides abstract operations to delegate operations to adaptee Using Delegate Objects A delegate interface specifies operations the adapter needs to implement
Adapter - comments An adapter may have more than one adaptee There may not be a one-to-one correspondence between operations of Target and those of Adaptee So it is possible that an operation of Target is realized with two separate adaptee classes. The pattern decouples Client from adaptee. When a different adaptee is needed, we only need to change to another adapter and the client does not need to change at all.
Structural Patterns - Summary Facade provides an interface to collections of objects Decorator adds to objects at runtime Composite represents trees of objects Proxy avoids calling expensive operations unnecessarily Adapter decouples client from an existing system