Understanding Pointers and Functions in C Programming
Explore the concepts of pointers, command line arguments, and functions in C programming. Learn how to manipulate values using pointers, handle character conversions, and return pointers from functions. Discover the limitations and best practices when working with pointers in C.
Download Presentation
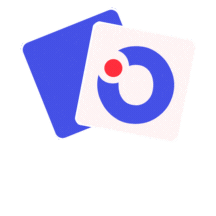
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
What did we talk about last time? Pointers Command line arguments
Imagine the following declarations int value = 10; int *pointer = &value; What are the types of the following? (one of them is illegal syntax) 1. &value 2. *pointer 3. &pointer 4. *value 5. pointer[0] 6. pointer + 4 7. value + 4 8. *(pointer + 4) 9. *&value
Let's write a function that takes a pointer to a char If the char is an upper case letter, we change it to lower case Otherwise, we do nothing Remember that most char values are not letters! Prototype: void makeLower(char* letter);
Functions can return pointers If you get a pointer back, you can update the value that it points to Pointers can also be used to give you a different view into an array char* moveForward(char* string) { return string + 1; } char* word = "pig feet"; while (*word) { printf ("%s\n", word); word = moveForward (word); }
Unfortunately, you can't return a pointer to a local variable Well, you can, but it would be crazy It would be pointing to a value that is no longer on the stack Maybe it's still there But the next time a function's called, it could be blown away
int* getPointer() { int value = 5; int* pointer = &value; return pointer; } main main p p getPointer value int* p = getPointer(); pointer After return
Just as we can declare a pointer that points at a particular data type, we can declare a pointer to a pointer Simply add another star int value = 5; int* pointer; int** amazingPointer; pointer = &value; amazingPointer = &pointer;
Well, a pointer to a pointer (**) lets you change the value of the pointer in a function Doing so can be useful for linked lists or other situations where you need to change a pointer Pointers to pointers are also used to keep track of dynamically allocated 2D arrays
Can you have a pointer to a pointer to a pointer to a pointer ? int*********** madness; Absolutely! The C standard mandates at least 12 modifiers are allowed for a declaration Most implementations of gcc allow for tens of thousands of stars There is no reason to do this, however
Three-Star Programmer A rating system for C-programmers. The more indirect your pointers are (i.e. the more "*" before your variables), the higher your reputation will be. No-star C-programmers are virtually non-existent, as virtually all non-trivial programs require use of pointers. Most are one-star programmers. In the old times (well, I'm young, so these look like old times to me at least), one would occasionally find a piece of code done by a three- star programmer and shiver with awe. Some people even claimed they'd seen three-star code with function pointers involved, on more than one level of indirection. Sounded as real as UFOs to me. Just to be clear: Being called a Three-Star Programmer is usually not a compliment. From C2.com
So far, we have only talked about using getchar() (and command line arguments) for input As some of you have discovered, there is a function that parallels printf() called scanf() scanf() can read strings, int values, double values, characters, and anything else you can specify with a % formatting string int number; scanf("%d", &number);
In the first place, you have to use pointers (or at least the reference operator &) I wanted you to understand character by character input (with getchar()) because sometimes that's the best way to solve problems Indeed, scanf() is built on character by character input Crazy things can happen if scanf() is used carelessly
These are mostly what you would expect, from your experience with printf() Specifier %d %u %o %x %hd %c %s %f %lf %Lf Type int unsigned int unsigned int (in octal for o or hex for x) short char null-terminated string float double long double
#include <stdio.h> int main () { char name[80]; int age; int number; printf("Enter your name: "); scanf("%s",name); printf("Enter your age: "); scanf("%d",&age); printf("%s, you are %d years old.\n", name, age); printf("Enter a hexadecimal number: "); scanf("%x",&number); printf("You have entered 0x%08X (%d)\n", number, number); return 0; }
scanf() returns the number of items successfully read Typically, scanf() is used to read in a single variable, making this value either 0 or 1 But it can also be used to read in multiple values int value1, value2, value3; int count = 0; do { printf("Enter three integers: "); count = scanf("%d %d %d",&value1, &value2, &value3); } while( count != 3 );
Write a program that asks a user how many strings they want to enter Read this number with scanf() Then, read in each string with scanf() Print out the string that comes earliest in the dictionary Hint: We don't need to store all the strings, only the current one and the earliest one we've found We can assume that the strings will be no longer than 100 characters (not including the null character)
Class will be replaced by a teaching talk from Dr. Hristov Extra credit opportunities (0.5% each): Hristov teaching demo: Hristov research talk: Time after that: Dynamic memory allocation 2/19 11:30-12:25 a.m. in Point 113 2/19 4:30-5:30 p.m. in Point 139
Keep reading K&R chapter 5 Work on Project 3