Introduction to Spring MVC with Template Engine
Spring MVC is a framework for building web applications in Java that follows a model-view-controller architecture. Controllers handle logic, process HTTP requests, and inject data into views using template engines like Thymeleaf. Views contain HTML pages with dynamic content injected from controllers using special tags. Models facilitate passing data to views, similar to Node.js. Controllers manage HTTP requests, identifying them with annotations like @Controller. Different controller types can return HTML views, JSON responses, or forward/redirect requests to other controllers, optimizing traffic and avoiding URL inflation.
Download Presentation
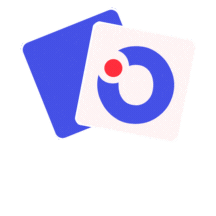
Please find below an Image/Link to download the presentation.
The content on the website is provided AS IS for your information and personal use only. It may not be sold, licensed, or shared on other websites without obtaining consent from the author. Download presentation by click this link. If you encounter any issues during the download, it is possible that the publisher has removed the file from their server.
E N D
Presentation Transcript
Spring MVC An intro to Spring MVC with template engine
A spring application Controllers Handle the logic Receive HTTP requests Manipulate data/objects and store them (session, cookies, database ) Inject data into a view Returns the view (response) Views Html pages Augmented html: special tags to insert dynamic content (the data injected from the controllers) Model The objects used to pass data to the view Q: similarity with Node.js?
Request / response thymeleaf
Controllers HTTP requests are handled by a controller (ex-Servlet) You can easily identify these requests by the @Controller annotation @Controller public class GreetingController { @GetMapping("/greeting") public String greeting(@RequestParam(name="somename", required=false, defaultValue="World") String name, model.addAttribute( username", somename); return "greeting"; } } - VIEW Model model) { - MODEL
Controllers @GetMapping : HTTP GET requests to /greeting @PostMapping for POST requests Equivalent to @RequestMapping(method = RequestMethod.POST) @GetMapping: shortcut for @RequestMapping(method = RequestMethod.GET) @RequestParam binds the value of the query String parameter name into the name parameter of the greeting() method. This query String parameter is not required; if it is absent in the request, the defaultValue of "World" is used.
Controller types Controller may return a view Response is HTML Controller may return JSON Response is intended for Javascript/Ajax client Controller may forward the request to another controller URL remains the URL of first controller, forward happens server side Controller may redirect to another controller Forward request sent to the browser, Twice as much traffic Solves the double submission problem Avoiding inflation of URLs: Some controllers do the logic, then redirect to controller that return views (and client populates the views with Ajax call) No data can be passed directly so it is based on states! (session, cookies, application scopes, database)
Returning HTML EJS ) ( - : 2 . view Model - .1 ( <input type= text name= somename > ) @Controller public class GreetingController { @GetMapping("/greeting") public String greeting(@RequestParam(name="somename", required=false, defaultValue="World") String aName, model.addAttribute( username", aName); return "greeting"; } } username greetings.html Model model) { view - ) ( - servlet String aName = request.getParameter( somename ); If (aName == null) aName = World ;
: templates Views view technology Thymeleaf (in Spring Java) EJS (in NodeJS) Thymeleaf performs server- side rendering of the HTML. Thymeleaf is a template engine: parses the greeting.html template below and evaluates the th:text expression to render the value of the username parameter that was set in the controller. <!DOCTYPE HTML> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>Getting Started: Serving Web Content</title> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> </head> <body> <p th:text="'Hello, ' + ${username} + '!'" /> </body> </html>
: Model the model can supply attributes used for rendering views To provide a view with usable data, we simply add this data to its Model object. Additionally, maps with attributes can be merged with Model instances: @GetMapping("/showViewPage") public String passParametersWithModel(Model model) { Map<String, String> map = new HashMap<>(); map.put("spring", "mvc"); model.addAttribute("message", hello"); // simple attribute model.mergeAttributes(map); // map attribute return "viewPage"; }
- : Map ModelMap pass a collection of values and treat these values as if they were within a Map @GetMapping("/printViewPage") public String passParametersWithModelMap(ModelMap map) { map.addAttribute("welcomeMessage", "welcome"); map.addAttribute("message", hello"); return "viewPage"; }
: model+view ModelAndView The final interface to pass values to a view @GetMapping("/goToViewPage") public ModelAndView passParametersWithModelAndView() { ModelAndView modelAndView = new ModelAndView("viewPage"); modelAndView.addObject("message", hello"); return modelAndView; }
Other params A controller method can accept various parameters such as: Session Request Model: hold data for the view BindingResult (later): holds the result of a validation and binding and contains errors that may have occurred. Any type with @Path, @RequestParam
