Understanding the State Design Pattern in Software Development
The State Design Pattern is a Behavioral pattern similar to Strategy, allowing objects to change behavior based on internal state changes. This pattern involves defining different states and their implementations to control object behavior dynamically. Real-life examples like managing mood states and manufacturing devices showcase the versatility and benefits of using state patterns in software design. For a comprehensive understanding, consider exploring Steve Metsker's real-world applications of the State Design Pattern.
- State Design Pattern
- Behavioral pattern
- Software Development
- Strategy Pattern
- Object-Oriented Design
Download Presentation
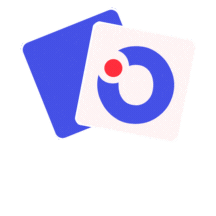
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
State Design Pattern C Sc 335 Rick Mercer 3-1
State Design Pattern State is one of the Behavioral patterns It is similar to Strategy Allows an object to alter its behavior when its internal state changes The object will appear to change its class
General Form from Wikipedia, copied from Gof4 Context class: Represents the interface to the outside world State abstract class: Base class which defines the different states of the state machine Derived classes from the State class: Defines the implementation of different states
Non Computer Example MyMood state variable MoodState doSomething() mad angry happy Client doSomething() doSomething() doSomething() doSomething()
Another Example from Steve Metsker's Design Patterns Java Workbook Consider the state of a carousel door in a factory Large smart rack that accepts material through a doorway and stores material according to a bar code
Behavior of this manufacturing device There is a single button to operate this door if closed, door begins opening if opening, another click begins closing once open, 2 seconds later (timeout), the door begins closing can be prevented by clicking after open state and before timeout begins Consider two ways to do this If statements State OO design pattern
Transition from one state to another This can get messy. Doing animations? read http://gameprogrammingpatterns.com/state.html public void click() { if (state == DoorStateEnum.CLOSED) { setState(DoorStateEnum.OPENING); } else if (state == DoorStateEnum.OPENING || state == DoorStateEnum.STAYOPEN) { setState(DoorStateEnum.CLOSING); } else if (state == DoorStateEnum.OPEN) { setState(DoorStateEnum.STAYOPEN); } else if (state == DoorStateEnum.CLOSING) { setState(DoorStateEnum.OPENING); } }
Alternative: The State Pattern Draw a State Diagram first A box representing one of the 5 states CLOSED OPEN When the carousel door can respond to a button click, or an observable s update message, draw an arrow to the next state and label the transition with click Or use the transitions complete or timeout
A UML State Diagram also a CSc 473 topic: finite state machine
State Machine characteristics Fixed set of states Closing, opening, closed, open Can only be in one state at a time Can t be opening and closing at the same time Events are sent to the machine Button clicks, observer notification Each state has a transition Machine changes to the state that transition points
Things to do implement state Define a context class to present a single interface Door Define a state abstract base class DoorState Represent different states by extending DoorState with DoorOpen, DoorClosed, DoorClosing, Define state-specific behavior in the appropriate subclasses public class DoorOpening extends DoorState { public void click() { door.setState(door.CLOSING); }
Things to do implement state Maintain current state in the context class private DoorState state = CLOSED; Change the state of the state machine in context and notify protected void setState(DoorState state) { this.state = state; setChanged(); notifyObservers(); }
Some of you might use State Complex if statements determine what to do if (thisSprite == running) doRunAnimation(); else if (thisSpite == shooting) doShootingAnimination(); else if (thisSpite == noLongerAlive) doRollOverAnimation(); ... Or An object can be in one of several states, with different behavior in each state state.doAnimation()