Programming Fundamentals Midterm Review
The review covers various topics including C parameters passing, compilation, program organization, C++ I/O, the use of stringstream, pointers, structures, classes, access control, constructors/destructor, and operator overloading. It includes code snippets and explanations on parameter passing, program organization rules, incremental compilation, pointers and structures, and classes with constructors and destructors.
Download Presentation
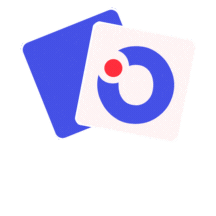
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
ECE 244 Programming Fundamentals: Midterm review
What we have learnt Review of C Parameters passing Compilation and program organization C++ I/O (the use of stringstream) Pointers + structures Classes Access control Constructors/destructor Operator overloading
Parameter passing: 2014 midterm Q1c 1 int do_something (int* & ptr1, int* ptr2) { 2 int* t; 3 *ptr1 = *ptr1 + *ptr2; 4 *ptr2 = *ptr1 + *ptr2; 5 t = ptr1; 6 ptr1 = ptr2; 7 ptr2 = ptr1; 8 return (*ptr1 + *ptr2); 9 } 10 int main () { 11 int* p = new int; 12 int* q = new int; 13 int z; 14 *p = 5; 15 *q = 8; 16 z = do_something (p, q); 17 z = z + *p + *q; 18 cout << z << endl; 19 return (0); 29 } p ptr1 q z Memory: 42 84 100 104 XX 104 addr. : 0 1 2 3 4 5 6 7 8 9 Memory: 13 5 21 8 addr. :100 104 t ptr2 100 104 104
Program organization The rule is very simple: you have to declare sth (e.g., a function or class) before you use it But you CANNOT declare a class/structure/global more than once! #ifndef SYMBOL #define SYMBOL Declarations go into header file Include all the necessary header files in .cpp file Only compile .cpp file link for incremental compilation
Incremental compilation g++ -c -Wall main.cc -o main.o g++ -c -Wall insert.cc -o insert.o g++ -c -Wall search.cc -o search.o g++ main.o insert.o search.o o linklist.exe
Pointers + structures int *p = new int; int **q = &p; **q = 100; cout << *p << endl; Pointers Pointers to pointers struct node { int data; struct node *next; } *head; Pointers to structures struct node *p = new node; struct node **q = &p; (*q)->data = 10; (*q)->next = new node; (*q)->next->data = 11; cout << p->next->data << endl; cout << ((*p).next)->data << endl; delete p->next; delete p;
Classes constructor/destructor 4 class A{ 5 public: 6 A () { cout << "A instantiated" << endl; } 7 ~A() { cout << "A destroyed" << endl; } 8 }; 9 A a; 10 A *foo() { 11 A b; 12 A *p = NULL; 13 cout <<"In foo << endl; 14 p = new A[2]; 15 return p; 16 } 17 int main() { 18 A c[3]; 19 A *q = NULL; 20 cout << "In main" << endl; 21 q = foo(); 22 delete [] q; 23 cout << "Done" << endl; 24 return 0; 25 } Output: A instantiated A instantiated A instantiated A instantiated In main A instantiated In foo A instantiated A instantiated A destroyed A destroyed A destroyed Done A destroyed A destroyed A destroyed A destroyed line 9 line 18 line 11 line 14 line 16 line 22 line 25, c[3] line 25, a
Array of objects line 18: A c[3]; An array of 3 objects, located on stack A *p = new A[2]; An array of 2 objects, located on heap A *p[10]; An array of 10 pointers No constructor is called! for (int i=0; i<10; i++) p[i] = new A;
Operator overloading Overloading binary operator: const Complex Complex::operator+ (const Complex &x) const { return Complex(real+x.getReal(), imag+x.getImag()); } A a = b; Complex a(3.2, 1.0); Complex b(4.0, 0.5); Complex c; c = a + b; Overloading unary operator: Complex& Complex::operator= (const Complex &x) { real = x.getReal(); imag = x.getImag(); return *this; }