? - Sessions - session @GetMapping("/someController") public String process(Model model, HttpSession session) { String message = (String) session.getAttribute("MY_MESSAGE"); // for example here we want to display the session variable MY_MESSAGE in the view if (message == null) { message =""; } model.addAttribute("sessionMessage", message); return someview"; }
Cookies - request or response @GetMapping("/change-username") public String setCookie(HttpServletResponse response) { // create a cookie Cookie cookie = new Cookie("username", Yosi"); //add cookie to response response.addCookie(cookie); } // more code end of controller annotation @GetMapping("/all-cookies") public String readAllCookies(HttpServletRequest request) { Cookie[] cookies = request.getCookies(); if (cookies != null) { // your code } // more code end of controller } @GetMapping("/get-one-cookie") public String getHeader(@CookieValue(name = username") String uname) { // .. . . }
/static : Static files HTML, CSS and JS are static files that need to be served as well from within the views (linked files) By default Spring Boot serves static content from resources in the classpath at "/static" (or "/public"). The index.html resource is special because it is used as a "welcome page" if it exists, which means it will be served up as the root resource, i.e. at http://localhost:8080/ (landing page)
Make the application executable package hello; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } }
The application annotations @SpringBootApplication is a convenience annotation that adds all of the following: @Configuration tags the class as a source of bean definitions for the application context. @EnableAutoConfiguration tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings. Normally you would add @EnableWebMvc for a Spring MVC app, but Spring Boot adds it automatically when it sees spring-webmvc on the classpath. This flags the application as a web application and activates key behaviors such as setting up a DispatcherServlet. @ComponentScan tells Spring to look for other components, configurations, and services in the hello package, allowing it to find the controllers. The main() method uses Spring Boot s SpringApplication.run() method to launch an application. No web.xml file! This web application is 100% pure Java and you dont t have to deal with configuring any plumbing or infrastructure.
? HTML Spring can handle views as well, that is generate dynamic pages The question is the following: should Java deal with server side only and leave the client side to other technologies? (Javascript, React) The answer is: probably yes What does it mean? Your application is probably a SPA (Single page application) or has very few HTML files All of you controllers return JSON data Your javascript code consists in making Ajax call and modifying the DOM
thymeleaf Thymeleaf is a Java template engine for processing and creating HTML, XML, JavaScript, CSS, and text. Must include in your HTML file: <html lang="en" xmlns:th="http://www.thymeleaf.org"> Automatically configured in SpringBoot projects under IntelliJ Can display property values Define a bean: @Bean @Description("Spring Message Resolver") public ResourceBundleMessageSource messageSource() { ResourceBundleMessageSource messageSource = new ResourceBundleMessageSource(); messageSource.setBasename("messages"); return messageSource; } Then use in view: <spanth:text="#{welcome.message}"/>
thymeleaf : Display model attributes Use the tag: th:text= ${attributename} model.addAttribute("serverTime", dateFormat.format(newDate())); Current time is <spanth:text="${serverTime}"/>
thymeleaf : Conditions th:if= ${condition} is used to display a section of the view if the condition is met. th:unless= ${condition} is used to display a section of the view if the condition is not met. public class Student implements Serializable { private Integer id; private String name; private Character gender; Model: // standard getters and setters <td> } <span th:if="${student.gender} == 'M'" th:text="Male" /> <span th:unless="${student.gender} == 'M'" th:text="Female" /> </td> View:
thymeleaf : Collections public class Student implements Serializable { private Integer id; private String name; // standard getters and setters } Assume the model is a collection: The controller adds: List<Student> students = new ArrayList<Student>(); // logic to build student data model.addAttribute("students", students); We can iterate in the view with: <tbody> <tr th:each="student: ${students}"> <td th:text="${student.id}" /> <td th:text="${student.name}" /> </tr> </tbody>
Avoid HTML duplication :Thymeleaf fragments A fragment is a piece of HTML for example <head><title>hello</title></head>) Include fragments of HTML in the resulting view 1. Define the controllers that return the fragments : 2. Create the HTML files (fragments) 3. Include fragments in views with th:insert or th:replace <head th:insert="fragments/head.html :: head"> </head> @Controller public class FragmentsController { @GetMapping("/header") public String getHeader() { return header.html"; } @GetMapping("/footer") public String getFooter() { return footer.html"; } } See: https://www.thymeleaf.org/doc/articles/layouts.html
More on thymeleaf Handling user input displaying Validation Errors Formatter (converting and displaying) Readings: https://www.thymeleaf.org/doc/articles/springmvcaccessdata.html https://www.baeldung.com/thymeleaf-in-spring-mvc
References https://spring.io/guides https://docs.spring.io/spring/docs/current/spring-framework- reference/index.html https://howtodoinjava.com/spring-5-tutorial/