Understanding Flask-SQLAlchemy: A Guide to Database Setup and Configuration
Dive into the world of Flask-SQLAlchemy and discover how to efficiently set up and configure databases for your software engineering projects. From installation requirements to creating models and connecting Python classes to database tables, this guide covers it all. Explore the motivation behind using SQLAlchemy as an Object Relational Mapping (ORM) tool and learn how Flask-SQLAlchemy simplifies the process by providing support for SQLAlchemy in Flask applications.
Download Presentation
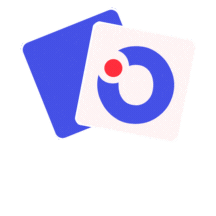
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Flask SQLAlchemy Setup CS331E Elements of Software Engineering II
Requirements Install Postgresql Install SQLAlchmey - pip install Flask-SQLAlchemy - pip install psycopg2
Outline SQLAlchemy Flask-SQLAlchmey Setup and configure the database
SQLAlchmey - Motivation Interacting with a database can be done using SQL syntax Using Flask, one can still use raw SQL Sending a query to the database will be as a strings But, python complier has no way of checking if there is a typo or reference a table that does not exist In Python (flask), every other data structure we use is some type of object. So, why not treat our database queries, tables, and rows as objects as well.
SQLAlchmey - Motivation Developer have created Object Relational Mapping (ORM) to handle such a situation. You can think of ORM as a translator, converting code from one form to another. One sends off code written in python The code is transformed by the ORM into SQL and sent off to the database The ORM also gets results from an SQL statement and allows us to use it as an object from within the Python code. SQLAlchemy is an open source ORM for Python with many features. https://www.sqlalchemy.org/library.html#tutorials
Flask-SQLAlchmey Flask extension to add support for SQLAlchmey to flask apps Facilitate connecting Python code to Database Provide a mapping between the Python classes with tables in the database
Creating a database with SQLAlchmey Configuration code: import all necessary modules - At the beginning of the file Import all modules needed Create a Flask object, have it pointing to the database, create an SQLAlchemy object and bind it to the Flask app - Creating models Class code: used to represent our data in Python Table: used to represent the specific table in the database Mapper: used to connect the columns of the table to the class that represents it - At the end of the file Create (or connect) the database and adds tables and columns
Creating a database with SQLAlchmey models.py Configuration code: import all necessary modules - At the beginning of the file Import all modules needed from flask import Flask from flask_sqlalchemy import SQLAlchemy import os Create a Flask object, have it pointing to the database, create an SQLAlchemy object and bind it to the Flask app
Creating a database with SQLAlchmey models.py Configuration code: import all necessary modules - At the beginning of the file Import all modules needed Create a Flask object, have it pointing to the database, create an SQLAlchemy object and bind it to the Flask app app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'postgresql://postgres:asd123@localhost:5432/bookdb' app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = True # to suppress a warning message db = SQLAlchemy(app)
Creating a database with SQLAlchmey models.py Configuration code: import all necessary modules - At the beginning of the file Import all modules needed Create a Flask object, have it pointing to the database, create an SQLAlchemy object and bind it to the Flask app - Creating models (will do it next) - At the end of the file Create (or connect) the database and adds tables and columns db.create_all()
Creating a database with SQLAlchmey models.py Configuration code: import all necessary modules Creating models - Class code: used to represent our data in Python - Table: used to represent the specific table in the database - Mapper: used to connect the columns of the table to the class that represents it class Book(db.Model): __tablename__ = 'book' title = db.Column(db.String(80), nullable = False) id = db.Column(db.Integer, primary_key = True)
Populating the DB - Create_db.py for oneBook in book['Books']: import json title = oneBook['title'] from models import app, db, Book id = oneBook['id'] def load_json(filename): newBook = Book(title = title, id = id) with open(filename) as file: jsn = json.load(file) # After I create the book, I can then add it to my session. file.close() db.session.add(newBook) return jsn # commit the session to my DB. db.session.commit() create_books()
Flask app - Main.py @app.route('/books/') from flask import render_template from create_db import app, db, Book, create_books def book(): db.session.query(Book).all() books = render_template('books.html', books = books) return @app.route('/') def index(): return render_template('hello.html') if __name__ == "__main__": app.run()
Hello.html <html> <head> </head> <body> <p>Hello World</b> </body> </html>
Books.html <html> <head> </head> <body> <p>This is the books page</b> <a href="{{url_for('index')}}">Main Menu</a> <br/> <h1>Books</h1> {% if books == [] %} <p>No books exist</p> {% else %} {% for i in books %} <div> {{i.title}} <br/> <br/> </div> {% endfor %} {% endif %} </body> </html>
Books.json { "Books": [ { "title": "Software Engineering", "id": "1" }, { "title": "Algorithm Design", "id": "2" }, { "title": "Python", "id": "3" } ] }